Handling Null Values in Java 8's Optional
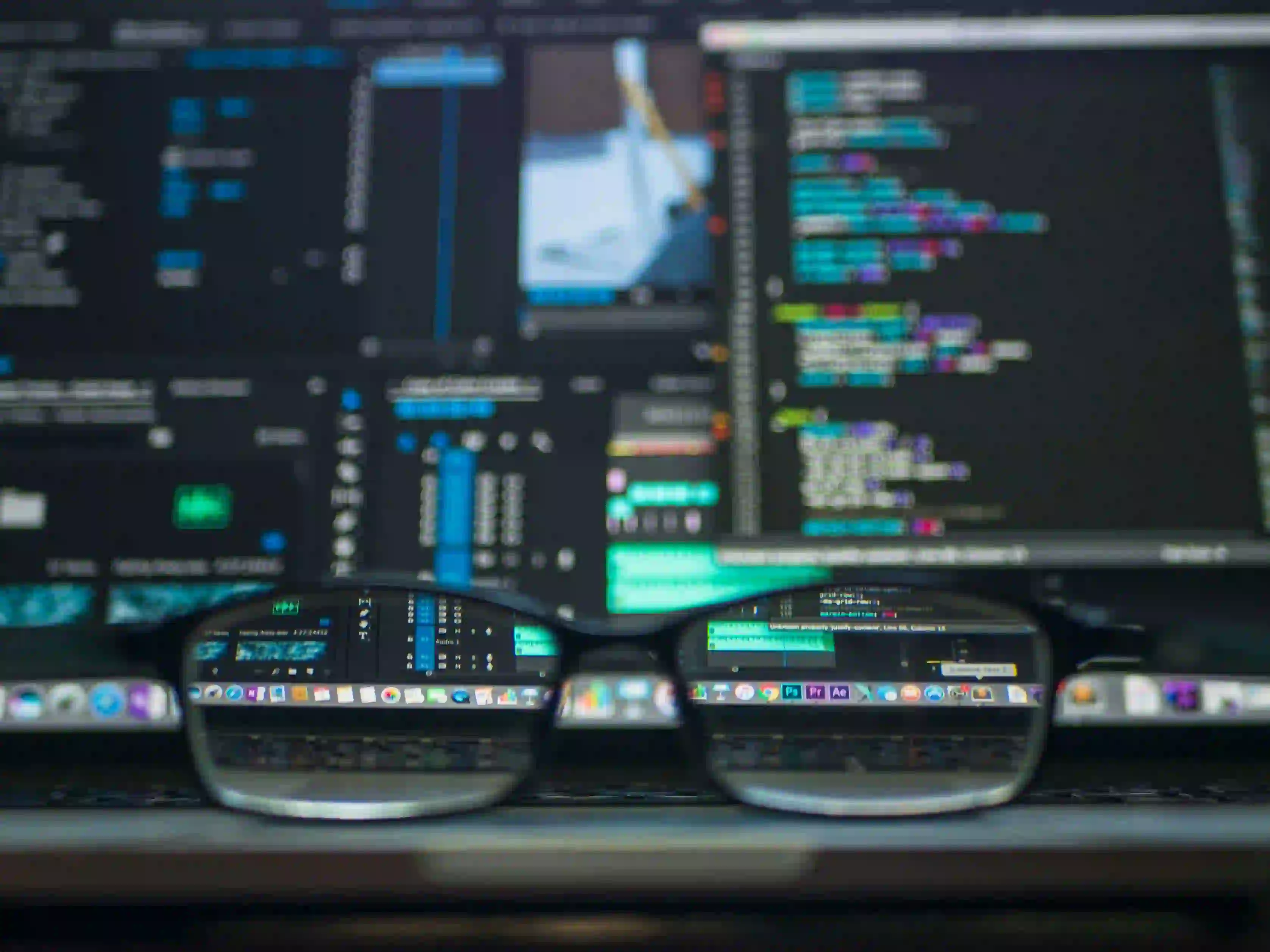
Handling Null Values in Java 8's Optional
In Java programming, dealing with null values is a common challenge. Null pointer exceptions are a notorious source of bugs, often leading to crashes and unexpected behavior. In Java 8, the introduction of the Optional
class provides a more elegant way to handle null values, offering a cleaner, more concise, and less error-prone alternative to null checks. This article explores the power of Optional
and how it can be effectively used to handle null values in Java applications.
Understanding the Problem with Null
In Java, null is often used to denote the absence of a value. However, the widespread use of null can result in code that is difficult to understand, reason about, and maintain. Null checks are commonly scattered throughout the code, leading to code clutter and greater potential for errors. Let's consider the following example:
String name = getName();
if (name != null) {
System.out.println("Name is: " + name);
} else {
System.out.println("Name is not available");
}
The code above contains a typical null check, and while it does the job, it adds clutter to the code. Additionally, if getName()
returns null, the handling of the null value is not centralized and can lead to code duplication.
Introducing Java 8's Optional
Java 8 introduced the Optional
class as a container object which may or may not contain a non-null value. This allows for a more expressive way of handling the absence of a value, without the need for explicit null checks. Let's rewrite the previous example using Optional
:
Optional<String> nameOptional = Optional.ofNullable(getName());
nameOptional.ifPresentOrElse(
name -> System.out.println("Name is: " + name),
() -> System.out.println("Name is not available")
);
In this updated version, the use of Optional
eliminates the need for an explicit null check and the code is more expressive. By doing so, the handling of null values is centralized, improving code clarity and reducing the risk of null pointer exceptions.
Dealing with Nulls Using Optional
Now that we understand the basics of Optional
, let's delve deeper into how it can be used to effectively handle null values.
Creating Optional Instances
The Optional
class provides several static factory methods to create instances of Optional
, such as empty()
, of(value)
, and ofNullable(value)
. Here's how you can create Optional
instances:
Optional<String> emptyOptional = Optional.empty();
Optional<String> nonNullOptional = Optional.of("Hello, Optional!");
Optional<String> nullableOptional = Optional.ofNullable(getNullableValue());
It's important to note that using of(value)
with a null value will result in a NullPointerException
, whereas ofNullable(value)
can handle null values by creating an empty Optional
.
Accessing the Value
Instead of directly accessing the value within an Optional
, the class provides methods like ifPresent()
, orElse()
, orElseGet()
, and orElseThrow()
to safely access the value or provide a default value or behavior if the optional value is empty. Here's an example:
Optional<String> optionalValue = Optional.ofNullable(getValue());
optionalValue.ifPresent(val -> System.out.println("Value is: " + val));
String result = optionalValue.orElse("Default Value");
Chaining Operations
Optional
also supports functional-style methods for chaining operations, such as map()
, flatMap()
, and filter()
. These methods allow for the transformation, composition, and filtering of optional values. Let's look at an example using map()
:
Optional<String> value = Optional.ofNullable(getValue());
Optional<Integer> length = value.map(String::length);
In this example, the map()
method applies the String::length
function to the value inside the optional and returns another optional containing the result.
By using these methods, it's possible to perform various operations on the optional value without exposing potential null pointer exceptions.
Best Practices and Considerations
While Optional
provides a powerful tool for handling null values, it's important to use it judiciously and consider best practices:
- Avoid Overuse: Use
Optional
where it provides clarity and enhances code readability, such as in public APIs. However, using it excessively within an application's internal code may lead to unnecessary complexity. - Consider Performance: As with any abstraction, there is a slight performance cost to using
Optional
. For performance-critical portions of code, it's worth considering whether the use ofOptional
is appropriate. - Avoid Nesting Optionals: Nesting optionals can lead to less readable code and increase complexity. If a method returns an optional, consider using flatMap instead of nesting optionals.
Bringing It All Together
Java 8's Optional
class provides a modern approach to handling null values, offering a more expressive, concise, and safer alternative to traditional null checks. By leveraging Optional
, developers can write cleaner, more resilient code that minimizes the risk of null pointer exceptions.
Incorporating Optional
into your codebase can lead to clearer and more maintainable code, and when used judiciously, it can greatly improve the robustness and reliability of Java applications.
In conclusion, the adoption of Optional
allows for a paradigm shift in handling null values, promoting code clarity, reducing bugs, and enabling safer and more maintainable Java codebases.
To further deepen your understanding, you can also explore the various methods that Optional
provides in the Java 8 documentation and consider best practices outlined in the Effective Java book.