Fixing UnknownHostException: Invalid Hostname for Server
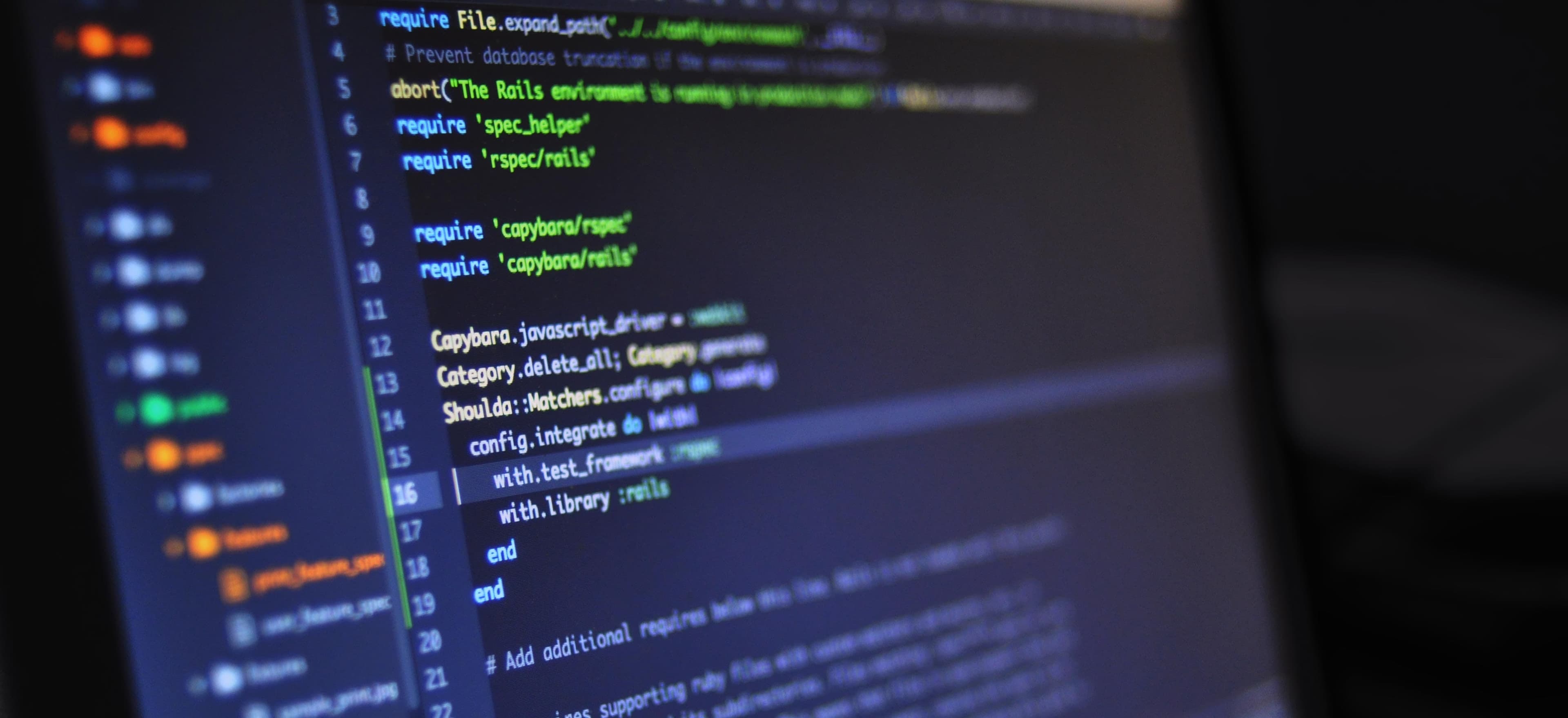
- Published on
Solving UnknownHostException: Invalid Hostname for Server
You're ready to deploy your Java application to a server, only to encounter the dreaded "UnknownHostException: Invalid Hostname" error. Don't worry; this issue is a common stumbling block, and we'll guide you through the diagnosis and solution in this article.
Understanding UnknownHostException
The UnknownHostException arises when Java's networking API cannot resolve a given hostname to an IP address. It is often caused by a typo, an incorrect server address, or a DNS misconfiguration.
Diagnosing the Issue
Before we jump into the solution, let's cover the diagnosis process. First, ensure that the server hostname or IP address is correct and accessible from your environment. Check for typos and verify that the server is reachable.
The Solution
1. Check the Hostname
Verify the server hostname or IP address you are trying to connect to. Ensure that there are no typos in the URL or IP address. Even a small mistake can lead to an UnknownHostException.
2. DNS Configuration
If you are using a hostname to connect to the server, ensure that the DNS is configured correctly. You can check the DNS resolution using the nslookup
command on Unix-based systems or ipconfig /displaydns
on Windows.
3. Proxy Settings
If your environment requires a proxy to connect to the server, ensure that the proxy settings are correctly configured. An incorrectly configured proxy can lead to hostname resolution issues.
4. Code Check
Review the code where the hostname is being used. Ensure that the hostname is being provided correctly to the networking API.
Here's an example of how to create a connection using HttpURLConnection
with the correct handling of UnknownHostException
:
try {
URL url = new URL("https://www.example.com");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// Perform operations with the connection
} catch (UnknownHostException e) {
// Handle the UnknownHostException
}
In this code snippet, when creating a connection, we handle the UnknownHostException
explicitly. It's essential to handle this exception gracefully in your code.
5. Network Configuration
Check the network configuration of the environment where the Java application is running. Ensure that there are no restrictions or firewalls blocking the outgoing connections to the server.
Testing the Solution
After implementing the above steps, test the application to ensure that the UnknownHostException is resolved. If the issue persists, double-check each step and consider involving your network or system administrators to review the network configuration and DNS settings.
Remember, solving the UnknownHostException requires attention to detail and a systematic approach to rule out potential causes.
Bringing It All Together
Encountering an UnknownHostException can be frustrating, but with a structured approach to troubleshooting and the steps outlined in this article, you can effectively address this issue. By verifying the hostname, reviewing DNS configurations, checking proxy settings, reviewing code, and ensuring network configurations, you can mitigate the UnknownHostException and successfully deploy your Java application to the server.
With these solutions in place, you are well-equipped to tackle the "UnknownHostException: Invalid Hostname" error and ensure smooth connectivity for your Java application.