Data Retrieval Issue with Couchbase in Java EE Application
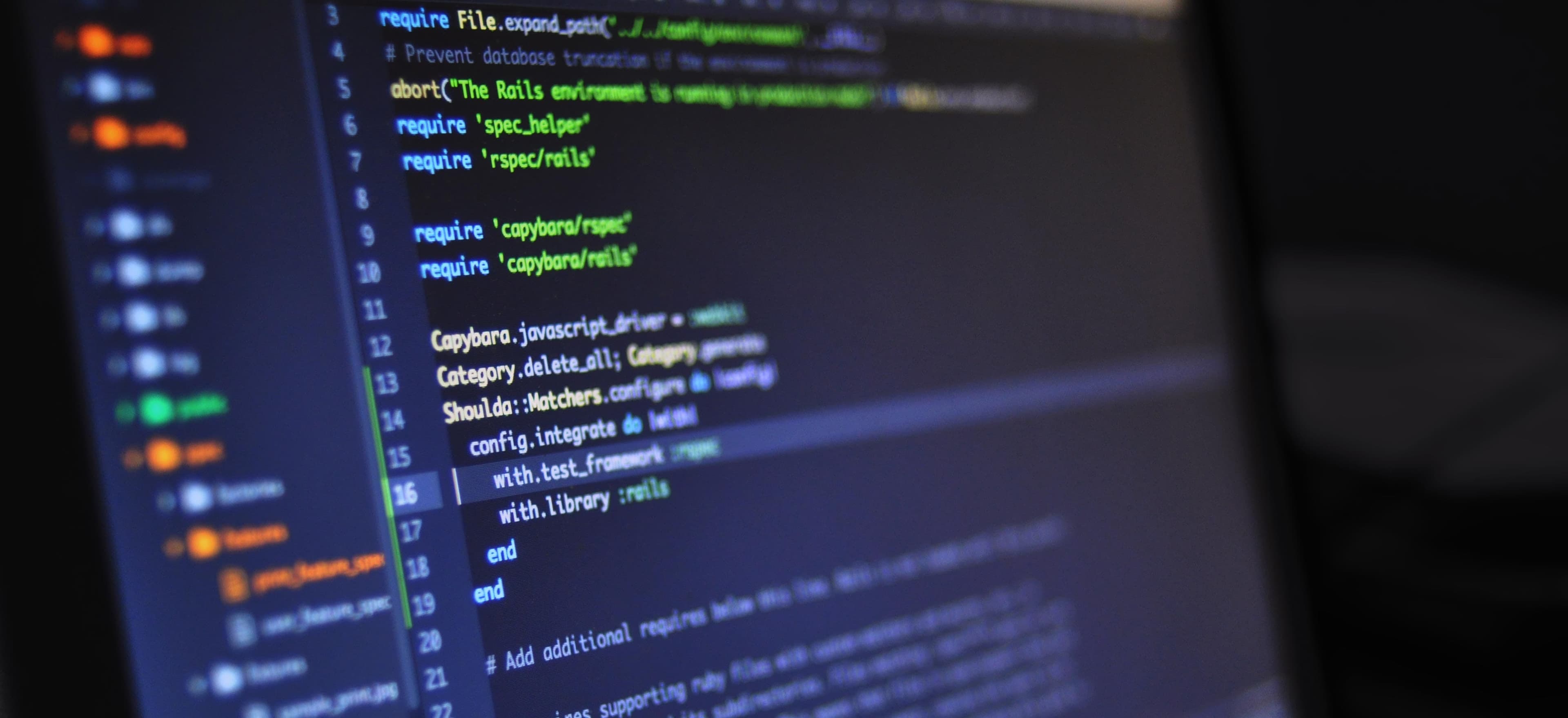
- Published on
Troubleshooting Data Retrieval Issue with Couchbase in Java EE Application
Couchbase is a popular NoSQL database that provides a flexible and scalable solution for storing and retrieving data. However, as with any technology, issues can arise, and troubleshooting becomes essential. In this article, we'll address a data retrieval issue with Couchbase in a Java EE application and explore potential solutions.
Understanding the Problem
Suppose you have a Java EE application that uses Couchbase as its primary data store. When attempting to retrieve data from Couchbase, you encounter unexpected results or, worse, no results at all. This can be a frustrating experience, but understanding the potential causes of this issue is the first step towards finding a solution.
Potential Causes
Several factors could contribute to a data retrieval issue when using Couchbase in a Java EE application. Some of the common causes include:
-
Incorrect Configuration: The configuration parameters for connecting to the Couchbase cluster might be incorrect, leading to connection failures.
-
Document Key Mismatch: The document key used to retrieve data may not match the actual key stored in Couchbase, resulting in no data being returned.
-
Serialization/Deserialization Errors: Issues with serializing or deserializing data between the Java application and Couchbase can lead to unexpected results.
-
Cluster/Node Unavailability: If a Couchbase cluster or node is unavailable or experiencing issues, data retrieval operations may fail.
Investigating the Issue
To address the data retrieval issue, let's start by examining the relevant Java code responsible for interacting with Couchbase. We'll look at an example method that retrieves a document from Couchbase using the Java SDK.
// Example code for retrieving a document from Couchbase
public class CouchbaseRepository {
private Cluster cluster;
private Bucket bucket;
// Constructor and initialization omitted for brevity
public Object retrieveDocument(String documentId) {
return bucket.defaultCollection().get(documentId);
}
}
In the above code, the retrieveDocument
method is responsible for retrieving a document from Couchbase based on its documentId
. However, if this method is not returning the expected data, we need to delve deeper into the potential causes and solutions.
Assessing the Code
Let's assess the mentioned code snippet and discuss why it might lead to a data retrieval issue:
-
Inefficient Error Handling: The method lacks proper error handling, which can result in exceptions being swallowed or ignored, making it challenging to diagnose the root cause of the issue.
-
Use of Default Collection: The code uses the default collection for the retrieval operation. While this may work for simple scenarios, it may not be suitable for more complex setups or specific use cases.
Solution: Enhanced Data Retrieval Method
To address the potential issues identified, let's enhance the retrieveDocument
method to incorporate better error handling and optimize the data retrieval process. The following updated code snippet demonstrates the improvements:
public class CouchbaseRepository {
private Cluster cluster;
private Bucket bucket;
// Constructor and initialization omitted for brevity
public Object retrieveDocument(String documentId) {
try {
return bucket.defaultCollection().get(documentId);
} catch (DocumentNotFoundException e) {
// Handle document not found error
return null;
} catch (CouchbaseException e) {
// Handle generic Couchbase exceptions
e.printStackTrace();
// Perform additional error handling or logging
return null;
}
}
public Object retrieveDocumentFromScopeAndCollection(String scopeName, String collectionName, String documentId) {
// Access a specific scope and collection for retrieval
return cluster.scope(scopeName).collection(collectionName).get(documentId);
}
}
In this enhanced version, we've introduced proper error handling using try-catch blocks to capture specific exceptions such as DocumentNotFoundException
and generic CouchbaseException
. Additionally, we've included a new method retrieveDocumentFromScopeAndCollection
to enable retrieval from a specific scope and collection, providing more flexibility.
Additional Considerations
While the code improvements enhance the data retrieval process, it's essential to consider other factors that might impact Couchbase data retrieval in a Java EE application:
-
Connection Configuration: Verify that the Couchbase connection parameters, such as cluster addresses, authentication credentials, and bucket details, are accurately configured in the Java EE application. Any discrepancies can lead to connection failures.
-
Data Consistency: Ensure that the document keys used for retrieval are consistent with the ones stored in Couchbase. Inconsistent key naming can result in failed retrieval attempts.
-
Serialization/Deserialization: Check that the Java objects being stored and retrieved from Couchbase are correctly serialized and deserialized to maintain data integrity.
Closing the Chapter
Troubleshooting data retrieval issues in a Java EE application using Couchbase requires a systematic approach that involves identifying potential causes, assessing the relevant code, and implementing solutions to enhance the data retrieval process. By incorporating enhanced error handling and optimizing data retrieval methods, you can mitigate common issues and ensure a more robust integration with Couchbase.
Addressing data retrieval issues not only improves the application's reliability but also enhances the overall user experience by ensuring seamless access to data stored in Couchbase.
In conclusion, a combination of meticulous code review, error handling improvements, and adherence to best practices can significantly contribute to resolving data retrieval issues with Couchbase in a Java EE application.
By addressing these fundamental aspects, you can effectively troubleshoot and resolve data retrieval issues with Couchbase, ensuring a robust and reliable data storage and retrieval mechanism within your Java EE application.
For further reference and advanced troubleshooting, you might find the official Couchbase documentation extremely helpful in understanding the nuances of interacting with Couchbase in a Java environment.
Checkout our other articles