5 Essential Product Discovery Tips
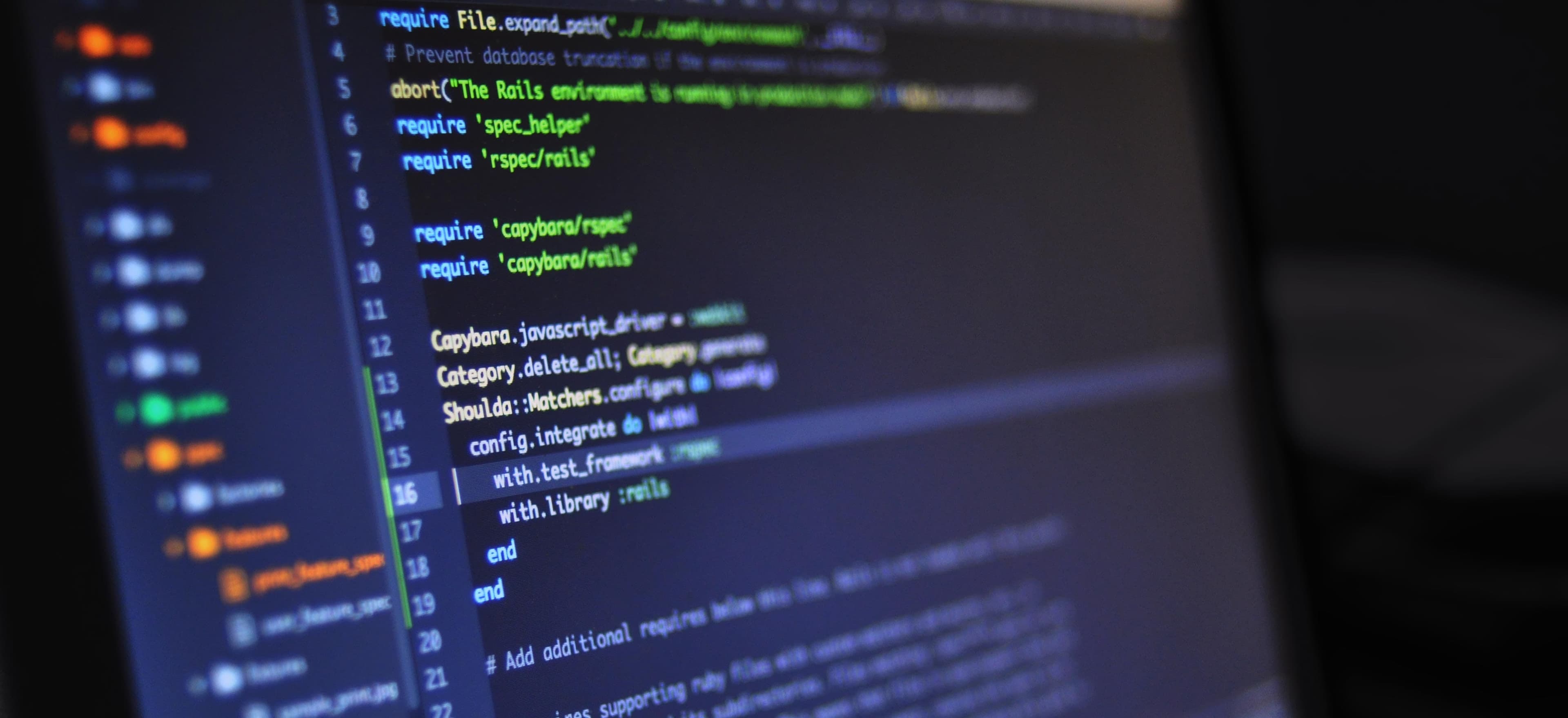
- Published on
5 Essential Product Discovery Tips
Product discovery is a crucial phase in the development of any software product. It involves identifying and defining the features and capabilities that will make the product successful. In this article, we'll discuss five essential tips for effective product discovery in Java development.
1. Understand the Problem Domain
Before diving into code, it's important to have a deep understanding of the problem domain. This involves understanding the market, the target audience, and the specific pain points that the product aims to solve. In Java development, this understanding helps in defining the scope of the project and identifying the most appropriate technical solutions.
// Example of problem domain understanding in Java
public class OrderService {
public List<Order> getOrdersByCustomer(Customer customer) {
// Business logic to retrieve orders for a specific customer
}
}
In the above Java code snippet, understanding the problem domain is crucial for defining the OrderService
class and its methods.
2. Collaborate with Stakeholders
Effective product discovery requires collaboration with stakeholders, including product managers, designers, developers, and end users. In Java development, involving stakeholders in the discovery process can help in gathering diverse perspectives and insights that contribute to a more comprehensive understanding of the product requirements.
// Example of stakeholder collaboration in Java
public class UserController {
public void createUser(User user) {
// Method to create a new user
}
}
Involving stakeholders in the design of the UserController
helps in ensuring that the requirements align with the needs of end users.
3. Prioritize Features
Not all features are created equal. Prioritizing features is essential in product discovery to focus development efforts on the most valuable aspects of the product. In Java development, this involves analyzing the technical complexity and business impact of each feature to determine the order in which they should be built.
// Example of feature prioritization in Java
public class ProductService {
public Product getProductById(String productId) {
// Method to retrieve product information by ID
}
}
Prioritizing features for the ProductService
helps in determining which product information should be retrieved first based on business value.
4. Validate Assumptions through Prototyping
Prototyping is a crucial step in product discovery to validate assumptions and gather early feedback. In Java development, creating prototypes allows for quick iteration and refinement of the product concept before investing significant resources into full-scale development.
// Example of prototyping in Java
public interface PaymentGateway {
boolean processPayment(Order order, PaymentDetails paymentDetails);
}
Creating a prototype for the PaymentGateway
interface helps in validating assumptions about the payment processing flow.
5. Embrace Agile Principles
Adopting agile principles in product discovery promotes flexibility and continuous improvement. In Java development, embracing agile practices such as iterative development, frequent feedback loops, and adaptive planning allows for efficient and responsive product discovery processes.
// Example of embracing agile principles in Java
public class SprintBacklog {
private List<UserStory> userStories;
// Other relevant data and methods related to the sprint backlog
}
Embracing agile principles in the design of the SprintBacklog
facilitates the iterative and adaptive nature of product discovery.
In conclusion, effective product discovery in Java development requires a deep understanding of the problem domain, collaboration with stakeholders, feature prioritization, validation through prototyping, and the embrace of agile principles. By following these essential tips, Java developers can ensure that their product discovery process sets the stage for successful product development.