Managing Context Propagation in Bulkhead CDs
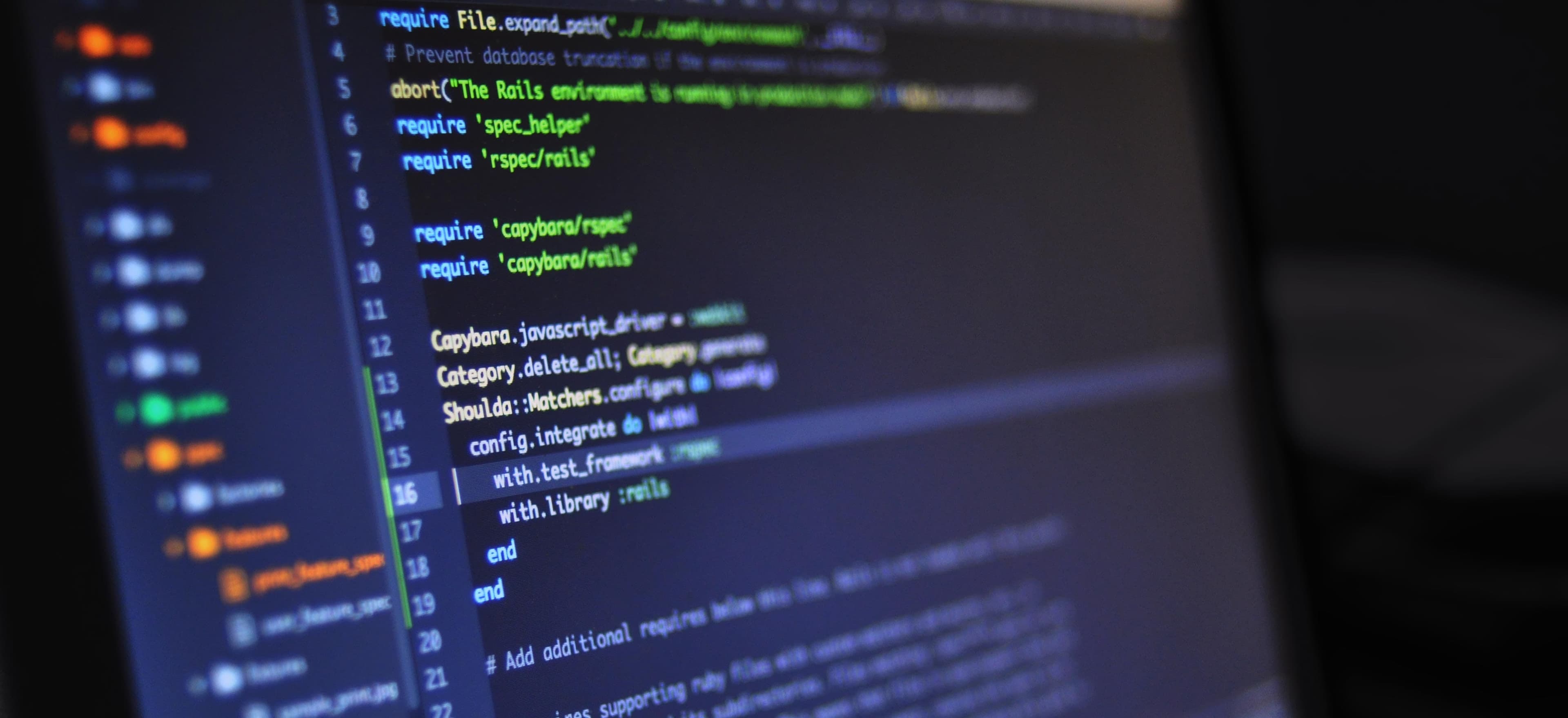
- Published on
Managing Context Propagation in Bulkhead CDs
In a microservices architecture, managing concurrent requests and ensuring the overall stability of the system is crucial. This is where the Bulkhead pattern comes into play. Bulkhead Control provides an effective way to isolate failures in a part of the system, preventing them from cascading to other parts. However, managing context propagation in Bulkhead Control can be challenging, especially when dealing with asynchronous and parallel processing.
In this article, we will explore how to manage context propagation in Bulkhead Control using Java and provide a solution to efficiently handle the propagation of contextual information.
Understanding Bulkhead Control
Before we delve into context propagation, it's essential to understand the concept of Bulkhead Control. The Bulkhead pattern is inspired by the physical concept of bulkheads on ships, which are watertight compartments that prevent flooding in case of a hull breach. Similarly, in software, the Bulkhead pattern isolates parts of the system to prevent failures from spreading.
In a microservices architecture, the Bulkhead pattern is commonly applied to limit the impact of failures in one microservice from affecting others, thereby improving overall system resilience. This is achieved by limiting the number of concurrent requests or connections that a component can handle at a given time.
Challenges with Context Propagation in Bulkhead Control
When implementing Bulkhead Control, one of the key challenges is managing context propagation. Context propagation involves carrying contextual information such as request metadata, authentication tokens, and correlation IDs throughout the execution flow. This becomes particularly complex in scenarios involving asynchronous processing, parallel execution, or distributed tracing.
Without proper context propagation, it becomes difficult to trace and correlate logs, monitor performance, and troubleshoot issues effectively. In the context of Bulkhead Control, ensuring that contextual information is propagated correctly across isolated components is crucial for maintaining visibility and observability within the system.
Solution: Using Thread-Local Context and ExecutorService
To address the challenge of context propagation in Bulkhead Control, we can leverage the use of Thread-Local variables and custom ExecutorService. By encapsulating contextual information within Thread-Local variables and managing the execution of tasks using a custom ExecutorService, we can ensure that context is propagated consistently across concurrent requests.
Let's take a look at a simplified example of how we can achieve this in Java:
Example: Managing Context Propagation
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ContextPropagatingExecutorService {
private static final ThreadLocal<String> context = new ThreadLocal<>();
private static ExecutorService executorService = Executors.newFixedThreadPool(10, runnable -> {
String originalContext = context.get();
return () -> {
try {
context.set(originalContext);
runnable.run();
} finally {
context.remove();
}
};
});
public static void executeWithContext(Runnable task, String contextValue) {
context.set(contextValue);
executorService.submit(task);
}
}
In this example, we create a ContextPropagatingExecutorService
that encapsulates an ExecutorService
with a custom thread factory. The ThreadLocal
variable context
is used to store the contextual information. When a new task is submitted for execution, the current context is captured and propagated to the executing thread via the custom thread factory.
Commentary: Why This Works
By using a Thread-Local variable to store contextual information and wrapping the execution logic within a custom thread factory, we ensure that the context is carried over to the concurrent tasks. This approach allows us to maintain contextual integrity within the isolated execution threads, effectively managing context propagation in the context of Bulkhead Control.
Applying the Solution in Bulkhead Control
Now that we have a solution for managing context propagation using Thread-Local variables and a custom ExecutorService, let's see how we can apply this in the context of Bulkhead Control.
Example: Applying Context Propagation in Bulkhead Control
public class BulkheadService {
private static final ContextPropagatingExecutorService executorService = new ContextPropagatingExecutorService();
public void processRequest(String requestId) {
// Retrieve contextual information, e.g., authentication token, from the incoming request
executorService.executeWithContext(() -> {
// Propagate the contextual information to the isolated execution context
// Perform the actual processing logic within the isolated context
}, requestId);
}
}
In this example, the BulkheadService
encapsulates the execution of a request within an isolated context using the ContextPropagatingExecutorService
. When a request is received, the contextual information, such as the request ID, is passed to the executeWithContext
method, ensuring that the context is propagated to the concurrent task execution.
With this approach, we effectively manage context propagation within the context of Bulkhead Control, ensuring that contextual information is consistently carried over to isolated execution contexts.
Closing the Chapter
Managing context propagation in Bulkhead Control is crucial for maintaining visibility and observability within a microservices architecture. By leveraging Thread-Local variables and a custom ExecutorService, we can effectively manage context propagation, ensuring that contextual information is consistently propagated across isolated execution contexts.
In this article, we explored the challenges of context propagation in Bulkhead Control, provided a solution using Java, and demonstrated how to apply this solution in the context of Bulkhead Control. By incorporating effective context propagation mechanisms, we can enhance the resilience and observability of microservices, contributing to a more robust and maintainable system architecture.
For further reading about context propagation and Bulkhead Control, you can explore the documentation on ThreadLocal and ExecutorService.
Remember, effective context propagation is just one piece of the puzzle in building robust and resilient microservices architectures, and it's essential to continuously explore best practices and patterns to enhance the overall system reliability.
By implementing effective context propagation mechanisms in Bulkhead Control, we pave the way for improved system visibility and resilience in microservices architecture.
Happy coding!
Checkout our other articles