Optimizing Retry Strategies in Apache Camel
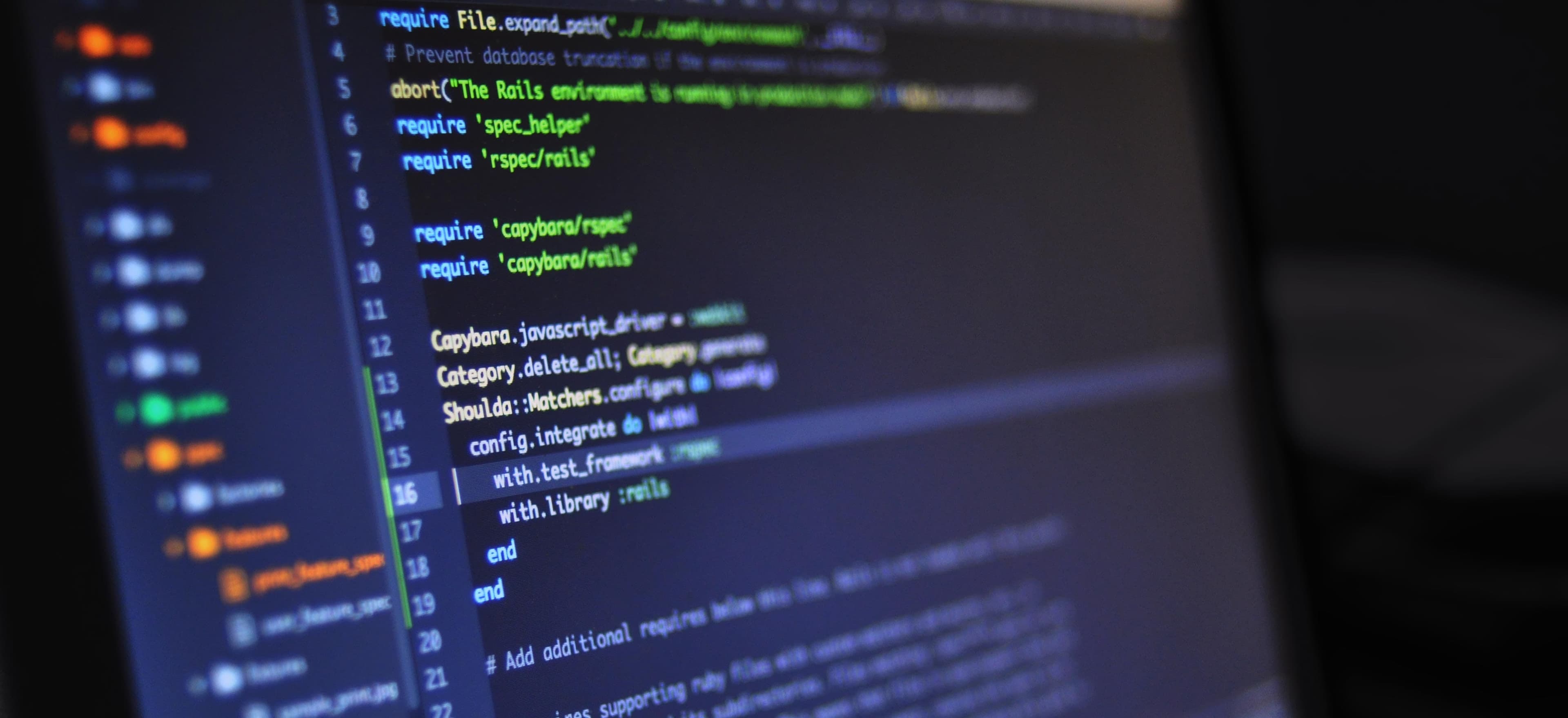
- Published on
Optimizing Retry Strategies in Apache Camel
When working with distributed systems, communication between different services is crucial. However, failures are inevitable, and handling them gracefully is a key aspect of building resilient systems. Apache Camel, an open-source integration framework, provides various options for implementing retry strategies to handle failures.
In this blog post, we will explore different retry strategies in Apache Camel and focus on optimizing these strategies to build robust and fault-tolerant applications.
Understanding Retry Strategies in Apache Camel
Retry strategies in Apache Camel allow developers to define the behavior of retrying a specific operation in case of failure. This can be particularly useful when calling external services, interacting with databases, or processing messages from a queue.
Apache Camel provides a rich set of options for defining retry strategies, including:
-
Simple Retry: A straightforward retry mechanism with options to specify the maximum number of attempts and the delay between each attempt.
-
Exponential Backoff: A more sophisticated strategy that increases the delay between each attempt exponentially, reducing the load on the failing service.
-
Custom Retry Policy: Developers can implement custom retry policies to have fine-grained control over the retry behavior based on the specific requirements of their application.
Optimizing Retry Strategies for Better Resilience
While implementing retry strategies is important, it is equally crucial to optimize them for better resilience and performance. Let's dive into some optimization techniques for retry strategies in Apache Camel.
1. Backoff Strategies
Exponential backoff strategies can be particularly effective in reducing the load on the failing service during periods of high contention or transient failures. By gradually increasing the delay between retry attempts, these strategies can prevent overwhelming the service and improve overall system stability.
Here's an example of implementing exponential backoff in Apache Camel:
onException(Exception.class)
.maximumRedeliveries(3)
.redeliveryDelay(1000, 2000, 8000)
.end();
In this example, the redeliveryDelay
method specifies the initial delay and subsequent incremental delays for each retry attempt. This approach helps in optimizing the retry strategy by introducing a gradual increase in the retry interval.
2. Circuit Breaker Pattern
Integrating a circuit breaker pattern with retry strategies can further enhance system resilience. The circuit breaker can be used to temporarily stop retrying an operation that is likely to fail, allowing the system to handle the failure gracefully without overwhelming the failing service. This can prevent cascading failures and improve overall system stability.
Apache Camel provides support for the circuit breaker pattern through the Hystrix component. By combining Hystrix with retry strategies, developers can build robust and resilient systems capable of gracefully handling failures.
3. Dead Letter Queue
In scenarios where retries are unsuccessful after a certain number of attempts, it is essential to have a mechanism for handling these "dead" messages or operations. Apache Camel offers the Dead Letter Queue (DLQ) pattern, where failed messages are moved to a separate queue for manual or automated handling. By implementing DLQ, developers can prevent message loss and gain visibility into failed operations for analysis and resolution.
Here's an example of configuring a Dead Letter Queue in Apache Camel:
errorHandler(deadLetterChannel("activemq:queue:DeadLetterQueue")
.maximumRedeliveries(5)
.redeliveryDelay(5000)
.onRedelivery(exchange -> {
// Perform logging or additional actions on redelivery
});
By incorporating DLQ with retry strategies, developers can optimize the error handling process and ensure that failed messages are handled appropriately, improving system reliability.
4. Dynamic Configuration
To optimize retry strategies, it is beneficial to have the ability to dynamically configure retry parameters based on real-time conditions or external factors. Apache Camel provides the flexibility to dynamically adjust retry settings using languages like Simple, Groovy, or even custom beans.
By leveraging dynamic configuration, developers can adapt retry behavior based on changing conditions, such as service availability, response times, and error patterns, thereby improving the effectiveness of retry strategies.
The Bottom Line
Optimizing retry strategies in Apache Camel is essential for building resilient and fault-tolerant applications in distributed environments. By implementing backoff strategies, integrating the circuit breaker pattern, utilizing Dead Letter Queues, and enabling dynamic configuration, developers can enhance the effectiveness of retry mechanisms and improve system stability.
As you delve into optimizing retry strategies in Apache Camel, keep in mind that the goal is to strike a balance between resilience and performance, ensuring that your applications can gracefully handle failures while maintaining efficient operation.
Explore the Apache Camel documentation for in-depth information on retry strategies and other powerful features offered by the framework. Additionally, stay updated with the latest best practices and patterns for building robust, fault-tolerant systems in distributed environments.
Remember, optimizing retry strategies is a continuous process, and it's essential to monitor and fine-tune these strategies based on real-world feedback and evolving system requirements. With a well-optimized retry mechanism in place, your applications can effectively navigate failure scenarios and deliver a seamless experience to users.
Start refining your retry strategies today to elevate the resilience of your Apache Camel-based applications!