Handling JSON Serialization and Deserialization in GWT
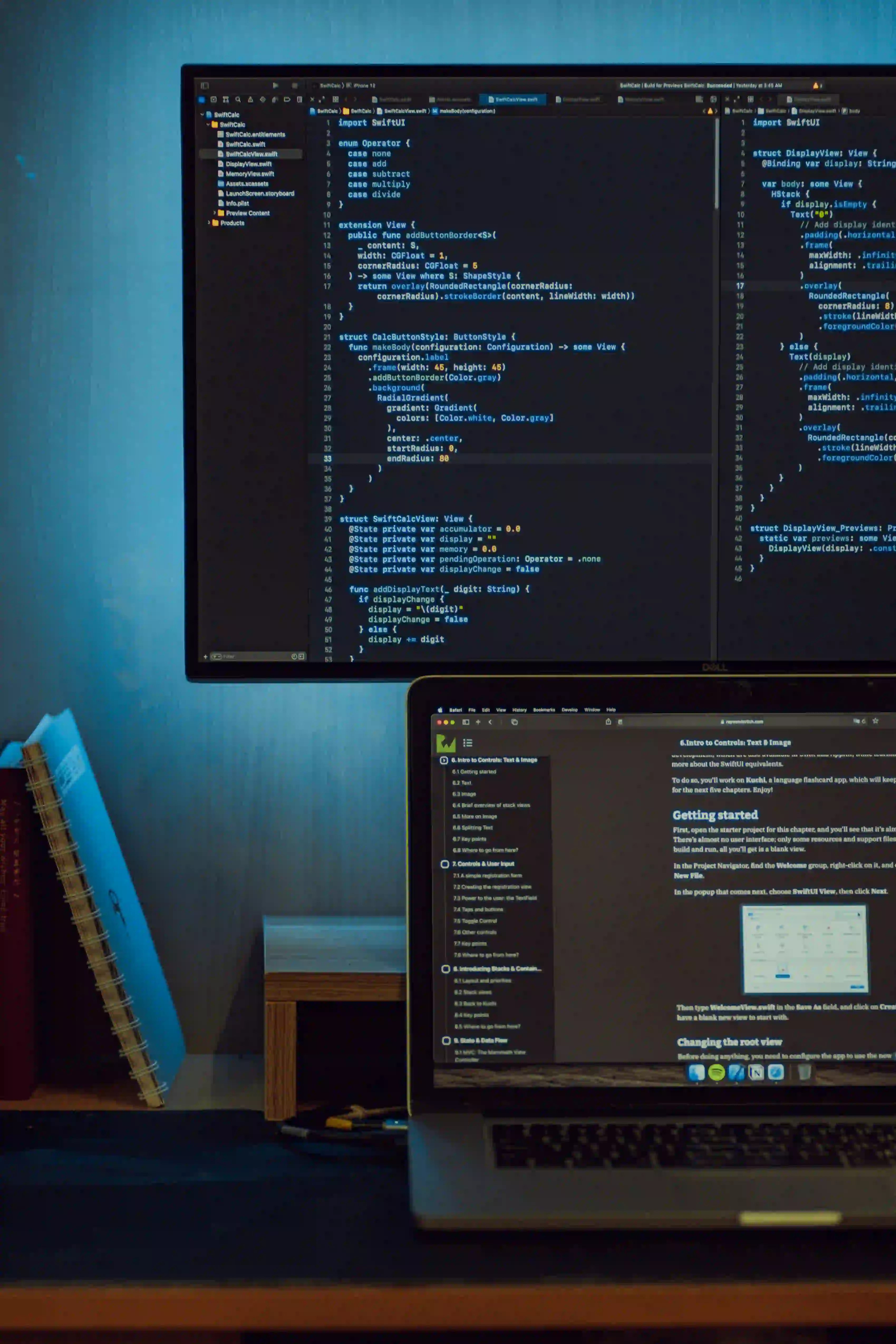
A Comprehensive Guide to Handling JSON Serialization and Deserialization in GWT
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. In the context of web development, JSON plays a crucial role in data transmission between the client and the server.
When working with GWT (Google Web Toolkit), handling JSON serialization and deserialization is an essential part of building modern web applications. In this guide, we will explore how to effectively manage JSON data in GWT applications, including the serialization and deserialization processes.
Understanding JSON Serialization and Deserialization
What is JSON Serialization?
JSON serialization in GWT refers to the process of converting Java objects into their JSON representation. This is particularly important when sending data from the server to the client, such as in the case of AJAX requests or when populating client-side models.
What is JSON Deserialization?
On the other hand, JSON deserialization involves the conversion of JSON data into Java objects. This is critical when processing data received from the client, for instance, when handling form submissions or processing client-side updates.
Using GWT's AutoBean Framework for JSON Serialization and Deserialization
GWT provides an elegant solution for handling JSON data through its AutoBean framework. This framework allows developers to define complex data structures in the form of interfaces, and GWT takes care of the implementation details for JSON serialization and deserialization.
Defining AutoBean Interfaces
Let's start by defining an AutoBean interface for a simple entity, Customer
:
public interface Customer {
String getName();
void setName(String name);
int getAge();
void setAge(int age);
}
In this interface, we define the properties of a Customer
object along with their accessor methods. This provides a clear contract for the structure of the Customer
object.
Creating AutoBean Instances
AutoBean<Customer> customerBean = AutoBeanCodex.decode(factory, Customer.class, json);
Customer customer = customerBean.as();
Here, we use the AutoBeanCodex
to decode a JSON string into an AutoBean
instance and then convert it into a Customer
object. This process is handled by the GWT runtime, making it a seamless operation.
Encoding AutoBean Instances to JSON
AutoBean<Customer> customerBean = factory.create(Customer.class);
customerBean.as().setName("John Doe");
customerBean.as().setAge(30);
String json = AutoBeanCodex.encode(customerBean).getPayload();
In this example, we create an AutoBean
instance for a Customer
object, set its properties, and then encode it into a JSON string using the AutoBeanCodex
. GWT will take care of the serialization process based on the structure defined in the Customer
interface.
Custom JSON Serialization and Deserialization with GWT
While GWT's AutoBean framework provides seamless JSON handling for simple cases, there are scenarios where custom serialization and deserialization may be required for more complex data structures or specific data formats.
Implementing Custom JSON Serialization
public class CustomSerializer implements JsonSerializer<CustomObject> {
@Override
public JSONObject serialize(CustomObject customObject) {
// Custom serialization logic to convert CustomObject to JSONObject
}
}
In this example, we define a custom serializer for a CustomObject
class by implementing the JsonSerializer
interface. Within the serialize
method, we can specify custom logic to convert the CustomObject
into a JSONObject
.
Implementing Custom JSON Deserialization
public class CustomDeserializer implements JsonDeserializer<CustomObject> {
@Override
public CustomObject deserialize(JSONObject json) {
// Custom deserialization logic to convert JSONObject to CustomObject
}
}
Similarly, we can implement a custom deserializer by extending the JsonDeserializer
interface and providing the logic to convert a JSONObject
into a CustomObject
.
Registering Custom Serializers and Deserializers
JsonUtils.registerSerializer(CustomObject.class, new CustomSerializer());
JsonUtils.registerDeserializer(CustomObject.class, new CustomDeserializer());
Once the custom serializer and deserializer are defined, we need to register them with GWT's JsonUtils
to ensure that the custom serialization and deserialization logic is utilized when working with CustomObject
instances.
Key Takeaways
In this comprehensive guide, we have delved into the details of JSON serialization and deserialization in GWT, exploring both the usage of GWT's AutoBean framework and the implementation of custom serialization and deserialization logic.
By leveraging GWT's built-in capabilities for JSON handling and understanding the scenarios where custom solutions may be necessary, developers can effectively manage JSON data within GWT applications, ensuring seamless communication between the client and the server.
For further insights into GWT JSON serialization and deserialization, you can explore the official GWT documentation and the AutoBean API reference.
Remember, mastering JSON serialization and deserialization is pivotal for building robust and efficient GWT applications that excel in modern web development landscapes.