Troubleshooting JUnit Theories
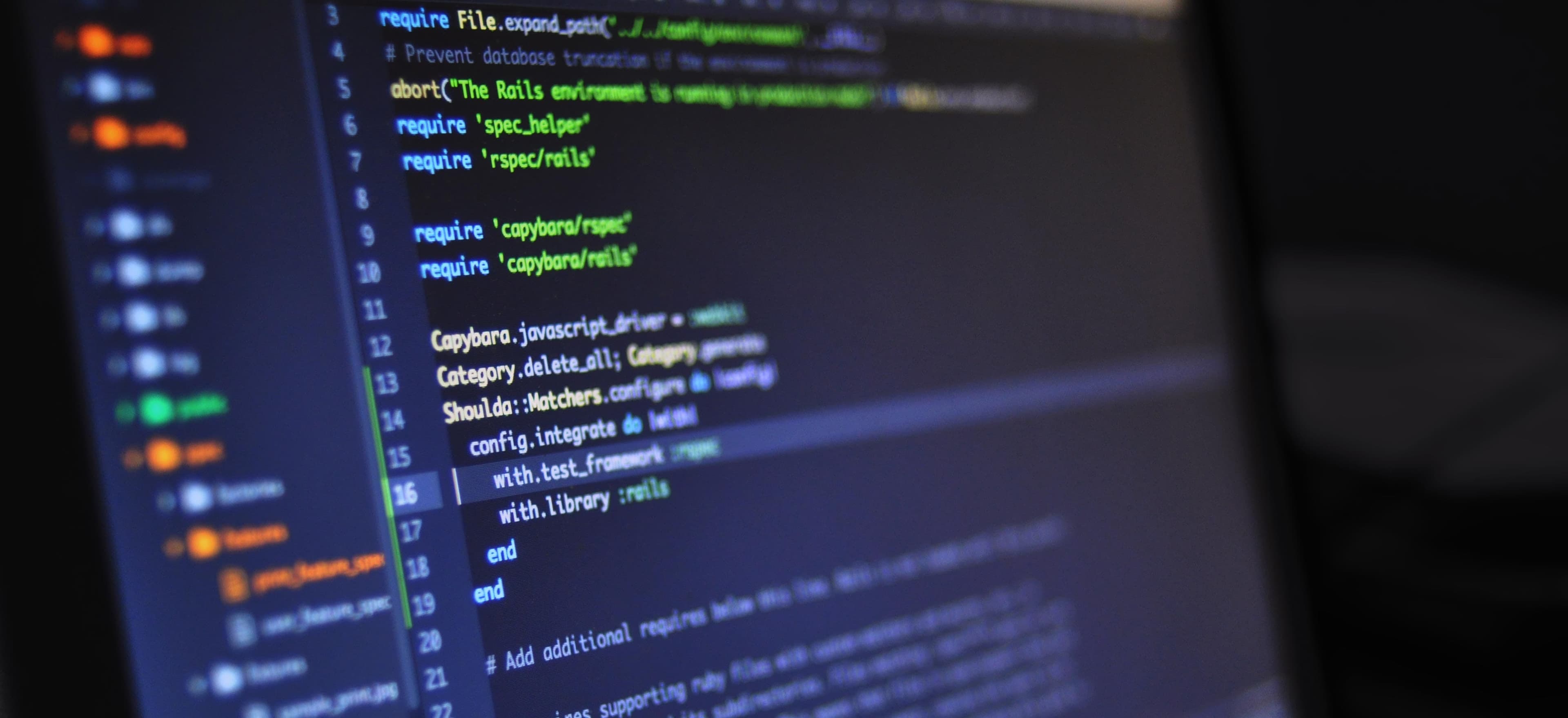
- Published on
Troubleshooting JUnit Theories: A Guide for Java Developers
JUnit is a popular testing framework for Java, known for its ease of use and effectiveness in ensuring the reliability of Java applications. One of the key features of JUnit is its support for parameterized testing, which allows developers to run the same test with different inputs. However, when working with parameterized tests, developers may encounter issues, particularly when using JUnit Theories.
In this post, we will explore common issues that arise when working with JUnit Theories and discuss troubleshooting strategies to resolve them.
Understanding JUnit Theories
Before delving into troubleshooting, let's first understand what JUnit Theories are and how they differ from regular parameterized tests. JUnit Theories, introduced in JUnit 4, are designed to verify a hypothesis about the code under test. Unlike parameterized tests, which focus on testing a set of input values, JUnit Theories allow developers to define general properties of the code and let the framework generate input values to validate those properties.
Common Issues and Troubleshooting Strategies
1. Unintended Test Invocation
One common issue with JUnit Theories is unintended test invocation, where a test method is executed with unexpected input values. This can occur due to incorrect test configurations or improper handling of input values by the theory method.
To troubleshoot unintended test invocation, ensure that the theory method specifies the correct input constraints using the @From
annotation from the org.junit.contrib.theories
package. Additionally, review the test method to verify that it correctly handles the generated input values.
import org.junit.contrib.theories.Theories;
import org.junit.contrib.theories.Theory;
import org.junit.contrib.theories.From;
public class TheoryTest {
@Theory
public void testProperty(@From(MyDataProvider.class) Object input) {
// Ensure proper handling of input
}
}
In the above example, MyDataProvider
should be a custom data provider class or an existing provider from the org.junit.contrib.theories
package.
2. Inconsistent Test Results
Another issue that developers may encounter when working with JUnit Theories is inconsistent test results, where the same theory yields different outcomes across test runs. This inconsistency can stem from non-deterministic behavior in the test method or the code under test.
To address inconsistent test results, carefully review the test method and the code being tested to identify any non-deterministic operations. It is important to ensure that the theory method and the test method are both deterministic to produce reliable and reproducible test results.
3. Unhandled Exceptions
When utilizing JUnit Theories, developers may face the challenge of unhandled exceptions within theory methods, leading to test failures and obscure error messages. This issue often arises from incomplete error handling within the theory method, resulting in unexpected exceptions during test execution.
To mitigate unhandled exceptions, implement robust error handling within the theory method to catch and appropriately handle any potential exceptions. Additionally, consider incorporating parameterized tests alongside JUnit Theories to validate exceptional scenarios with specific input values.
Closing Remarks
In conclusion, JUnit Theories provide a powerful mechanism for testing general properties of code with varying input values. While they offer numerous benefits, troubleshooting issues with JUnit Theories is essential for maintaining the reliability and effectiveness of tests.
By understanding the common issues and employing the suggested troubleshooting strategies, developers can address challenges with JUnit Theories to ensure the integrity of their test suites and ultimately improve the quality of their Java applications.
For further reading on JUnit Theories, refer to the official JUnit documentation and JUnit parameterized tests.
Happy testing!