Challenges with Scaling Test Automation in Continuous Testing
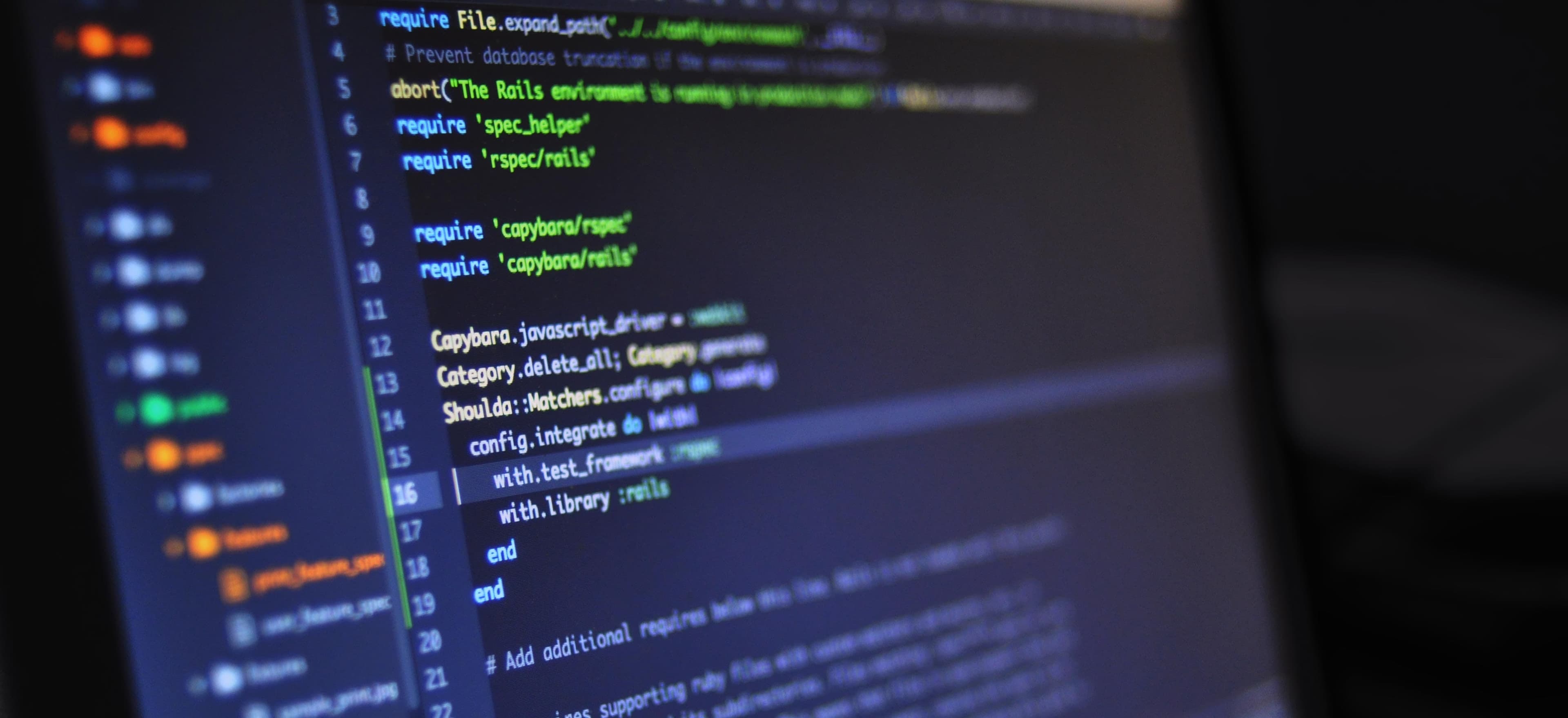
- Published on
Challenges with Scaling Test Automation in Continuous Testing
In today's fast-paced software development industry, continuous testing has become a crucial practice for ensuring the quality and reliability of software products. Test automation plays a pivotal role in continuous testing, enabling teams to execute tests quickly and efficiently. However, as the scale of software projects and test suites grows, teams often encounter challenges with scaling test automation in the context of continuous testing.
In this article, we'll delve into the common challenges faced when scaling test automation in continuous testing and explore strategies to address them effectively.
Understanding the Challenges
1. Test Environment Management
As the number of automated tests increases, managing the test environment becomes complex. Ensuring that the test environment is consistently configured and available for running tests across different platforms, browsers, and devices presents a significant challenge.
2. Test Data Management
Maintaining test data for a large number of automated tests becomes unwieldy. Generating and managing diverse sets of test data to cover various scenarios and edge cases is a time-consuming task that escalates with the scale of automation.
3. Execution Time
Executing a vast number of automated tests within a reasonable time frame is a substantial hurdle. Long test execution times can hamper the feedback loop, delaying the identification of issues and impeding the development velocity.
4. Maintenance Overhead
As the test suite expands, so does the maintenance overhead. Test scripts require constant updates to accommodate changes in the application under test, leading to increased maintenance efforts and potential brittleness of the automation suite.
5. Reporting and Analysis
With an abundance of automated tests, aggregating and analyzing test results to derive actionable insights becomes challenging. Generating comprehensive reports and identifying patterns in test failures necessitates robust reporting mechanisms.
Strategies for Addressing the Challenges
1. Infrastructure as Code (IaC)
Implementing Infrastructure as Code practices allows teams to automate the setup and configuration of test environments. Leveraging tools like Terraform and Ansible facilitates the provisioning of consistent test environments across various infrastructures, streamlining test environment management.
// Example using Terraform to define infrastructure
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
2. Data Provisioning Tools
Utilize data provisioning tools to automate the generation and management of test data. Tools such as Faker and Mockaroo enable the creation of diverse and realistic test data, reducing the manual effort involved in test data management.
// Using Faker library to generate mock data
Faker faker = new Faker();
String name = faker.name().fullName();
3. Parallel Execution
Employ parallel test execution to reduce overall execution time. Distributing tests across multiple agents or leveraging cloud-based parallel execution platforms, such as Selenium Grid or BrowserStack, can significantly diminish test execution duration.
// Parallel execution using TestNG
@Test
public void testMethod() {
// Test logic
}
4. Test Design Principles
Adopting robust test design principles, such as the Page Object Model (POM) or the Screenplay Pattern, promotes maintainable and scalable test automation. These design patterns enhance test modularity and reduce the impact of application changes on the test suite.
// Example of Page Object Model
public class LoginPage {
private final WebDriver driver;
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void enterUsername(String username) {
driver.findElement(By.id("username")).sendKeys(username);
}
// Other login page methods
}
5. Integrated Reporting Tools
Integrate reporting tools, like ExtentReports or Allure, to generate comprehensive test reports with interactive visuals. These tools offer insights into test execution status, trends, and historical data, facilitating informed decision-making based on test results.
// Integrate ExtentReports for test reporting
ExtentReports extent = new ExtentReports();
ExtentTest test = extent.createTest("MyFirstTest");
test.log(Status.INFO, "Test Step 1");
test.pass("Details");
extent.flush();
Scaling Responsively
As teams navigate the challenges of scaling test automation in continuous testing, it's imperative to prioritize responsive scaling. Proactive monitoring, strategic refactoring, and periodic reassessment of automation strategies are essential to adapt to the evolving needs of the project and prevent scalability issues from impeding development momentum.
By addressing these challenges proactively and implementing effective strategies, teams can not only scale their test automation efforts but also enhance the efficiency and reliability of their continuous testing practices.
In conclusion, scaling test automation in the context of continuous testing presents its share of challenges, but with the right strategies and tools, teams can surmount these obstacles and ensure that their test automation efforts keep pace with the demands of modern software development.
For further understanding of continuous testing and its challenges, explore articles on The Shift-Left Approach in Continuous Testing and The Importance of Test Environment Management.
Remember, scaling test automation is not just about adding more tests; it's about building a robust and sustainable automation ecosystem that aligns with the dynamics of continuous testing.