Optimizing Session Management in Spring Boot
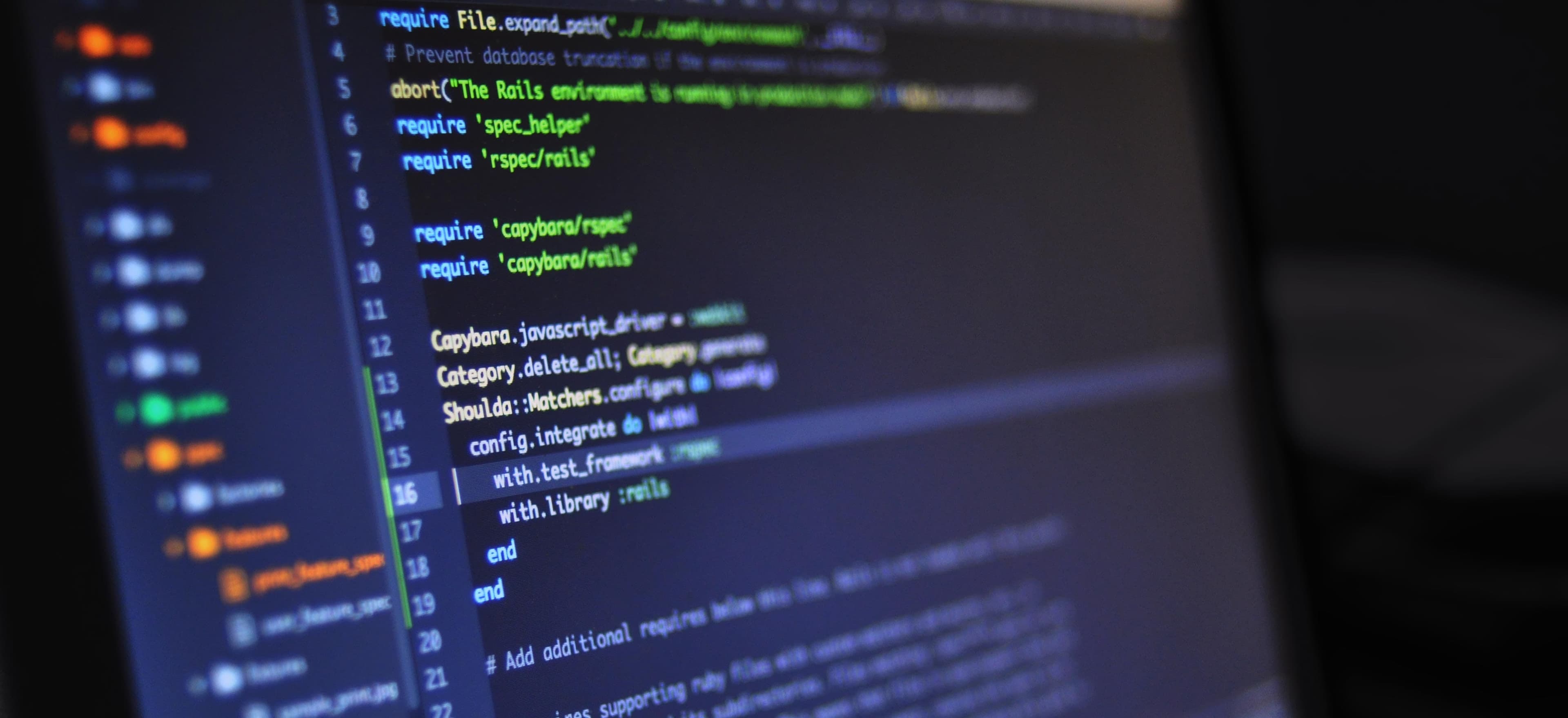
- Published on
Optimizing Session Management in Spring Boot
When it comes to web applications, session management plays a crucial role in maintaining user state across multiple requests. In a Java Spring Boot application, efficient session management is essential for scalability, security, and performance. In this article, we'll explore various strategies to optimize session management in a Spring Boot application, along with best practices to ensure a robust and secure implementation.
Understanding Session Management in Spring Boot
In Spring Boot, session management is primarily handled by the underlying servlet container, typically Tomcat, Jetty, or Undertow. The session data can be stored either on the client side using cookies or on the server side. By default, Spring Boot uses server-side session storage, with the data being stored in memory.
Optimizing Session Storage
1. Using Redis for Session Storage
Storing session data in-memory may not be suitable for applications requiring scalability and high availability. Integrating Redis, an in-memory data store, for session storage can significantly improve performance and reliability. Spring Session provides seamless integration with Redis for managing distributed sessions. By storing session data in Redis, you can ensure that sessions are resilient to server failures and easily share session data across multiple application instances.
Example Configuration:
@Configuration
@EnableRedisHttpSession
public class SessionConfig extends AbstractHttpSessionApplicationInitializer {
@Bean
public LettuceConnectionFactory connectionFactory() {
return new LettuceConnectionFactory();
}
}
In the above example, @EnableRedisHttpSession
enables Redis-backed session management, while the LettuceConnectionFactory
provides the connection to the Redis server.
2. Using Database for Session Storage
For even greater persistence and fault tolerance, storing session data in a database such as MySQL or PostgreSQL can be a viable option. Spring Session also offers support for JDBC-backed session storage, allowing seamless integration with relational databases.
Example Configuration:
@Configuration
@EnableJdbcHttpSession
public class SessionConfig extends AbstractHttpSessionApplicationInitializer {
@Bean
public JdbcOperationsSessionRepository sessionRepository() {
return new JdbcOperationsSessionRepository(jdbcTemplate);
}
}
In this example, @EnableJdbcHttpSession
enables JDBC-backed session management, and JdbcOperationsSessionRepository
is used to store and retrieve session data from the database.
By utilizing Redis or a database for session storage, you can ensure that the session data is durable, distributed, and highly available.
Session Timeout Configuration
Configuring session timeouts is vital to ensure that inactive sessions are invalidated, freeing up server resources. It's essential to strike a balance between user experience and resource utilization by setting an appropriate session timeout value.
Example Configuration:
server:
servlet:
session:
timeout: 30m
In this example, the session timeout is set to 30 minutes, ensuring that inactive sessions are invalidated after the specified period.
Session Fixation Protection
Session fixation is a security vulnerability where an attacker sets a user's session identifier before the user authenticates, potentially compromising the user's session. Spring Security provides built-in protection against session fixation attacks by regenerating the session identifier upon authentication.
Example Configuration:
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.sessionManagement()
.sessionFixation()
.newSession();
}
}
In this configuration, sessionFixation().newSession()
instructs Spring Security to generate a new session after successful authentication, effectively mitigating session fixation risks.
Container-based Session Management
When deploying a Spring Boot application to a containerized environment, such as Docker or Kubernetes, it's crucial to consider session management in a stateless fashion. Utilizing technologies like JWT (JSON Web Tokens) for stateless authentication and session management can eliminate the need for server-side session storage altogether, providing a scalable and distributed approach to managing user sessions.
Example Implementation with JWT:
// (JWT implementation code goes here)
By leveraging container-based session management with JWT, you can achieve seamless scalability and eliminate the overhead of server-side session storage.
Closing the Chapter
Efficient session management is fundamental to the performance, scalability, and security of a Spring Boot application. By optimizing session storage, configuring appropriate timeouts, and considering stateless session management, you can ensure a robust and secure session management strategy tailored to your application's requirements.
Optimizing session management not only enhances the user experience but also contributes to the overall stability and performance of your Spring Boot application. By implementing the strategies and best practices discussed in this article, you can maximize the efficiency and security of session management in your Spring Boot applications.
For further reading and implementation details, refer to the official Spring Session documentation and Spring Security documentation.