Building Reactive Microservices with Ktor
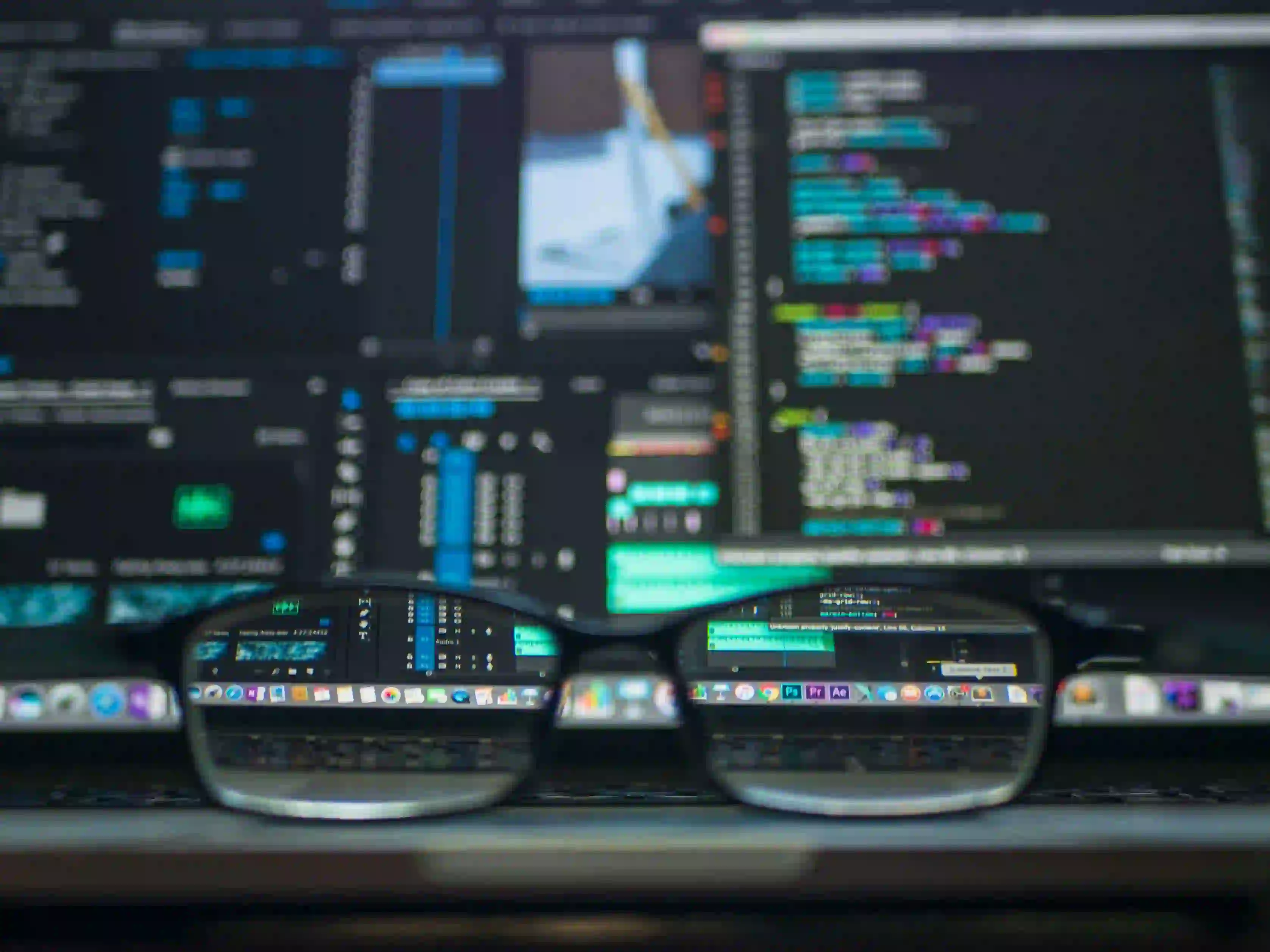
Building Reactive Microservices with Ktor
Reactive microservices have become increasingly popular due to their scalability, resilience, and performance. Building reactive microservices in Java involves selecting the right framework to handle the asynchronous and non-blocking nature of reactive programming. In this blog post, we will explore how to build reactive microservices in Java using Ktor, a modern asynchronous web framework.
What is Ktor?
Ktor is a framework for building asynchronous servers and clients in connected systems using Kotlin, which is interoperable with Java. It is built from the ground up to take advantage of Kotlin's expressive syntax and provides a concise and powerful API for building web applications, including reactive microservices. Ktor is lightweight, easy to use, and integrates seamlessly with coroutines to handle asynchronous operations.
Setting Up the Project
To get started with building reactive microservices using Ktor, first, we need to set up a new project. We can use Gradle as the build tool and include the Ktor server dependency.
// build.gradle.kts
plugins {
kotlin("jvm") version "1.5.31"
id("application")
}
repositories {
mavenCentral()
}
dependencies {
implementation("io.ktor:ktor-server-netty:$ktor_version")
implementation("io.ktor:ktor-server-core:$ktor_version")
implementation("io.ktor:ktor-gson:$ktor_version")
}
application {
mainClassName = "com.example.ApplicationKt"
}
In the build.gradle.kts
file, we specify the Ktor dependencies for the server and Gson for JSON serialization/deserialization. Additionally, we set the main class for the application.
Creating a Simple Reactive Microservice
Let's create a simple reactive microservice using Ktor. We will create an endpoint that returns a list of items asynchronously.
// Application.kt
import io.ktor.application.*
import io.ktor.features.ContentNegotiation
import io.ktor.gson.gson
import io.ktor.response.*
import io.ktor.routing.*
import io.ktor.server.engine.*
import io.ktor.server.netty.*
import kotlinx.coroutines.delay
import kotlinx.coroutines.runBlocking
fun main() {
val server = embeddedServer(Netty, port = 8080) {
install(ContentNegotiation) {
gson {
setPrettyPrinting()
}
}
routing {
get("/items") {
val items = runBlocking {
delay(1000) // Simulate asynchronous operation
listOf("item1", "item2", "item3")
}
call.respond(items)
}
}
}
server.start(wait = true)
}
In this example, we create a simple Ktor server that responds to the /items
endpoint with a list of items. To simulate an asynchronous operation, we use delay
from kotlinx.coroutines
. When a request is made to the /items
endpoint, the server delays for 1 second before responding with the list of items.
Handling Asynchronous Operations
Reactive microservices often need to handle asynchronous operations such as database queries, external API calls, or long-running computations. Ktor integrates seamlessly with Kotlin coroutines to handle asynchronous operations in a concise and expressive manner.
// Application.kt
import io.ktor.application.*
import io.ktor.features.ContentNegotiation
import io.ktor.gson.gson
import io.ktor.response.*
import io.ktor.routing.*
import io.ktor.server.engine.*
import io.ktor.server.netty.*
import kotlinx.coroutines.delay
import kotlinx.coroutines.launch
import kotlinx.coroutines.runBlocking
fun main() {
val server = embeddedServer(Netty, port = 8080) {
install(ContentNegotiation) {
gson {
setPrettyPrinting()
}
}
routing {
get("/items") {
val items = runBlocking {
val deferred = mutableListOf<Deferred<String>>()
repeat(3) {
deferred.add(launch {
delay(1000) // Simulate asynchronous operation
"item$it"
})
}
deferred.map { it.await() }
}
call.respond(items)
}
}
}
server.start(wait = true)
}
In this modified example, we use launch
to asynchronously create three items, each with a simulated delay of 1 second, and then await their completion. This showcases Ktor's ability to handle multiple asynchronous operations concurrently while ensuring responsiveness and efficiency.
Wrapping Up
In this blog post, we've explored how to build reactive microservices in Java using the Ktor framework. We've seen how Ktor's integration with Kotlin coroutines enables concise and expressive handling of asynchronous operations. With its lightweight and intuitive approach, Ktor proves to be an excellent choice for building reactive microservices.
Reactive microservices are a key component of modern, scalable, and resilient systems, and Ktor empowers developers to build such systems with ease. By leveraging Ktor's capabilities for asynchronous and non-blocking operations, developers can create high-performance microservices that are well-suited for the demands of today's distributed systems.
By embracing Ktor's capabilities and best practices for reactive microservices, developers can ensure that their systems are responsive, resilient, and capable of handling high loads with ease.
In conclusion, Ktor offers a modern, efficient, and hassle-free approach to building reactive microservices in Java.
Start building your reactive microservices with Ktor and experience the power of asynchronous, non-blocking, and scalable web development in Java.