Optimizing Spring Batch Step Partitioning for Scalability
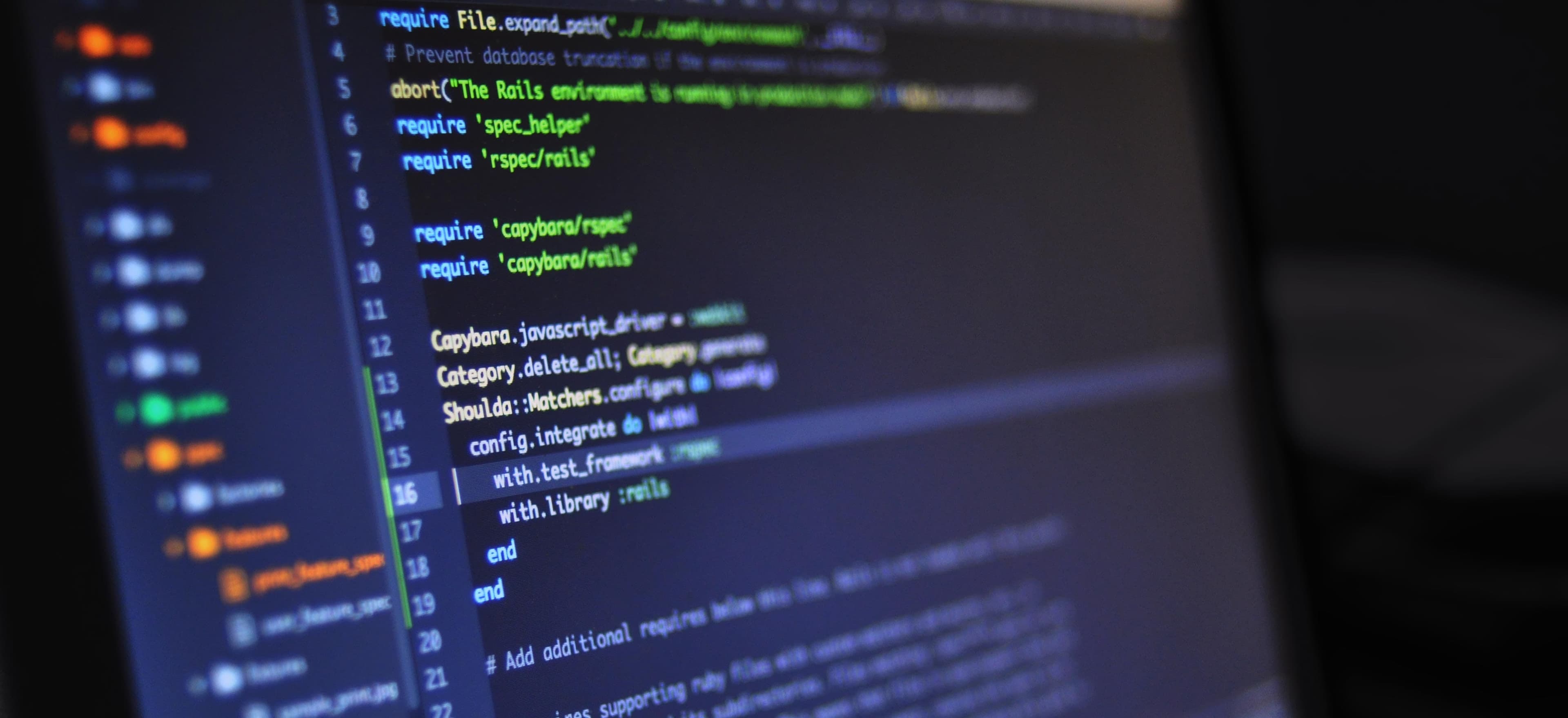
- Published on
Optimizing Spring Batch Step Partitioning for Scalability
When dealing with large dataset processing, it's essential to ensure that our batch jobs are scalable and efficient. Spring Batch provides a powerful feature called Step Partitioning, which allows us to divide a batch job into multiple parallel processes to handle large volumes of data. However, to truly harness the power of step partitioning, we need to optimize our configuration for scalability. In this article, we will explore techniques to optimize Spring Batch Step Partitioning for scalability.
Understanding Step Partitioning
Before delving into optimization techniques, let's grasp the concept of Step Partitioning in Spring Batch. Step Partitioning is a feature that enables us to split the processing of a step into multiple threads or processes, each handling a subset of the input data. This technique is particularly useful when dealing with large datasets since it allows us to process data in parallel, significantly improving performance.
With Spring Batch's Step Partitioning, a master step delegates the work to multiple worker steps, where each worker processes a distinct partition of the input data. The master step orchestrates the overall execution and combines the results obtained from the worker steps. This approach distributes the workload across multiple threads, thereby enhancing the efficiency and scalability of batch processing.
Optimizing Step Partitioning for Scalability
Optimizing the configuration of Step Partitioning is crucial to achieve scalability and performance. Let's explore several techniques to optimize Spring Batch Step Partitioning.
1. Partitioning Strategy
The choice of partitioning strategy plays a pivotal role in the performance of Step Partitioning. Spring Batch offers various partitioning strategies such as range partitioning, multicolumn range partitioning, and custom partitioning. When selecting a partitioning strategy, consider the characteristics of the input data and the nature of processing.
Range Partitioning
Range partitioning is suitable when the input data can be evenly divided based on a range of keys. This strategy ensures that each partition receives a roughly equal amount of data, promoting balanced workload distribution. It is effective for scenarios where the input data can be logically divided into contiguous ranges, such as processing data based on a numeric or date range.
Multicolumn Range Partitioning
Multicolumn range partitioning extends the concept of range partitioning by allowing partitioning based on multiple columns. This strategy is beneficial when the input data needs to be partitioned considering multiple attributes, providing more flexibility in defining the partitions.
Custom Partitioning
In cases where the standard partitioning strategies do not suffice, custom partitioning comes into play. Custom partitioning empowers us to tailor the partitioning logic based on our specific requirements. It grants the freedom to define partitioning logic that aligns with the intricacies of the input data and the processing requirements.
Choosing the appropriate partitioning strategy is essential for distributing the workload effectively and optimizing the performance of Step Partitioning.
2. Data Volume Assessment
Efficient partitioning relies on a thorough assessment of the data volume. Understanding the size and distribution of the input data is imperative in determining the optimal number of partitions. Oversized partitions may lead to uneven distribution of workload, while overly fine-grained partitions could induce overhead due to increased coordination overhead.
By analyzing the characteristics of the input data, such as range distribution, cardinality, and data skew, we can make informed decisions about the number and size of partitions, ensuring an equitable distribution of workload and efficient resource utilization.
3. Throttling and Parallelism
Throttling and parallelism management are integral aspects of Step Partitioning optimization. Controlling the degree of parallelism ensures that the system operates within its capacity without overwhelming the resources. Moreover, applying proper throttling mechanisms prevents resource contention and optimizes the throughput of the processing.
Spring Batch provides mechanisms to control parallelism, such as setting the maximum number of concurrent executions, configuring thread pools, and managing thread allocation. By fine-tuning these parameters based on the resource capabilities and workload characteristics, we can achieve optimal parallelism and throughput, thereby enhancing scalability.
4. Remote Partitioning
In scenarios where the workers need to operate in separate JVMs, such as distributed environments or when the processing requires different resources or dependencies, leveraging Spring Batch's Remote Partitioning is advantageous. Remote Partitioning facilitates the distribution of step execution across JVM boundaries, allowing for horizontal scalability and efficient utilization of resources.
By utilizing Remote Partitioning, we can harness the computational power of multiple nodes, distributing the processing workload across diverse environments, and achieving enhanced scalability.
Example Code Implementation
Let's exemplify the discussed optimization techniques through a sample implementation using Spring Batch Step Partitioning.
Range Partitioning Configuration
@Bean
public Step partitionedStep(ItemReader<MyEntity> itemReader, ItemProcessor<MyEntity, ProcessedEntity> itemProcessor,
ItemWriter<ProcessedEntity> itemWriter, TaskExecutor taskExecutor) {
return stepBuilderFactory.get("partitionedStep")
.partitioner(workerStep().getName(), rangePartitioner())
.step(workerStep())
.gridSize(5) // Number of partitions
.taskExecutor(taskExecutor)
.build();
}
@Bean
@StepScope
public Partitioner rangePartitioner() {
return new RangePartitioner();
}
@Bean
public Step workerStep() {
return stepBuilderFactory.get("workerStep")
.<MyEntity, ProcessedEntity>chunk(100)
.reader(itemReader)
.processor(itemProcessor)
.writer(itemWriter)
.build();
}
In this example, we configure a Step with range partitioning strategy, specifying the number of partitions and utilizing a custom RangePartitioner
.
By following the optimization techniques mentioned earlier and tailoring the partitioning logic, we have optimized the Step Partitioning for scalability.
My Closing Thoughts on the Matter
Optimizing Spring Batch Step Partitioning for scalability is essential for efficient processing of large datasets. By carefully selecting partitioning strategies, assessing data volume, managing parallelism, and leveraging remote partitioning when necessary, we can enhance the scalability and performance of our batch jobs.
Ensuring optimal partitioning not only improves the efficiency of batch processing but also enables us to harness the computational resources effectively. By incorporating the discussed optimization techniques into our Spring Batch Step Partitioning configuration, we can achieve enhanced scalability and performance, thereby catering to the demands of large-scale data processing.
In conclusion, optimizing Spring Batch Step Partitioning empowers us to scale our batch jobs efficiently, making them resilient and high-performing in handling massive datasets.
Implementing optimization techniques in Spring Batch Step Partitioning configuration paves the way for robust and scalable batch processing, offering a competitive advantage in handling large-scale data operations.
By harnessing the power of Step Partitioning and adopting optimization best practices, we can propel our batch jobs to new heights of scalability and efficiency.
Scaling Spring Batch Step Partitioning is pivotal in addressing modern data processing demands, and optimization is the key that unlocks its full potential.