Handling Asynchronous Tasks with ListenableFuture
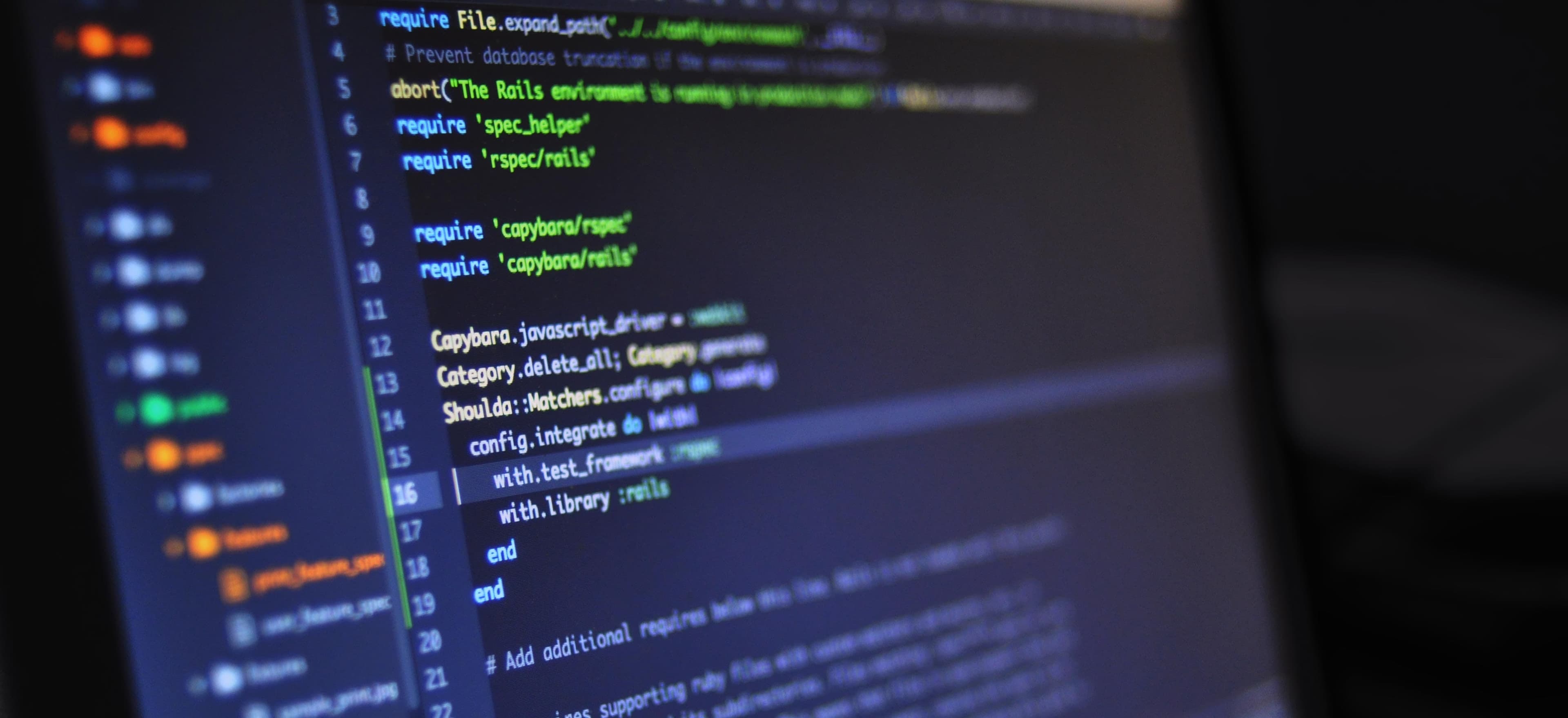
- Published on
When developing Java applications, it's often necessary to handle asynchronous tasks to improve performance and user experience. Java provides the ListenableFuture interface, which offers a way to represent a future result of an asynchronous computation. In this article, we'll explore how ListenableFuture works and how to use it effectively in your Java applications.
What is ListenableFuture?
ListenableFuture is part of the Guava library, which provides fundamental utilities for Java. It's similar to Java's built-in Future interface, but it adds the ability to attach listeners to the future's result. This makes it a powerful tool for dealing with asynchronous tasks and handling their results.
ListenableFuture is part of the com.google.common.util.concurrent package. To use ListenableFuture, you need to include the Guava library in your project. You can do this by adding the following dependency to your Maven project:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version>
</dependency>
Creating ListenableFuture
To create a ListenableFuture, you can use the ListeningExecutorService, which is an extension of the standard Java ExecutorService. The ListeningExecutorService allows you to submit tasks that return ListenableFuture instances.
Here's an example of creating a ListenableFuture using the MoreExecutors.directExecutor():
import com.google.common.util.concurrent.*;
import java.util.concurrent.Callable;
public class ListenableFutureExample {
public static void main(String[] args) {
ListeningExecutorService service = MoreExecutors.listeningDecorator(Executors.newFixedThreadPool(10));
ListenableFuture<String> future = service.submit(new Callable<String>() {
public String call() {
// Perform some asynchronous computation
return "Hello, ListenableFuture!";
}
});
Futures.addCallback(future, new FutureCallback<String>() {
public void onSuccess(String result) {
System.out.println("Success: " + result);
}
public void onFailure(Throwable t) {
System.out.println("Failure: " + t.getMessage());
}
}, MoreExecutors.directExecutor());
}
}
In this example, we create a ListeningExecutorService using MoreExecutors.listeningDecorator() to convert a standard ExecutorService into a ListeningExecutorService. We then submit a Callable task to the service, which returns a ListenableFuture. We use Futures.addCallback() to attach success and failure callbacks to the future.
Handling ListenableFuture Results
One of the key features of ListenableFuture is the ability to attach listeners to handle the result of the asynchronous computation. This allows you to perform actions as soon as the computation is complete, whether it's a success or a failure.
In the previous example, we used Futures.addCallback() to add success and failure callbacks to the ListenableFuture. This method takes three arguments:
- The ListenableFuture instance
- A FutureCallback for handling the result on success or failure
- An executor, which specifies where the callback should be executed
Futures.addCallback(future, new FutureCallback<String>() {
public void onSuccess(String result) {
System.out.println("Success: " + result);
}
public void onFailure(Throwable t) {
System.out.println("Failure: " + t.getMessage());
}
}, MoreExecutors.directExecutor());
In this example, the onSuccess() method is called when the computation is successful, and onFailure() is called when there's an exception or failure. You can perform any necessary actions, such as updating the UI or handling errors, within these callback methods.
Chaining ListenableFutures
ListenableFutures can also be chained together to create a pipeline of asynchronous computations. This allows you to express complex concurrency patterns in a clear and manageable way.
Here's an example of chaining two ListenableFutures using the transform() method:
ListenableFuture<Integer> future1 = service.submit(new Callable<Integer>() {
public Integer call() {
// Perform some asynchronous computation
return 42;
}
});
Function<Integer, String> intToString = new Function<Integer, String>() {
public String apply(Integer input) {
return "The answer is " + input;
}
};
ListenableFuture<String> future2 = Futures.transform(future1, intToString, MoreExecutors.directExecutor());
In this example, we submit a Callable task that returns an Integer to the service, creating future1. We then define a Function that transforms an Integer to a String, and use Futures.transform() to create future2, which represents the result of applying the transformation to future1.
Chaining ListenableFutures allows you to build complex asynchronous workflows while keeping the code organized and easy to understand.
The Closing Argument
ListenableFuture is a powerful tool for handling asynchronous tasks in Java, providing a way to represent the result of an asynchronous computation and attach listeners to handle success or failure. By using ListenableFuture, you can write more concise and readable code for managing asynchronous operations and improving the responsiveness of your applications.
In this article, we've covered the basics of ListenableFuture, including creating and handling ListenableFutures, as well as chaining them together. By incorporating ListenableFuture into your Java projects, you can effectively manage asynchronous tasks and provide a better user experience.
By mastering ListenableFuture, you can take your Java applications to the next level of performance and responsiveness.
For more in-depth information on ListenableFuture, you can refer to the official Guava documentation: Guava ListenableFuture Documentation
Start integrating ListenableFuture into your Java projects and unlock the potential of asynchronous task handling!