The Abstraction Principle: Key Questions & Answers
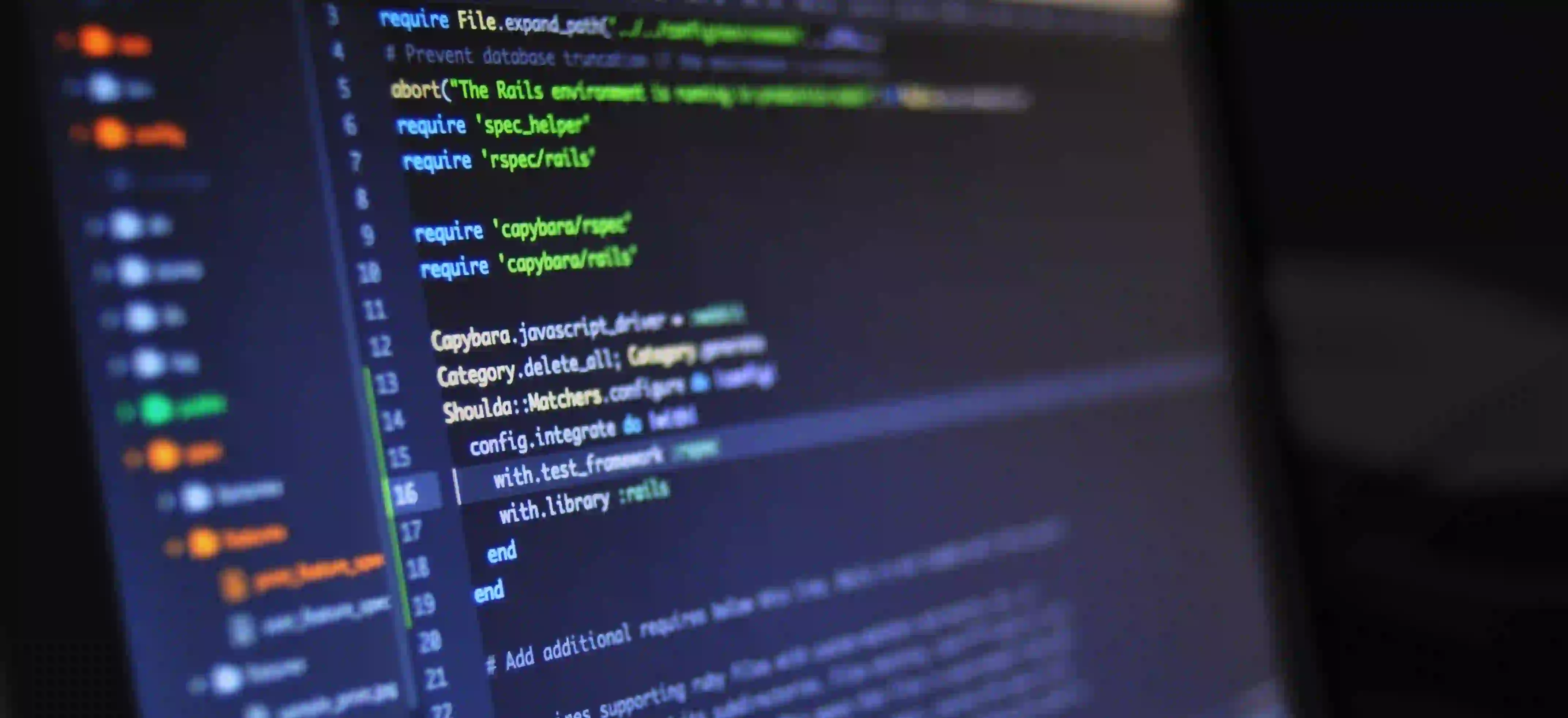
The Abstraction Principle: Key Questions & Answers
When it comes to writing clean, maintainable, and scalable Java code, the Abstraction Principle plays a pivotal role. This principle, which is one of the key components of object-oriented programming, emphasizes the use of abstraction to manage complexity and improve code structure. In this blog post, we will explore the Abstraction Principle in Java, understand its significance, and provide answers to some common questions surrounding this fundamental concept.
What is the Abstraction Principle in Java?
In the context of Java programming, the Abstraction Principle refers to the practice of defining abstract types that encapsulate implementation details while providing a clear and simplified interface for interacting with the underlying functionality. This principle allows developers to create a level of separation between the what and the how of a system, enabling them to focus on high-level design without getting bogged down by implementation specifics.
Why is the Abstraction Principle important?
The Abstraction Principle is vital because it enables developers to build robust and adaptable software systems. By hiding implementation details behind abstractions, developers can reduce dependencies, minimize code duplication, and enhance the maintainability of their codebase. Furthermore, abstraction promotes a clear separation of concerns, allowing different parts of a system to evolve independently without causing ripple effects throughout the code.
How is the Abstraction Principle implemented in Java?
In Java, the Abstraction Principle is implemented using abstract classes and interfaces. Abstract classes provide a way to define classes that cannot be instantiated on their own and may contain abstract methods that must be implemented by derived classes. On the other hand, interfaces define a contract for behaviors without specifying the implementation details, allowing disparate parts of a system to communicate through a common interface.
Example of an abstract class in Java
public abstract class Shape {
public abstract double calculateArea();
}
In this example, the Shape
class is defined as abstract, and it contains an abstract method calculateArea()
. Any concrete subclass of Shape
must provide an implementation for the calculateArea()
method, thus adhering to the Abstraction Principle by separating the definition of the method from its implementation.
Example of an interface in Java
public interface Printable {
void print();
}
In this example, the Printable
interface declares a method print()
without specifying how it should be implemented. This allows various classes to implement the Printable
interface according to their specific printing requirements, promoting code flexibility and reusability.
What are the benefits of applying the Abstraction Principle?
The application of the Abstraction Principle in Java offers several benefits:
- Code Reusability: By defining abstract types and interfaces, developers can create reusable components that can be utilized in various parts of the system.
- Flexibility: Abstraction allows for interchangeable implementations, enabling developers to swap components without affecting the overall system architecture.
- Testability: Abstracting complex logic into well-defined interfaces makes it easier to write unit tests and verify the behavior of the code.
- Maintenance: Abstraction simplifies maintenance efforts by isolating changes to specific components, reducing the risk of unintended side effects.
Common misconceptions about the Abstraction Principle
Misconception 1: Abstraction is all about creating interfaces
While interfaces are a powerful tool for abstraction in Java, the Abstraction Principle is not solely about creating interfaces. It also involves creating abstract classes, defining clear boundaries, and encapsulating common behavior and attributes.
Misconception 2: Abstraction leads to over-engineering
Some developers mistakenly believe that employing abstraction results in over-engineering and unnecessary complexity. However, when applied judiciously, abstraction simplifies code and makes it more understandable by hiding implementation details that are not relevant at a particular level of abstraction.
Best practices for leveraging the Abstraction Principle
- Identify cohesive behaviors and attributes: When designing abstractions, ensure that they represent cohesive sets of behaviors and attributes that are relevant to a specific context.
- Favor composition over inheritance: Prefer using interfaces and composition to define abstractions, as it promotes flexibility and avoids the issues associated with deep inheritance hierarchies.
- Strive for clarity: The abstractions should provide a clear and intuitive interface, enabling developers to understand and use them without delving into unnecessary details.
A Final Look
In conclusion, the Abstraction Principle in Java is a fundamental concept that promotes modularity, reusability, and maintainability in software development. By using abstract classes and interfaces, developers can create well-defined boundaries and clear communication channels between different parts of a system, leading to more robust and adaptable codebases.
Understanding and applying the Abstraction Principle is key to writing clean, scalable, and maintainable Java code, and it is essential for any developer looking to elevate their object-oriented programming skills.
For further reading on the Abstraction Principle and its role in Java development, consider exploring the following resources:
- Oracle's Java Tutorials on Interfaces and Inheritance
- Effective Java by Joshua Bloch (Chapter 4: "Classes and Interfaces")
Stay tuned for more insightful discussions on Java best practices and principles. Happy coding!