Maximizing Container Resource Efficiency
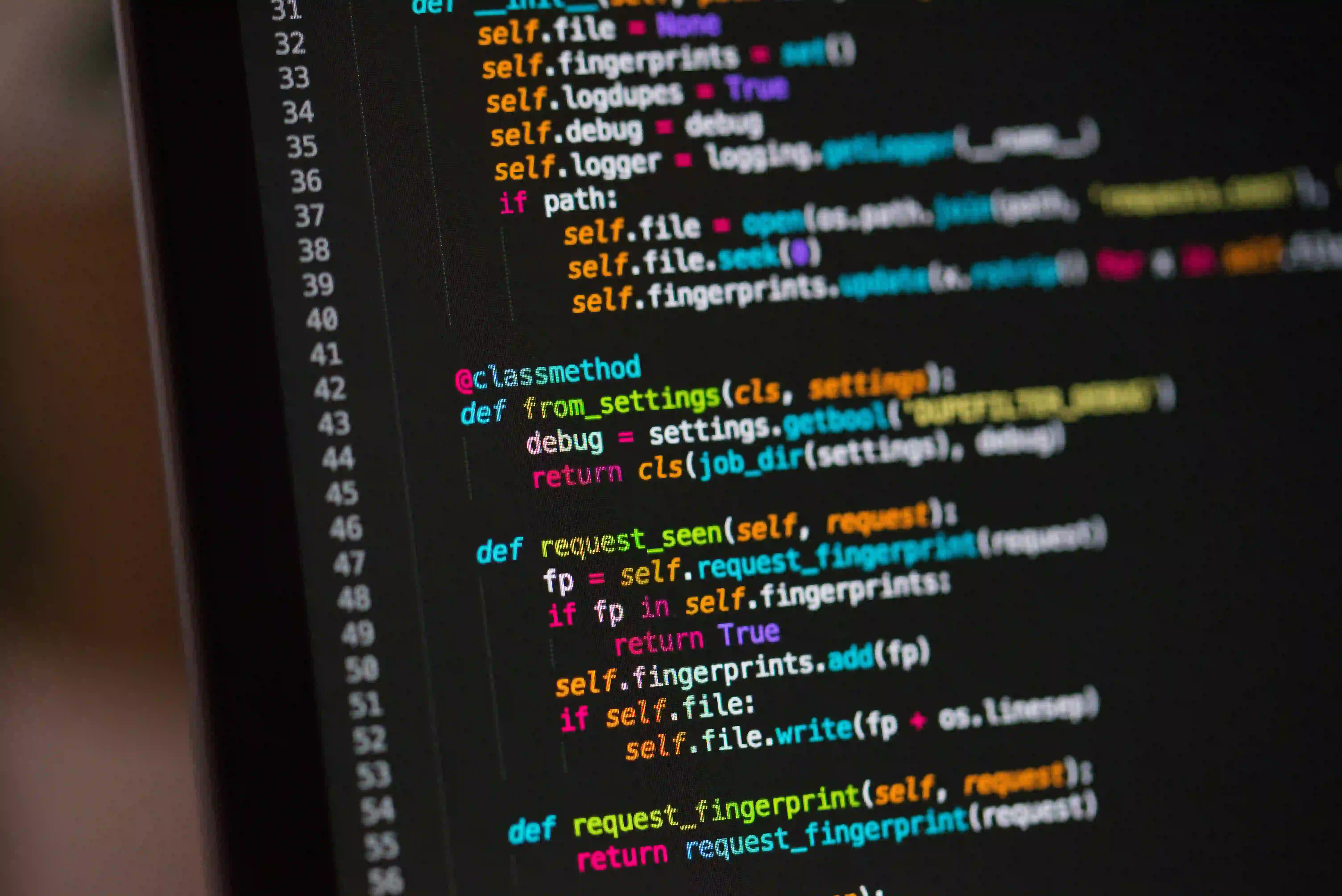
Maximizing Container Resource Efficiency: A Guide for Java Developers
In recent years, containerization has become a popular choice for deploying and managing applications due to its lightweight nature and scalability. However, with great power comes great responsibility, and as a Java developer, it's crucial to ensure that your containers are running efficiently to maximize resource utilization and optimize performance. In this guide, we'll explore various strategies and best practices for maximizing container resource efficiency in the context of Java applications.
Understanding Container Resource Efficiency
When it comes to container resource efficiency, it's essential to focus on three main resources: CPU, memory, and storage. Each plays a critical role in the performance and scalability of your Java applications running within containers.
CPU Utilization
Optimizing CPU utilization involves ensuring that the available CPU resources are effectively used by the running containers without causing resource contention. This includes efficient use of multi-core CPUs, proper load balancing, and minimizing unnecessary CPU spikes.
Memory Management
Proper memory management is crucial for containerized Java applications. Efficient memory allocation and garbage collection strategies are essential to prevent memory leaks and optimize the use of available memory resources.
Storage Optimization
Effective storage management within containers involves minimizing disk I/O, optimizing storage capacity, and implementing efficient data storage strategies to prevent excessive disk space consumption.
Best Practices for Java Container Efficiency
1. Use Lightweight Base Images
When creating Docker images for your Java applications, start with lightweight base images such as Alpine Linux or AdoptOpenJDK to minimize image size and reduce resource overhead. This approach ensures faster image pull times and efficient resource utilization.
FROM adoptopenjdk:11-jre-hotspot
COPY target/my-application.jar /app/app.jar
CMD ["java", "-jar", "/app/app.jar"]
2. Optimize JVM Settings
Tune the Java Virtual Machine (JVM) settings based on the resource requirements of your application. This includes configuring heap size, garbage collection settings, and thread management to align with the available container resources.
java -Xmx256m -Xms128m -XX:+UseG1GC -XX:MaxGCPauseMillis=200
3. Implement Microservices Architecture
Utilize a microservices architecture to decompose your Java application into smaller, independently deployable services. This approach allows for better resource utilization by scaling individual components based on their specific resource demands.
4. Utilize Container Resource Limits
Set resource limits (CPU and memory) for your Java containers using Kubernetes or Docker Compose to prevent resource hogging and ensure fair resource allocation across multiple containers running on the same host.
resources:
limits:
memory: "512Mi"
cpu: "0.5"
5. Leverage Container Orchestration
Use container orchestration platforms like Kubernetes to automate the deployment, scaling, and management of containerized Java applications. Kubernetes provides resource-aware scheduling and efficient utilization of cluster resources.
6. Monitor and Optimize
Implement comprehensive monitoring using tools like Prometheus and Grafana to collect and analyze container resource metrics. Use this data to identify performance bottlenecks and optimize resource utilization iteratively.
Final Thoughts
Efficiently managing resources within containerized Java applications is pivotal for ensuring optimal performance, scalability, and cost-effectiveness. By following the best practices outlined in this guide, Java developers can maximize container resource efficiency and reap the benefits of a well-optimized and high-performing application deployment.
For further insights into optimizing Java applications within containers, stay tuned to our blog for more in-depth articles and tutorials.