Managing Design Pressure: A Guide for Engineering Teams
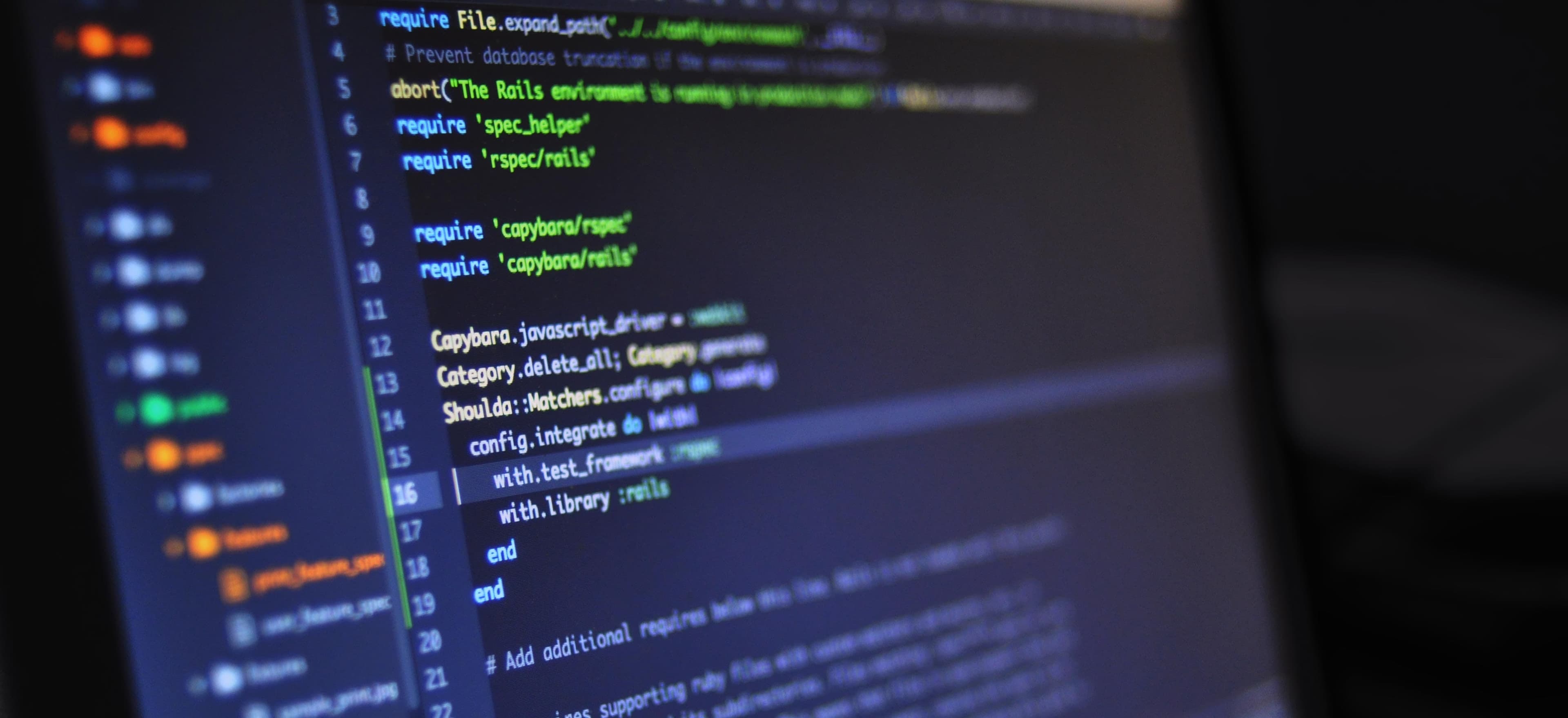
- Published on
Understanding Design Pressure in Java
In the world of Java development, understanding and managing design pressure is crucial for engineering teams. Design pressure refers to the tension between the existing codebase and the need to implement new features or make changes. It encompasses the challenges of maintaining code quality, managing technical debt, and ensuring the scalability and maintainability of the software.
The Impact of Design Pressure
When design pressure is not effectively managed, it can lead to a variety of issues such as:
- Technical Debt: Accumulation of technical debt due to shortcuts taken to meet deadlines or lack of refactoring.
- Decreased Productivity: Developers spend more time navigating and understanding complex code, reducing their productivity.
- Bugs and Errors: Poorly designed or tightly coupled code can lead to an increase in bugs and errors.
- Scalability Challenges: Code that's not designed for scalability can hinder the growth and performance of the application.
- Team Morale: Constantly working under design pressure can lead to low team morale and burnout.
Effective Techniques for Managing Design Pressure in Java
1. Use of Design Patterns
When building new features or making changes, leveraging design patterns can significantly reduce design pressure. Design patterns provide proven solutions to common problems in software design and promote code reusability, flexibility, and maintainability.
Example of the Strategy Pattern in Java:
public interface PaymentMethod {
void pay(double amount);
}
public class CreditCardPayment implements PaymentMethod {
public void pay(double amount) {
// Implement credit card payment logic
}
}
public class PayPalPayment implements PaymentMethod {
public void pay(double amount) {
// Implement PayPal payment logic
}
}
public class PaymentProcessor {
private PaymentMethod paymentMethod;
public void setPaymentMethod(PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
public void processPayment(double amount) {
// Perform common payment processing logic
paymentMethod.pay(amount);
}
}
In this example, the Strategy Pattern facilitates the implementation of different payment methods without altering the core logic of the payment processor.
2. Refactoring and Code Reviews
Regular refactoring of codebase and conducting thorough code reviews are essential for managing design pressure. Refactoring helps in eliminating duplication, reducing complexity, and improving the overall design of the code, while code reviews ensure that the changes align with the architecture and coding standards.
Example of Refactoring in Java:
// Before refactoring
public double calculateTotalPrice(List<Item> items) {
double totalPrice = 0;
for (Item item : items) {
totalPrice += item.getPrice();
}
return totalPrice;
}
// After refactoring
public double calculateTotalPrice(List<Item> items) {
return items.stream().mapToDouble(Item::getPrice).sum();
}
The refactored code uses Java Streams to achieve the same functionality with reduced boilerplate and improved readability.
3. Test-Driven Development (TDD)
Adopting Test-Driven Development (TDD) can help in managing design pressure by ensuring that new features or changes are implemented with comprehensive test coverage. Writing tests before writing the actual code encourages thoughtful design and loose coupling, leading to a more maintainable codebase.
Example of TDD in Java:
public class StringUtil {
public static String reverse(String input) {
return new StringBuilder(input).reverse().toString();
}
}
In this example, the implementation of the reverse
method is preceded by the creation of a test that specifies the expected behavior. This approach guides the design and implementation process, reducing the chances of tightly coupled or poorly designed code.
4. Modularization and Encapsulation
Breaking down the codebase into modular components and encapsulating the implementation details within those modules can alleviate design pressure. Modularization promotes code reusability, eases maintenance, and enables developers to work on specific parts of the application without affecting others.
Example of Modularization and Encapsulation in Java:
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(long userId) {
return userRepository.findById(userId);
}
public void updateUser(User user) {
userRepository.save(user);
}
}
In this example, the UserService
class encapsulates the user-related operations, with the UserRepository
dependency abstracted away. This modular design allows for independent development and testing of the user-related functionality.
My Closing Thoughts on the Matter
Managing design pressure in Java development is a continuous effort that requires a proactive approach and consistent adoption of best practices. By incorporating design patterns, embracing refactoring and code reviews, practicing TDD, and promoting modularization and encapsulation, engineering teams can effectively mitigate the impact of design pressure on the codebase. Ultimately, a well-managed design pressure leads to a more maintainable, scalable, and robust software system.
To further explore the significance of design patterns in Java, consider the timeless principles presented in the book "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides.
In addition, for a comprehensive understanding of Test-Driven Development in Java, you may find value in Kent Beck's seminal work, "Test Driven Development: By Example".
Remember, managing design pressure not only benefits the codebase but also contributes to the overall productivity and satisfaction of the engineering team.