Handling Java 8 Lambda Expressions in JUnit Exception Testing
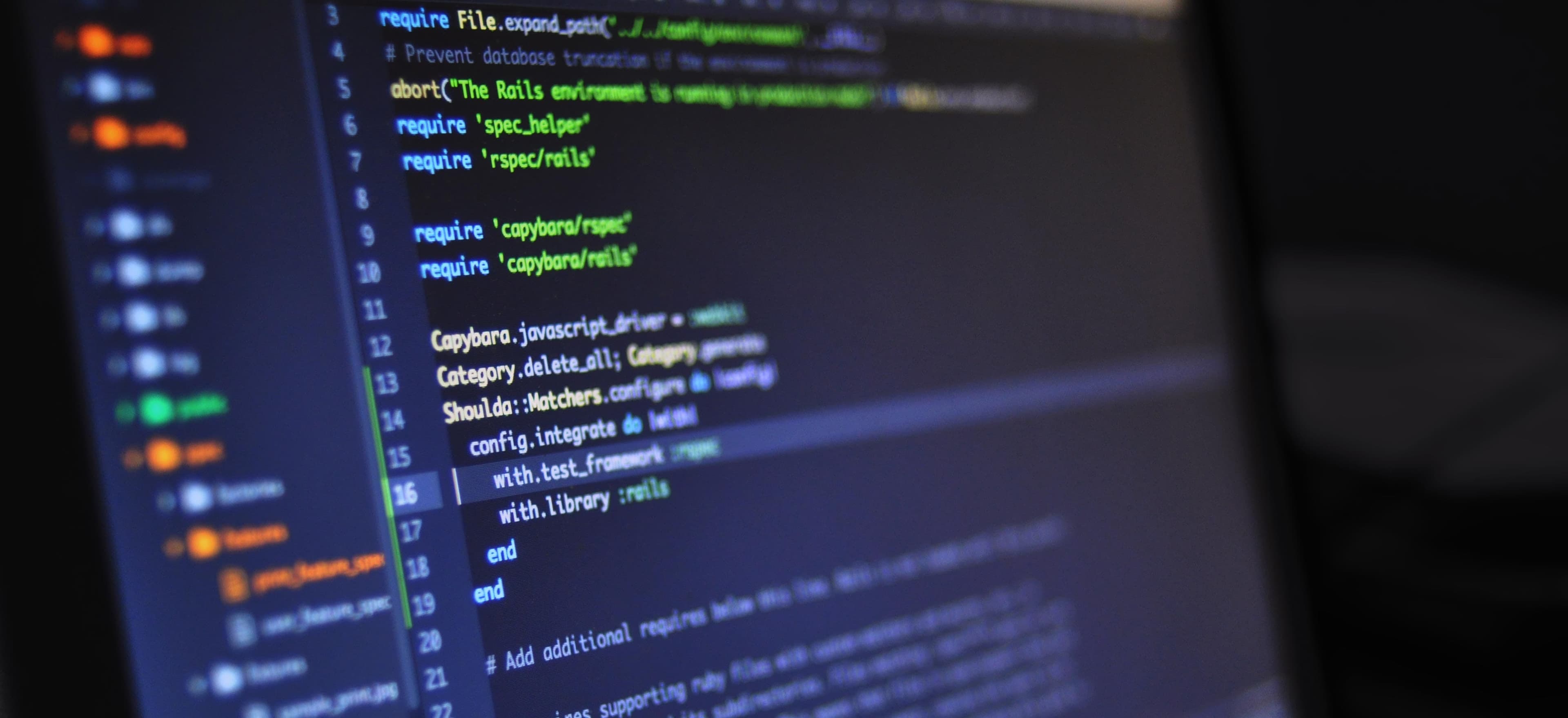
- Published on
When working with Java, handling exceptions is an integral part of writing reliable code. One common tool for testing exception handling is the JUnit framework. In this post, we will delve into using Java 8 Lambda expressions with JUnit to test exception scenarios.
Understanding Lambda Expressions
Lambda expressions introduce a new syntax for writing anonymous methods in Java. They facilitate functional programming by treating functions as first-class citizens. In simpler terms, Lambda expressions allow us to pass functionality as an argument to a method, such as in the case of exception testing in JUnit.
Setting Up Dependencies
Before we dive into exception testing with Lambda expressions, make sure you have the necessary dependencies set up in your project.
Here's a build.gradle
example:
testImplementation 'junit:junit:4.12'
testCompile 'org.assertj:assertj-core:3.11.1'
The above configuration includes JUnit as the testing framework and AssertJ for fluent assertions.
Testing Exceptions with JUnit 4
Traditionally, testing for exceptions in JUnit involved using the @Test
annotation in conjunction with the expected
attribute. However, this approach has limitations. Here’s how you would typically test for an exception using the expected
attribute:
@Test(expected = MyException.class)
public void testException() {
// Test logic that should throw MyException
}
Although this approach works, it has a drawback – it doesn’t allow us to perform assertions on the exception itself. This is where Lambda expressions come into play.
Leveraging Lambda Expressions for Exception Testing
Starting with Java 8, we can use Lambda expressions within JUnit to handle exceptions more effectively. The assertThrows
method from JUnit's Assertions
class allows us to validate both the occurrence of an exception and its content. Here is an example of how to use Lambda expressions with assertThrows
:
@Test
public void testExceptionWithLambdaExpression() {
MyService myService = new MyService();
Throwable exception = assertThrows(MyException.class, () -> myService.methodThatThrowsException());
assertEquals("Exception message", exception.getMessage());
// Additional assertions on the exception
}
In the example above, the assertThrows
method takes two arguments: the expected exception type and a lambda expression representing the code that should throw the exception. We then perform assertions on the caught exception, such as checking its message or any custom properties.
Advantages of Using Lambda Expressions
Using Lambda expressions for exception testing provides several benefits:
- It allows for more granular assertions on the exception, ensuring that the correct exception is thrown with the expected properties.
- The test remains concise and readable, as the exception-handling logic is encapsulated within the lambda expression.
- Lambda expressions promote a more functional approach to writing tests, making the codebase more expressive and maintainable.
Combining with AssertJ for Enhanced Assertions
AssertJ is a popular assertion library that provides a fluent, easy-to-read way of expressing assertions in tests. When combined with Lambda expressions, AssertJ can further enhance the readability and expressiveness of our exception tests.
Here’s an example of using AssertJ along with Lambda expressions for exception testing:
@Test
public void testExceptionWithAssertJ() {
MyService myService = new MyService();
assertThatThrownBy(myService::methodThatThrowsException)
.isInstanceOf(MyException.class)
.hasMessage("Exception message");
// Additional assertions using AssertJ
}
In this example, the assertThatThrownBy
method from AssertJ allows us to perform assertions specifically on the thrown exception. We can use chained methods like isInstanceOf
and hasMessage
to make the test more descriptive and insightful.
Best Practices and Considerations
While using Lambda expressions for exception testing, it’s crucial to keep some best practices in mind:
- Use descriptive messages in assertions to provide clear feedback when tests fail.
- Avoid placing complex logic within the lambda expression, as it may hinder readability and maintainability.
- Exercise caution when asserting multiple properties of an exception, as it could lead to overspecifying the test.
Wrapping Up
In conclusion, Java 8 Lambda expressions provide a powerful mechanism for testing exceptions in conjunction with JUnit. They allow us to write more expressive, readable, and maintainable tests, promoting a functional approach to handling exceptions. By combining Lambda expressions with libraries like AssertJ, the process of testing and asserting exceptions becomes even more streamlined and insightful.
Embracing the functional capabilities of Java 8 can greatly enhance the way we approach exception testing, leading to more robust and reliable code.
Incorporate Lambda expressions and AssertJ into your exception testing arsenal, and experience a more streamlined and expressive way of handling exceptions in your Java projects!