Troubleshooting Common Issues with Spring LDAP 2.0.0
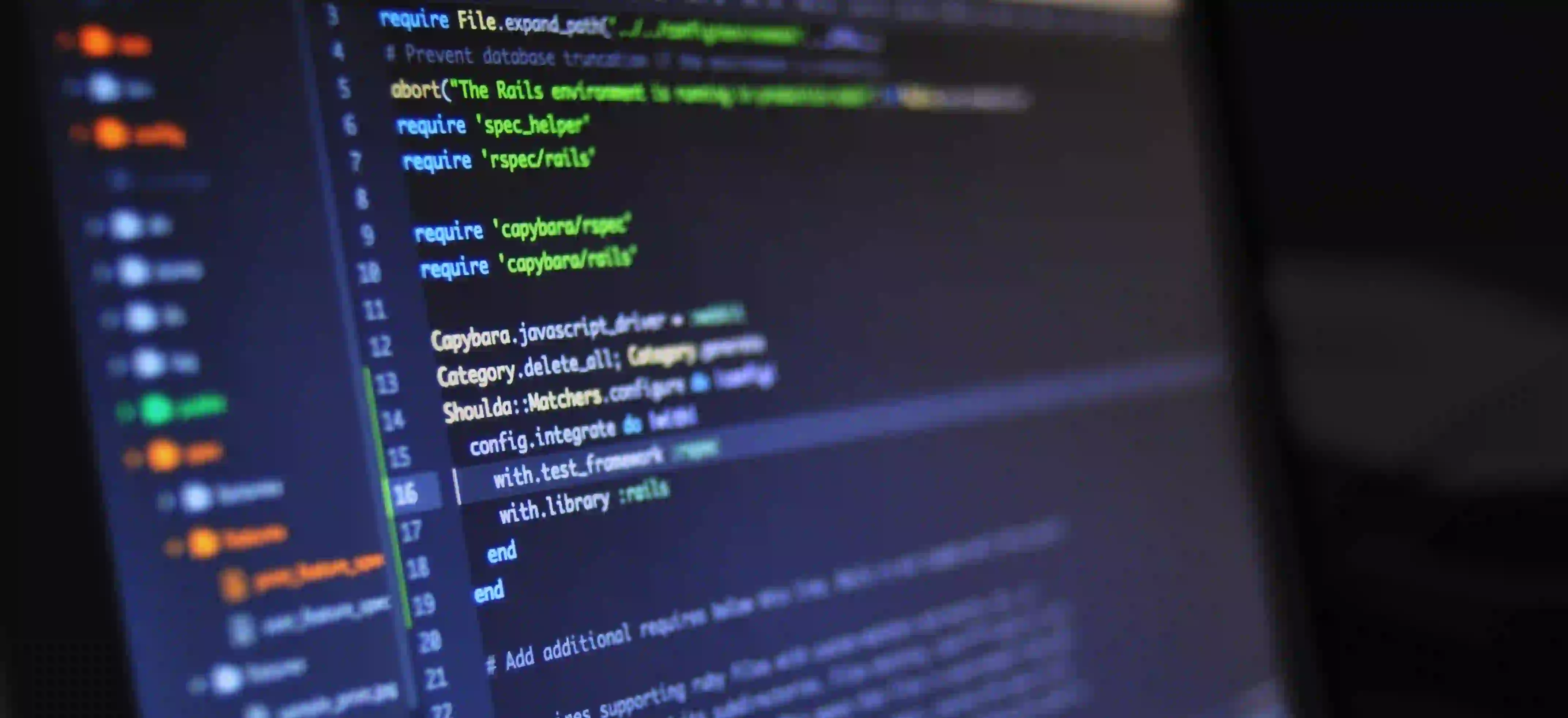
Troubleshooting Common Issues with Spring LDAP 2.0.0
Spring LDAP is a powerful tool for integrating LDAP (Lightweight Directory Access Protocol) functionality into Java applications. However, as with any technology, users may encounter issues when working with Spring LDAP 2.0.0. In this post, we will explore some common problems that developers may face when using Spring LDAP 2.0.0 and discuss how to troubleshoot and resolve these issues.
Issue 1: Connection Problems
One of the most common issues when working with Spring LDAP is connection problems. This can manifest as connection timeouts, connection refused errors, or simply being unable to establish a connection to the LDAP server.
Troubleshooting Steps
To troubleshoot connection problems with Spring LDAP, first, ensure that the LDAP server is running and accessible from the application environment. Check the server address, port, and any firewall settings that may be blocking the connection.
Next, verify the configuration of the LDAP context source in the Spring configuration. Ensure that the context source properties such as URLs, base, username, and password are correctly set.
@Bean
public LdapContextSource contextSource() {
LdapContextSource contextSource = new LdapContextSource();
contextSource.setUrl("ldap://ldap.example.com:389");
contextSource.setBase("dc=example,dc=com");
contextSource.setUserDn("cn=admin,dc=example,dc=com");
contextSource.setPassword("adminpassword");
return contextSource;
}
Additional Resources
- Spring LDAP Documentation
- LDAP Connection 101
Issue 2: Search Filter Errors
Another common issue when working with Spring LDAP is encountering errors with search filters. This can lead to unexpected or no search results when querying the LDAP server.
Troubleshooting Steps
When troubleshooting search filter errors, verify the search filter syntax and ensure that it conforms to the LDAP query language. Also, make sure that the base DN and search scope are correctly configured in the LDAP query.
public List<String> search(String filter) {
LdapTemplate ldapTemplate = new LdapTemplate(contextSource());
ldapTemplate.setIgnorePartialResultException(true);
return ldapTemplate.search(
LdapQueryBuilder.query()
.base("ou=users,dc=example,dc=com")
.where(filter),
(AttributesMapper<String>) attrs -> attrs.get("cn").get().toString()
);
}
Additional Resources
Issue 3: Authentication and Authorization
Authentication and authorization issues are often encountered when using Spring LDAP for user authentication and access control.
Troubleshooting Steps
When facing authentication or authorization problems, double-check the user credentials provided to the LDAP authentication manager and ensure that the user is authorized to perform the requested operation.
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth
.ldapAuthentication()
.contextSource(contextSource())
.userDnPatterns("uid={0},ou=users")
.groupSearchBase("ou=groups")
.groupSearchFilter("member={0}");
}
Additional Resources
- LDAP Authentication
- Spring LDAP Authentication
Issue 4: SSL/TLS Configuration
When configuring secure communication with the LDAP server using SSL/TLS, developers may encounter issues with certificate validation, truststore, or keystore configuration.
Troubleshooting Steps
When facing SSL/TLS configuration issues, ensure that the LDAP server's SSL certificate is valid and trusted. Verify the truststore and keystore configuration in the Spring application to establish secure connections.
@Bean
public LdapContextSource contextSource() {
LdapContextSource contextSource = new LdapContextSource();
contextSource.setUrl("ldaps://ldap.example.com:636");
contextSource.setBase("dc=example,dc=com");
contextSource.setUserDn("cn=admin,dc=example,dc=com");
contextSource.setPassword("adminpassword");
contextSource.setPooled(true);
contextSource.setBaseEnvironmentProperties(sslEnvironmentProperties());
return contextSource;
}
private Properties sslEnvironmentProperties() {
Properties env = new Properties();
env.setProperty("java.naming.ldap.factory.socket", "com.example.CustomSSLSocketFactory");
// Additional SSL/TLS properties
return env;
}
Additional Resources
- LDAP SSL/TLS Configuration
- Spring LDAP SSL Configuration
Wrapping Up
In this post, we have discussed common issues that developers may encounter when working with Spring LDAP 2.0.0 and provided troubleshooting steps to address these issues. By following these steps and leveraging the resources provided, developers can effectively troubleshoot and resolve issues related to connection problems, search filter errors, authentication, authorization, and SSL/TLS configuration in their Spring LDAP applications.
Remember, troubleshooting issues with Spring LDAP may require a combination of understanding LDAP concepts, reviewing Spring LDAP documentation, and careful examination of the application's configuration. With patience and attention to detail, these common issues can be successfully resolved, allowing developers to harness the full power of Spring LDAP in their Java applications.