Migrating from MySQL to Cassandra: Data Consistency Challenges
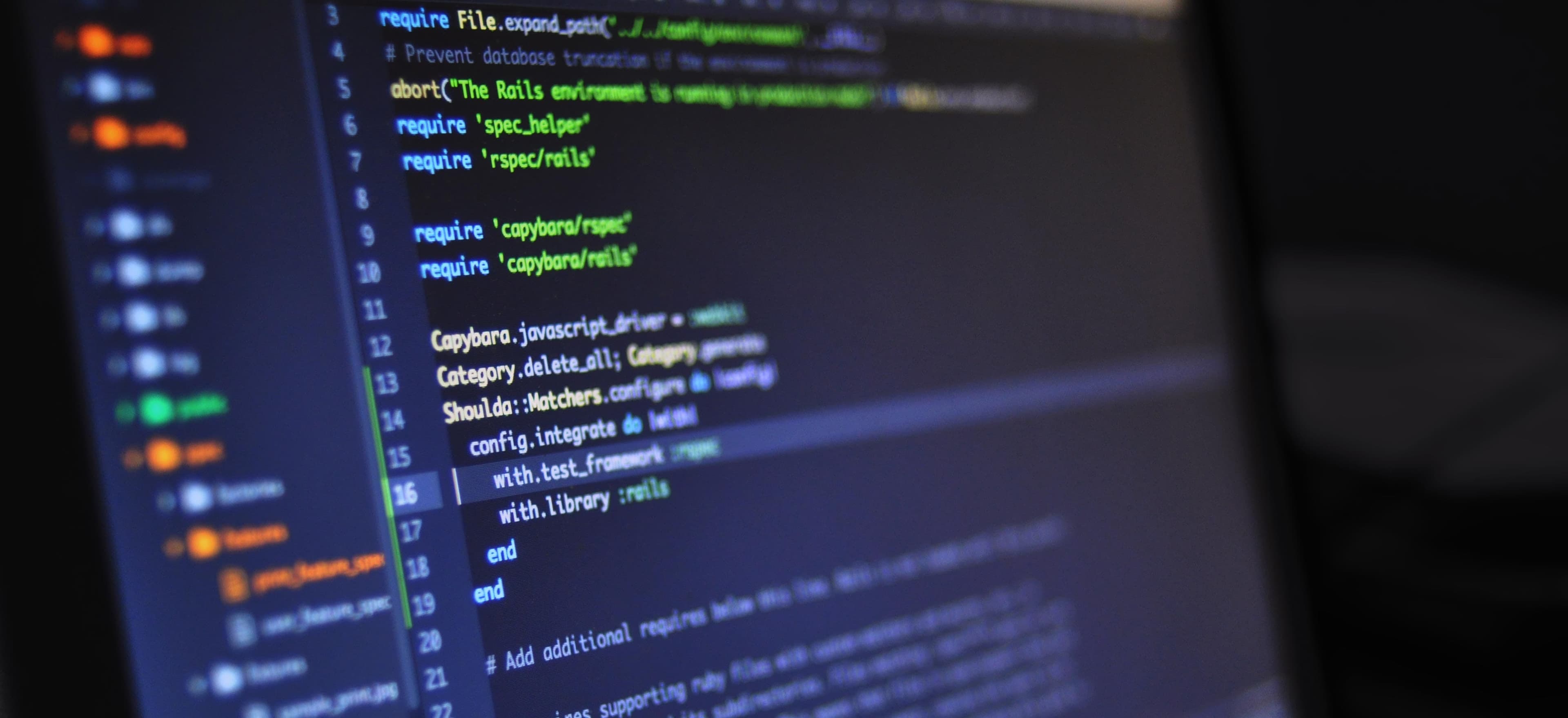
- Published on
Migrating from MySQL to Cassandra: Data Consistency Challenges
Migrating from MySQL to Cassandra can bring a range of advantages such as scalability, high availability, and fault tolerance. However, one of the most significant challenges during this migration process is managing data consistency. In this article, we will explore the data consistency challenges and how to address them when migrating from MySQL to Cassandra.
Understanding Data Consistency
In a MySQL database, data consistency is typically achieved using transactions. When multiple operations are grouped into a transaction, either all of them are successfully applied or none of them are applied. This ensures that the data is always in a consistent state.
Cassandra, on the other hand, is a NoSQL database that offers high availability and partition tolerance at the expense of immediate consistency. It uses eventual consistency, where the data is replicated across multiple nodes over time, thus allowing for eventual consistency across the cluster.
Challenges of Data Consistency in Cassandra
Eventual Consistency
Cassandra’s eventual consistency model can lead to scenarios where a read operation might not reflect the most recent write. This can be challenging when migrating from a system like MySQL, where immediate consistency is the norm.
Data Modeling
In MySQL, data modeling is primarily focused on normalizing the data to minimize redundancy. However, in Cassandra, data modeling revolves around denormalization and optimizing queries for fast reads, leading to potential challenges in maintaining consistency during the migration process.
Transaction Support
While MySQL supports ACID transactions, Cassandra supports atomicity at the row level but does not support multi-row transactions. This can lead to challenges when ensuring consistency across multiple rows during the migration process.
Addressing Data Consistency Challenges
Use of Lightweight Transactions
Cassandra provides support for lightweight transactions using the IF
clause in CQL (Cassandra Query Language). By using lightweight transactions, you can enforce conditions on insert or update operations, thus ensuring data consistency for critical operations.
// Example of using lightweight transactions in CQL
session.execute("BEGIN BATCH \n" +
"UPDATE table_name SET column1 = value1 WHERE condition IF column1 = previous_value;\n" +
"APPLY BATCH;");
Implementing Compensating Transactions
During the migration process, it's essential to identify scenarios where compensating transactions can be applied. Compensating transactions help in reverting or adjusting the data to maintain consistency when inconsistencies are encountered.
// Example of compensating transaction
try {
// Perform data migration
// ...
} catch (Exception e) {
// Perform compensating transaction to revert the changes
// ...
}
Leveraging Time-to-Live (TTL) for Data Expiration
In Cassandra, Time-to-Live (TTL) allows data to expire after a certain period, ensuring that outdated data does not impact the consistency of the system. Leveraging TTL for specific data sets can help in managing data consistency during the migration.
// Example of using TTL for data expiration
session.execute("INSERT INTO table_name (column1, column2) VALUES (value1, value2) USING TTL 86400;");
Monitoring and Resolving Consistency Issues
Throughout the migration process, it's crucial to monitor data consistency using tools like nodetool
and resolve any inconsistencies that arise. Understanding the distribution of data across the cluster and using repair operations can help in maintaining consistency.
Wrapping Up
Migrating from MySQL to Cassandra presents a range of data consistency challenges due to the fundamental differences in the underlying data models and consistency models. By leveraging lightweight transactions, compensating transactions, TTL, and proactive monitoring, these challenges can be effectively addressed, ensuring a smooth and consistent migration process.
In conclusion, understanding the nuances of data consistency in Cassandra and proactively addressing the challenges can pave the way for a successful migration, enabling organizations to harness the scalability and fault tolerance offered by Cassandra while maintaining data consistency.
To delve deeper into data consistency in Cassandra, check out DataStax’s comprehensive guide on data consistency levels in Cassandra.
Feel free to share your experiences and thoughts on managing data consistency during database migrations in the comments section below!