Optimizing Slick Database Access on JVM Platform
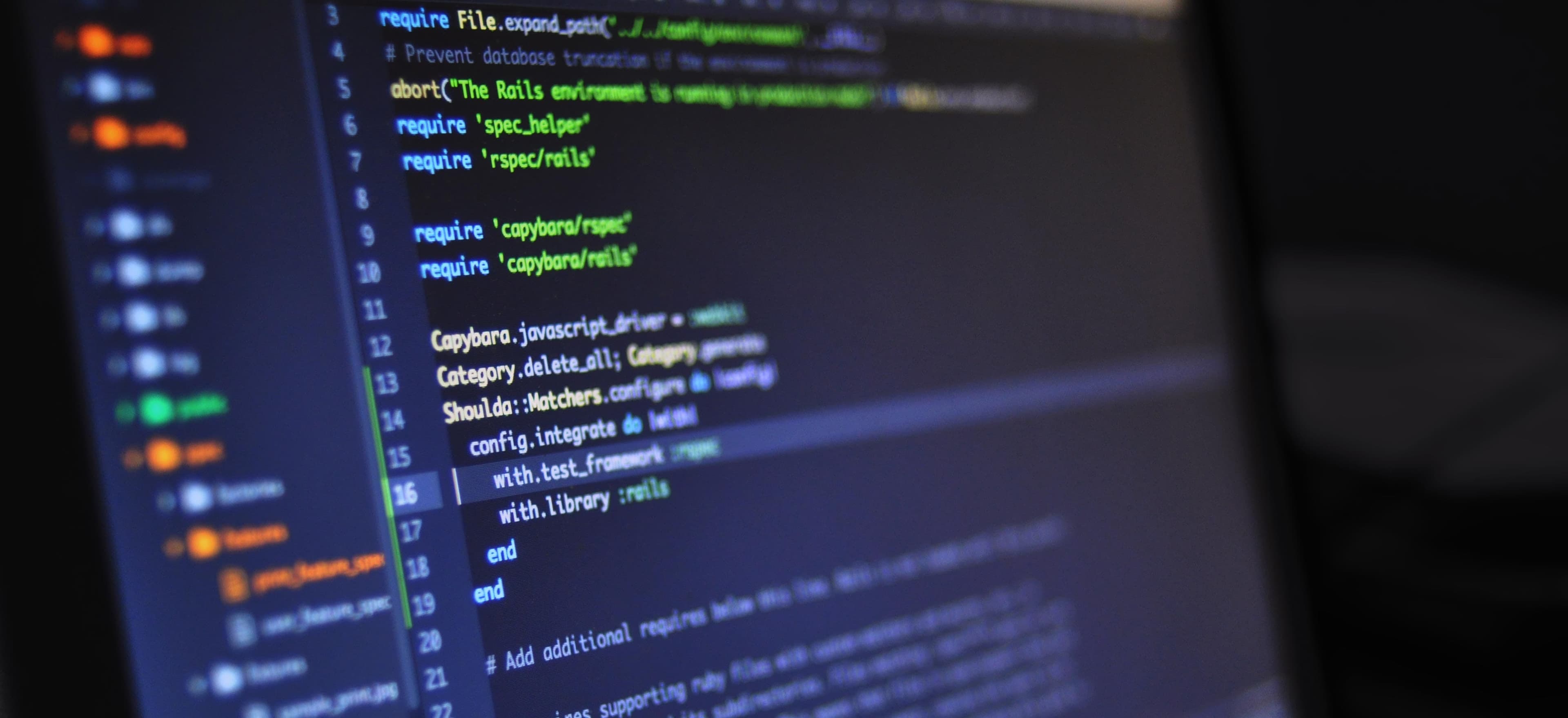
- Published on
Optimizing Slick Database Access on JVM Platform
When it comes to building robust and scalable Java applications, efficient database access is crucial. Slick, a modern database query and access library for Scala, provides a powerful and intuitive way to interact with databases on the JVM platform. In this post, we'll explore techniques to optimize database access using Slick, improving both performance and maintainability of your Java applications.
Understanding Slick
Slick is a functional relational mapping (FRM) library that allows you to work with databases in a type-safe and composable way. It provides a DSL for building and executing database queries, abstracting the underlying SQL and offering a seamless integration with Scala and Java. Slick supports a wide range of database systems including MySQL, PostgreSQL, Oracle, and more.
Leveraging Asynchronous IO
In high-performance applications, leveraging asynchronous IO can significantly improve throughput and responsiveness. Slick provides built-in support for asynchronous database access through Scala's Future
and Await
constructs. By using Future
s, you can execute non-blocking database operations, allowing the application to continue processing other tasks while waiting for the database response.
import scala.concurrent.Future
import scala.concurrent.Await
import scala.concurrent.duration._
val queryResult: Future[Seq[Entity]] = db.run(tableQuery.result)
val result: Seq[Entity] = Await.result(queryResult, 5.seconds)
The code snippet above demonstrates how to execute an asynchronous query using Slick. The db.run
method returns a Future
representing the query execution, and Await.result
is used to block and wait for the result within a specified time frame. However, it's important to handle timeouts and potential failures when using Await
, to prevent blocking the application indefinitely.
Batched Database Operations
When dealing with large datasets, batched database operations can offer significant performance improvements. Slick provides a convenient API for executing batch insert, update, and delete operations, reducing the overhead of individual database round-trips.
val data: Seq[Entity] = // Retrieve data to be inserted
val batchInsertAction = tableQuery ++= data
val insertResult: Future[Option[Int]] = db.run(batchInsertAction)
In the above example, we're using Slick's ++=
operator to concatenate a sequence of entities and perform a batch insert into the database. By batching multiple operations together, we can minimize the number of interactions with the database, thereby improving overall throughput.
Query Optimization and Projection
Efficient database access also involves optimizing the queries themselves. With Slick, you can leverage query projection to fetch only the required columns from the database, reducing the amount of data transferred over the network and improving query performance.
val query = tableQuery.map(_.id) // Projection to retrieve only the 'id' column
val result: Future[Seq[Int]] = db.run(query.result)
By specifying the desired columns in the map
operation, Slick generates optimized SQL queries that fetch only the required data, resulting in more efficient database access. This approach is particularly beneficial when dealing with wide tables and complex entities, as it minimizes unnecessary data retrieval.
Connection Pool Tuning
Configuring the connection pool parameters can have a significant impact on the overall performance and scalability of database access. Slick allows fine-grained control over the connection pool settings, including parameters such as maximum pool size, connection timeout, and validation interval.
val customConfig = DatabaseConfig.forConfig[JdbcProfile]("custom")
val customDb = customConfig.db
// Set custom connection pool parameters
customDb.createSession()
.force() // Ensure the session is initialized
.conn.setAutoCommit(false) // Disable auto-commit mode
.setTransactionIsolation(Connection.TRANSACTION_READ_COMMITTED) // Set transaction isolation level
By customizing the connection pool settings, you can better align the database access patterns with the specific requirements of your application, optimizing resource utilization and mitigating contention issues.
Bringing It All Together
Optimizing database access is a critical aspect of building high-performance Java applications, and Slick provides a potent toolkit for achieving efficient and maintainable database interactions. By leveraging asynchronous IO, batched operations, query optimization, and connection pool tuning, you can maximize the throughput and responsiveness of your applications while ensuring scalability and reliability.
Incorporating these optimization techniques into your Java applications using Slick will not only enhance the overall performance but also contribute to a more resilient and responsive system.
Remember, effective optimization is a continuous process, and it's essential to profile and benchmark the application to identify bottlenecks and fine-tune the database access strategies accordingly.
As you delve deeper into the world of Slick and database optimization on the JVM platform, keep experimenting and refining your approach, always striving for the most efficient and elegant solutions.
Happy optimizing!