Choosing Strong Passwords for Encryption Keys
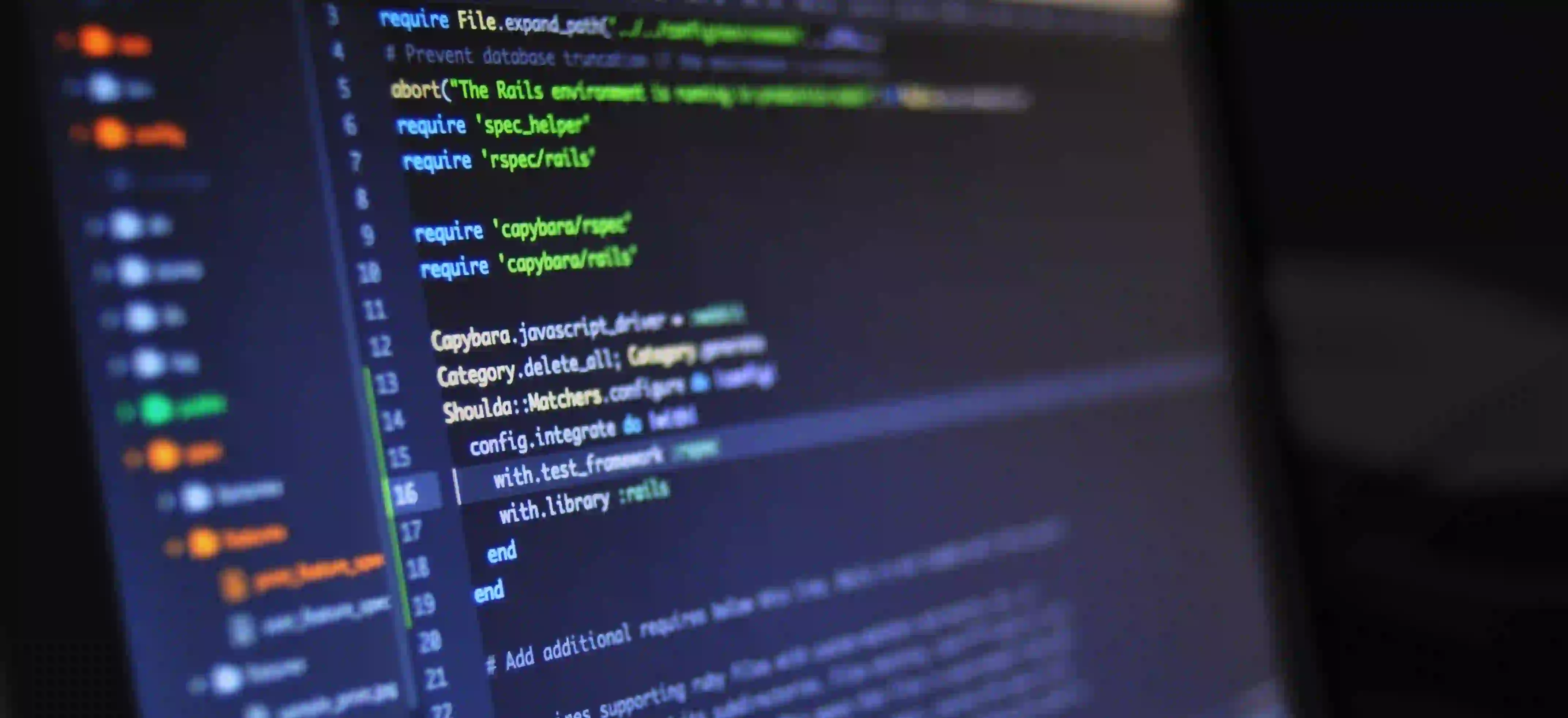
The Importance of Strong Passwords for Encryption Keys in Java
In the realm of software development, security is paramount. Protecting sensitive data from unauthorized access is crucial, and one way to achieve this is through encryption. In Java, encryption is commonly used to secure data by converting it into a form that can only be read with the appropriate decryption key. However, the strength of this security is heavily dependent on the password used to generate the encryption key. In this article, we will explore the significance of strong passwords for encryption keys in Java and provide insights into best practices.
Understanding Encryption Keys in Java
In Java, encryption keys are often derived from passwords using algorithms such as PBKDF2 (Password-Based Key Derivation Function 2). A strong password plays a critical role in the generation of a secure encryption key. It is important to note that a weak password can undermine the entire encryption process, making the data vulnerable to unauthorized access.
The Characteristics of a Strong Password
A strong password for encryption keys should exhibit the following characteristics:
- Length: A longer password is generally more secure as it provides a larger pool of possible combinations, making it harder to crack.
- Complexity: Including a mix of uppercase letters, lowercase letters, numbers, and special characters enhances the complexity of the password, making it more resilient against various attack methods such as brute forcing.
- Unpredictability: A strong password should not be easily guessable and should not be based on readily available personal information like names, birthdates, or common words.
Generating Strong Passwords in Java
In Java, the SecureRandom
class can be utilized to generate strong random passwords. Here’s an example of how it can be used:
import java.security.SecureRandom;
import java.math.BigInteger;
public class StrongPasswordGenerator {
private static SecureRandom secureRandom = new SecureRandom();
public static String generateStrongPassword() {
return new BigInteger(130, secureRandom).toString(32);
}
public static void main(String[] args) {
String strongPassword = generateStrongPassword();
System.out.println("Generated Strong Password: " + strongPassword);
}
}
In this example, the SecureRandom
class is used to generate a strong, random password. The BigInteger
class creates a random integer and then converts it to a base-32 string, resulting in a strong password.
Implementing Password-Based Key Derivation in Java
In Java, the javax.crypto
package provides the necessary classes for implementing password-based key derivation. Here’s a basic example demonstrating how to derive an encryption key from a password using the PBKDF2 algorithm:
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.PBEKeySpec;
import java.security.spec.KeySpec;
import java.security.NoSuchAlgorithmException;
import java.security.spec.InvalidKeySpecException;
import java.security.SecureRandom;
import java.security.spec.InvalidKeyLengthException;
public class EncryptionKeyDerivation {
public static byte[] deriveKey(String password, byte[] salt) {
int iterations = 10000; // Number of iterations
int keyLength = 256; // Key length in bits
KeySpec keySpec = new PBEKeySpec(password.toCharArray(), salt, iterations, keyLength);
try {
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("PBKDF2WithHmacSHA256");
return keyFactory.generateSecret(keySpec).getEncoded();
} catch (NoSuchAlgorithmException | InvalidKeySpecException e) {
// Handle exceptions
return new byte[0];
}
}
public static void main(String[] args) {
String password = "StrongPassword123!";
byte[] salt = new byte[16]; // Generate salt
SecureRandom secureRandom = new SecureRandom();
secureRandom.nextBytes(salt);
byte[] encryptionKey = deriveKey(password, salt);
System.out.println("Derived Encryption Key: " + javax.xml.bind.DatatypeConverter.printHexBinary(encryptionKey));
}
}
In this example, the PBEKeySpec
class is utilized to create a key specification with the provided password and salt. The SecretKeyFactory
class then generates the encryption key using the PBKDF2WithHmacSHA256 algorithm.
Best Practices for Securing Passwords
When it comes to securing passwords for encryption keys in Java, the following best practices should be considered:
- Never store passwords in plaintext. Instead, store salted hashes of passwords to prevent direct exposure.
- Utilize a secure and well-established password hashing algorithm like bcrypt or Argon2 to hash the passwords.
- Implement appropriate techniques for managing and protecting passwords, such as using secure storage mechanisms and following secure coding practices.
Key Takeaways
In conclusion, the strength of encryption in Java heavily relies on the robustness of the passwords used to derive encryption keys. By generating strong passwords and implementing sound practices for deriving encryption keys from passwords, developers can bolster the security of their applications and safeguard sensitive data from potential breaches. It is imperative to continuously stay abreast of the evolving landscape of security threats and adhere to best practices for ensuring robust password security in Java applications.
In the ever-changing landscape of cybersecurity, staying informed about best practices for password security in Java is crucial. By implementing the techniques and best practices outlined in this article, developers can fortify the security of their applications and protect sensitive data from unauthorized access.