Common Mistakes in Naming Variables and How to Fix Them
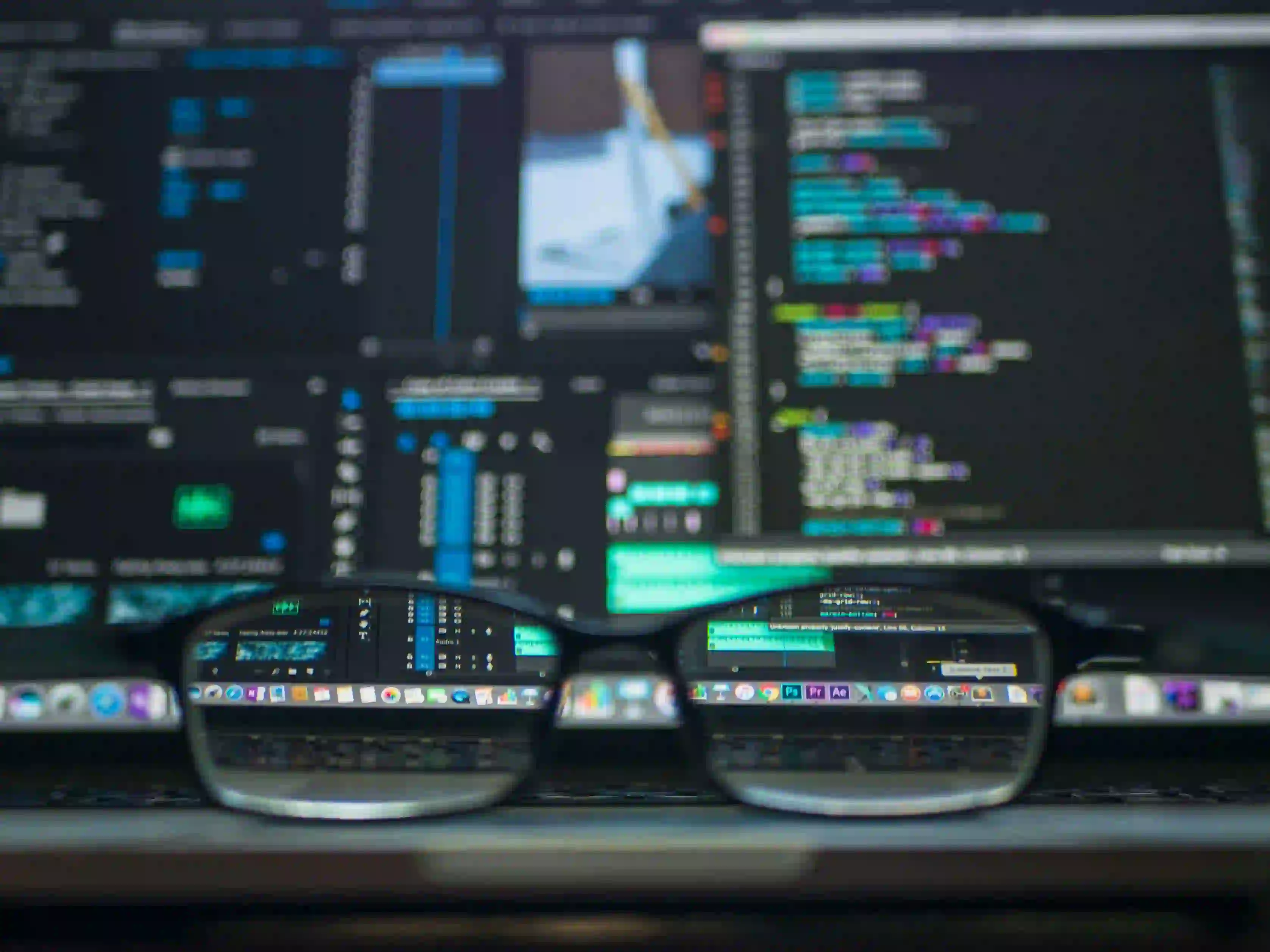
Common Mistakes in Naming Variables and How to Fix Them
When it comes to writing Java code, naming variables might seem like a trivial task, but it plays a crucial role in the readability and maintainability of your code. In this article, we'll discuss some common mistakes developers make when naming variables in Java and how to fix them.
1. Using Single Letter Names
One of the most common mistakes is using single letter names for variables. For example:
int x = 10;
This makes the code difficult to understand, especially in larger codebases or for someone else reading your code.
How to fix it: Be descriptive with your variable names. For example:
int age = 10;
2. Using Abbreviations
Abbreviations might seem like a good idea to save typing time, but they can make the code less readable.
int qtyAvl = 100;
This might be unclear to someone else reading the code.
How to fix it: Use descriptive names instead of abbreviations:
int quantityAvailable = 100;
3. Not Following Naming Conventions
Java has naming conventions for variables, which many developers often overlook.
How to fix it: Follow the standard conventions:
- Use camelCase for variable names.
- Start variable names with a lowercase letter.
- Use meaningful names that describe the variable's purpose.
int totalAmount = 500;
4. Inconsistent Naming Styles
Inconsistent naming styles can make the code look messy and unprofessional.
int total_amount = 500;
int totalAmount = 500;
This makes it harder to maintain and understand the code.
How to fix it: Pick a naming style and stick to it. If you’re working in a team, ensure everyone follows the same naming conventions.
5. Using Meaningless Names
Using names that don't convey the purpose of the variable is a common mistake.
int a = 10;
This leaves readers wondering what the variable represents.
How to fix it: Use descriptive names that convey the meaning:
int studentAge = 10;
6. Overly Verbose Names
While it's important to use meaningful names, excessively long variable names can make the code harder to read.
int numberOfStudentsPresentInClassroom = 30;
This can lead to excessive line wrapping and reduced readability.
How to fix it: Find a balance between clarity and brevity:
int numStudentsInClass = 30;
To Wrap Things Up
In conclusion, naming variables may seem like a small aspect of coding, but it significantly impacts the readability and maintainability of your code. By avoiding common mistakes and following best practices, you can ensure that your code is more understandable and easier to maintain.
Remember, the goal is to write code that not only works but is also easy for others to read and understand.
Feel free to share your thoughts and experiences with naming variables in Java!