Testing Failure: Ensuring OpenShift MongoDB App Reliability
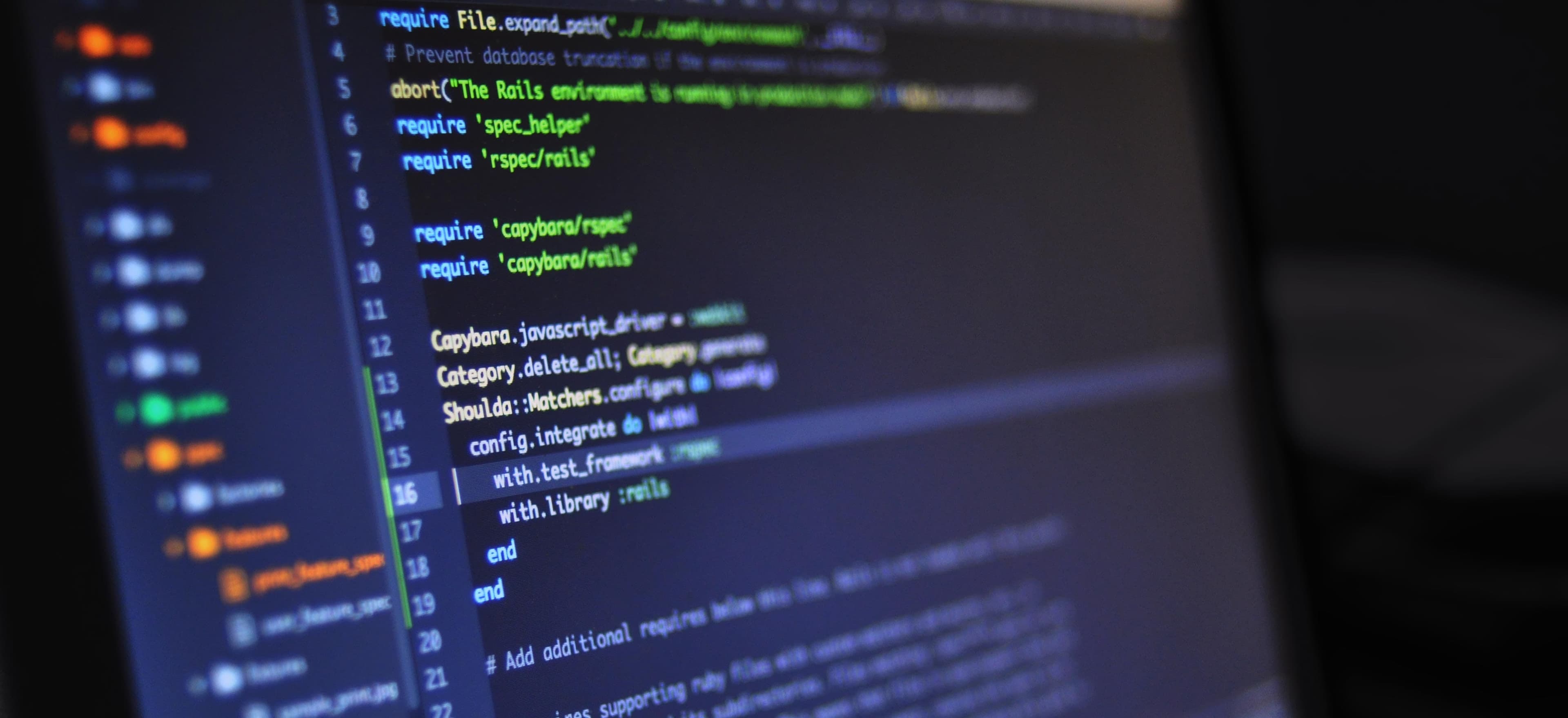
- Published on
Testing Failure: Ensuring OpenShift MongoDB App Reliability
When developing and deploying applications, guaranteeing their reliability is crucial. This is even more significant while working with databases, as any failure can lead to significant data loss and application disruption. In this blog post, we will explore how to ensure the reliability of a MongoDB database within an OpenShift environment by implementing effective testing strategies.
Understanding the Importance of Reliability Testing
Reliability testing is vital to ensure that an application can withstand adverse conditions without failure. As the backbone of many applications, databases must be thoroughly tested to confirm their ability to recover from potential failures. OpenShift is a popular platform for hosting applications, leveraging Kubernetes to manage containerized workloads. MongoDB, as a NoSQL database, offers significant flexibility and scalability, making it a popular choice for many applications deployed on OpenShift.
Employing Robust Testing Strategies
Integration Testing with MongoDB
During integration testing, it's crucial to simulate different failure scenarios to observe how the MongoDB database behaves. For instance, deliberately stopping the MongoDB service to see how the application responds can provide valuable insights.
@Test
public void testMongoDBConnectionFailure() {
// Simulate MongoDB service failure
mongoDBService.stopService();
// Verify application response to MongoDB failure
// Assert.assertEquals(expectedResult, actualResult);
}
In the above code snippet, a test case simulates the failure of the MongoDB service and then verifies the application's response. Understanding how the application handles such failures is essential for identifying potential weak points.
Utilizing Chaos Engineering Principles
Chaos engineering involves deliberately injecting failures into a system to assess its resilience. Tools such as Chaos Monkey can be employed to randomly terminate MongoDB pods within an OpenShift cluster, allowing for real-time observation of the system's behavior under stress.
Implementing Automated Backup and Restore Tests
Automated tests for database backup and restore processes are essential for ensuring data recoverability in the event of a failure. By automating these tests, developers can verify the integrity of the backup processes and the ability to restore data accurately.
@Test
public void testDatabaseBackupAndRestore() {
// Simulate data loss
databaseService.deleteData();
// Restore data from backup
// Assert.assertEquals(originalData, restoredData);
}
By testing the backup and restore processes, teams can verify the reliability of their disaster recovery mechanisms.
Leveraging OpenShift for Reliability Testing
OpenShift provides robust features for implementing reliability testing in a MongoDB environment. By utilizing features such as StatefulSets for managing MongoDB pods and Kubernetes liveness and readiness probes, developers can create a resilient infrastructure.
StatefulSets for Persistent Storage
StatefulSets in Kubernetes are ideal for managing stateful applications like MongoDB. They ensure stable, unique network identifiers and persistent storage, enabling reliable data storage even in the event of pod failures.
Liveness and Readiness Probes
Liveness and readiness probes are essential for monitoring the health of MongoDB pods. By configuring these probes, developers can ensure that only healthy instances serve traffic, effectively preventing potential data corruption.
Wrapping Up
Ensuring the reliability of MongoDB within an OpenShift environment is imperative for the overall stability of applications. By implementing comprehensive testing strategies, utilizing OpenShift’s features, and embracing chaos engineering principles, developers can confidently deploy MongoDB-backed applications with the assurance of resilience in the face of failures.
In conclusion, reliability testing is a fundamental aspect of ensuring the robustness of applications deployed on OpenShift with MongoDB, and it is essential for maintaining the integrity of data and the seamless operation of the entire system.
By following these best practices and harnessing the capabilities of OpenShift and MongoDB, developers can fortify their applications against potential data loss and downtime, thus delivering a reliable and resilient experience for end-users.
Remember, the goal of testing failure is not to cause chaos, but to ensure that chaos doesn't cause catastrophic repercussions.