Optimizing Performance for Headless Ecommerce
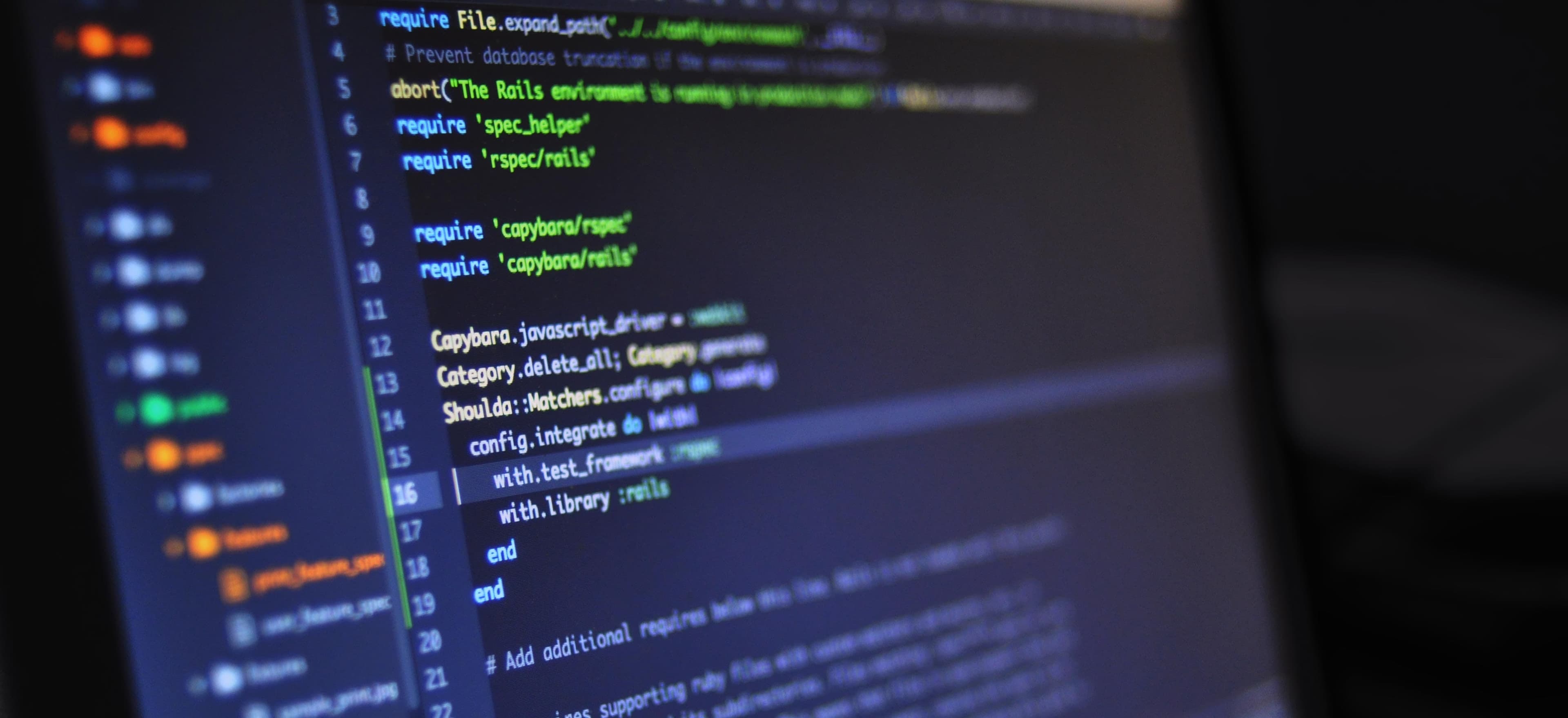
- Published on
Maximizing Performance in Headless Ecommerce with Java
In the competitive world of ecommerce, providing a seamless and lightning-fast user experience is critical. One way to achieve this is by leveraging headless architecture, where the frontend and backend are decoupled, allowing for greater flexibility and performance optimization. In this article, we'll explore how Java can be utilized to optimize the performance of headless ecommerce platforms.
Understanding the Importance of Performance Optimization
Performance optimization is crucial for ecommerce platforms, as it directly impacts user satisfaction, conversion rates, and search engine rankings. Slow loading times can lead to high bounce rates and abandoned carts, negatively impacting revenue.
Leveraging Java for Backend Optimization
Java, known for its performance, scalability, and robustness, is a popular choice for backend development in headless ecommerce. Its multithreading capabilities, memory management, and vast ecosystem make it well-suited for handling heavy loads and complex business logic.
Multithreading for Concurrent Processing
Java's support for multithreading allows for concurrent processing of tasks, enabling the backend to handle multiple requests simultaneously. This is particularly beneficial for ecommerce platforms experiencing high traffic and concurrent user interactions. Here's an example of how multithreading can be utilized in Java:
ExecutorService executor = Executors.newFixedThreadPool(10);
for (int i = 0; i < 10; i++) {
executor.submit(() -> {
// Perform task asynchronously
});
}
executor.shutdown();
In this example, an ExecutorService
is created to manage a pool of threads, allowing for efficient execution of tasks in parallel.
Memory Management for Efficiency
Java's automatic memory management through garbage collection helps optimize resource utilization in the backend, preventing memory leaks and excessive memory consumption. Efficient memory management is crucial for maintaining consistent performance, especially during high load periods.
Integration with Caching Solutions
Utilizing caching solutions such as Redis or Memcached in Java applications can significantly improve performance by storing frequently accessed data in memory. This reduces the need to fetch data from the database repeatedly, leading to faster response times. Here's an example of integrating Redis caching in Java using the Jedis library:
Jedis jedis = new Jedis("localhost");
jedis.set("key", "value");
String cachedValue = jedis.get("key");
By leveraging caching, frequent database queries can be minimized, resulting in improved responsiveness of the ecommerce platform.
Frontend Performance Optimization with Java
While backend optimization is vital, frontend performance is equally significant in a headless architecture. Java can be utilized in conjunction with modern frontend technologies to enhance the user experience.
Server-Side Rendering (SSR) with Java
Server-side rendering, where the server pre-renders the initial HTML content, can significantly improve perceived page load times and search engine optimization. Java frameworks such as Spring Boot can be used to implement SSR by rendering the initial view on the server before sending it to the client.
Optimizing JavaScript Bundling and Minification
Java build tools such as Maven or Gradle can be employed to automate the bundling and minification of JavaScript files, reducing their size and improving frontend load times. By integrating tools like Webpack with Java build processes, developers can ensure that frontend assets are optimized for performance.
Implementing Lazy Loading for Images and Assets
Lazy loading of images and other assets can be achieved in the frontend using JavaScript libraries such as LazyLoad. When combined with Java-powered backend APIs that support partial content retrieval, lazy loading contributes to faster initial page loads and reduced bandwidth consumption.
Utilizing Performance Monitoring and Optimization Tools
In the quest for performance optimization, leveraging monitoring and profiling tools is essential to identify bottlenecks and fine-tune the system.
Application Performance Monitoring (APM) Tools
Tools like New Relic, AppDynamics, or Java-specific APM solutions provide in-depth insights into application performance, including response times, database queries, and server resource utilization. These tools enable proactive identification and resolution of performance issues, ensuring an optimal user experience.
Profiling and Tuning with Java Mission Control
Java Mission Control, a part of the Java Development Kit (JDK), offers powerful profiling and diagnostic capabilities to analyze the performance of Java applications. By identifying hotspots, memory leaks, and excessive resource consumption, developers can make informed optimizations to enhance the overall system performance.
Closing Remarks
In the realm of headless ecommerce, performance optimization is a continuous journey driven by evolving technologies and user expectations. By harnessing the power of Java for backend efficiency, leveraging modern frontend techniques, and embracing performance monitoring tools, ecommerce platforms can deliver a blazing-fast and seamless shopping experience. With Java's robust capabilities, optimizing the performance of headless ecommerce becomes an achievable goal, propelling businesses to new heights in the digital marketplace.
As ecommerce continues to evolve, staying ahead in performance optimization will be critical for businesses. By understanding the significance of Java in enhancing performance, developers and organizations can pave the way for a competitive edge in the ever-growing ecommerce landscape.
In conclusion, optimizing performance for headless ecommerce with Java is not just a good practice, it is a necessity in today's fast-paced digital world. By leveraging the strengths of Java, businesses can ensure that their ecommerce platforms are responsive, scalable, and capable of delivering exceptional user experiences.