Managing Concurrent Delete and Update Operations
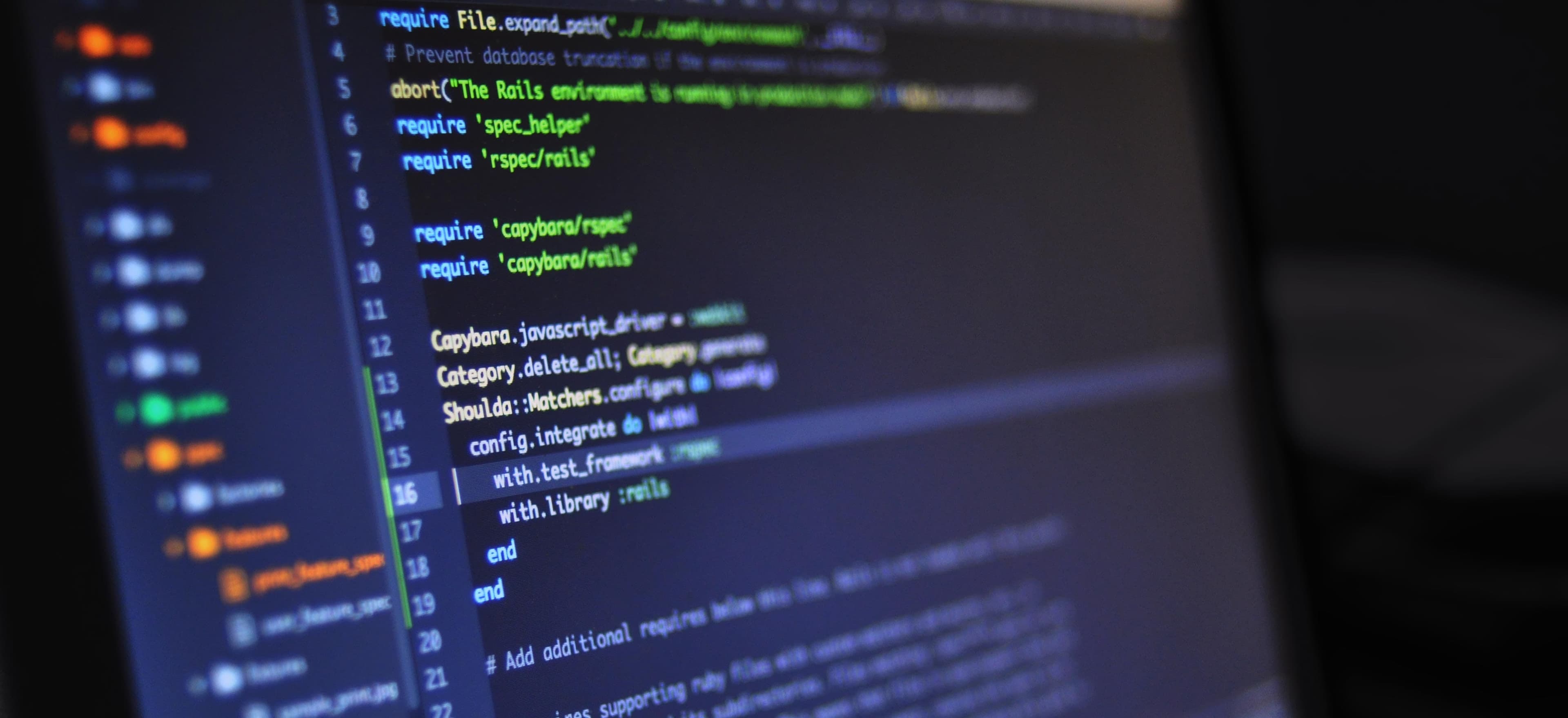
- Published on
Optimizing Java Applications for Concurrent Delete and Update Operations
In a multi-threaded Java application, concurrent delete and update operations are common occurrences that can lead to race conditions, data inconsistencies, and performance issues if not managed properly. In this article, we will discuss strategies for optimizing Java applications to handle concurrent delete and update operations efficiently and safely.
Understanding the Problem
Concurrent delete and update operations occur when multiple threads attempt to modify the same data simultaneously. In the context of a Java application, this can happen when multiple threads access and modify shared data structures such as collections, databases, or files.
The potential issues that arise from concurrent delete and update operations include:
-
Race Conditions: When multiple threads access and modify the same data without proper synchronization, race conditions can occur, leading to unexpected behavior and data corruption.
-
Data Inconsistencies: If one thread deletes or updates data while another thread is still reading or referencing it, inconsistencies can occur, resulting in incorrect or unpredictable behavior.
-
Performance Bottlenecks: Inefficient handling of concurrent operations can lead to performance bottlenecks and reduced application responsiveness.
To address these issues, it is essential to implement strategies that ensure safe and efficient concurrent delete and update operations in Java applications.
Synchronization and Locking
One of the fundamental ways to address concurrent delete and update operations is through synchronization and locking mechanisms provided by Java, such as using synchronized
blocks or using the java.util.concurrent.locks
package.
Synchronized Blocks
public class SynchronizedExample {
private List<String> dataList = new ArrayList<>();
public synchronized void performUpdate(String data) {
// Perform update operation
dataList.add(data);
}
public synchronized void performDelete(String data) {
// Perform delete operation
dataList.remove(data);
}
}
In the above example, the performUpdate
and performDelete
methods are synchronized, ensuring that only one thread can execute these methods at a time, preventing concurrent modification issues. However, this approach may lead to contention and potential performance degradation if the synchronized block is too broad.
Reentrant Locks
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class LockExample {
private List<String> dataList = new ArrayList<>();
private Lock lock = new ReentrantLock();
public void performUpdate(String data) {
lock.lock();
try {
// Perform update operation
dataList.add(data);
} finally {
lock.unlock();
}
}
public void performDelete(String data) {
lock.lock();
try {
// Perform delete operation
dataList.remove(data);
} finally {
lock.unlock();
}
}
}
In the LockExample
, the ReentrantLock
is used to provide a finer-grained control over locking, potentially reducing contention and improving performance compared to synchronized
blocks.
Using Concurrent Data Structures
Java provides concurrent versions of some data structures, such as ConcurrentHashMap
, CopyOnWriteArrayList
, and ConcurrentSkipListMap
, which are designed to handle concurrent operations without external synchronization.
ConcurrentHashMap
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentHashMapExample {
private Map<String, String> dataMap = new ConcurrentHashMap<>();
public void performUpdate(String key, String value) {
// Perform update operation
dataMap.put(key, value);
}
public void performDelete(String key) {
// Perform delete operation
dataMap.remove(key);
}
}
In the ConcurrentHashMapExample
, the ConcurrentHashMap
allows multiple threads to perform updates and deletes concurrently without the need for explicit synchronization, improving performance and scalability.
Optimistic Locking
Optimistic locking is a concurrency control technique that allows multiple threads to perform operations concurrently, assuming that conflicts are rare. When a conflicting update or delete operation is detected, the optimistic locking mechanism resolves the conflict.
Java Persistence API (JPA) Optimistic Locking
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Version
private int version;
// Other fields and methods
}
In the above example, the @Version
annotation in a JPA entity enables optimistic locking, where the version attribute is used to detect conflicting update operations.
Handling Failures and Retries
In a concurrent environment, delete and update operations can fail due to conflicts or other factors. It's essential to handle these failures gracefully and implement retry mechanisms when appropriate.
Exponential Backoff Retry
public class RetryExample {
private static final int MAX_RETRIES = 3;
private static final long BACKOFF_INTERVAL = 1000; // in milliseconds
public void performUpdateWithRetry(String data) {
int numRetries = 0;
while (numRetries < MAX_RETRIES) {
try {
// Perform update operation
// If successful, break from the loop
break;
} catch (ConcurrentModificationException | TimeoutException e) {
// Handle the exception and back off
Thread.sleep((long) (Math.pow(2, numRetries) * BACKOFF_INTERVAL));
numRetries++;
}
}
}
}
In the RetryExample
, an exponential backoff retry strategy is used to handle concurrent update operations that may encounter conflicts or timeouts.
Final Considerations
Managing concurrent delete and update operations in a Java application requires careful consideration of synchronization, locking, concurrent data structures, optimistic locking, and handling failures. By employing these strategies, developers can ensure the safety, consistency, and performance of their applications in multi-threaded environments.
In summary, optimizing Java applications for concurrent delete and update operations involves:
- Using synchronization and locking mechanisms
- Leveraging concurrent data structures
- Implementing optimistic locking strategies
- Handling failures and retries effectively
By applying these best practices, Java developers can build robust and efficient multi-threaded applications that handle concurrent delete and update operations with ease.
For further reading on concurrent programming in Java, consider referring to the Java Concurrency in Practice book, which offers in-depth insights into concurrent programming and best practices. Additionally, the official Java documentation on concurrency provides detailed information on managing concurrent operations in Java.
Remember, by understanding the nuances of concurrent delete and update operations and implementing effective strategies, Java developers can optimize their applications for robustness and scalability in multi-threaded environments.