Maximizing Java Performance with ConstantDynamic
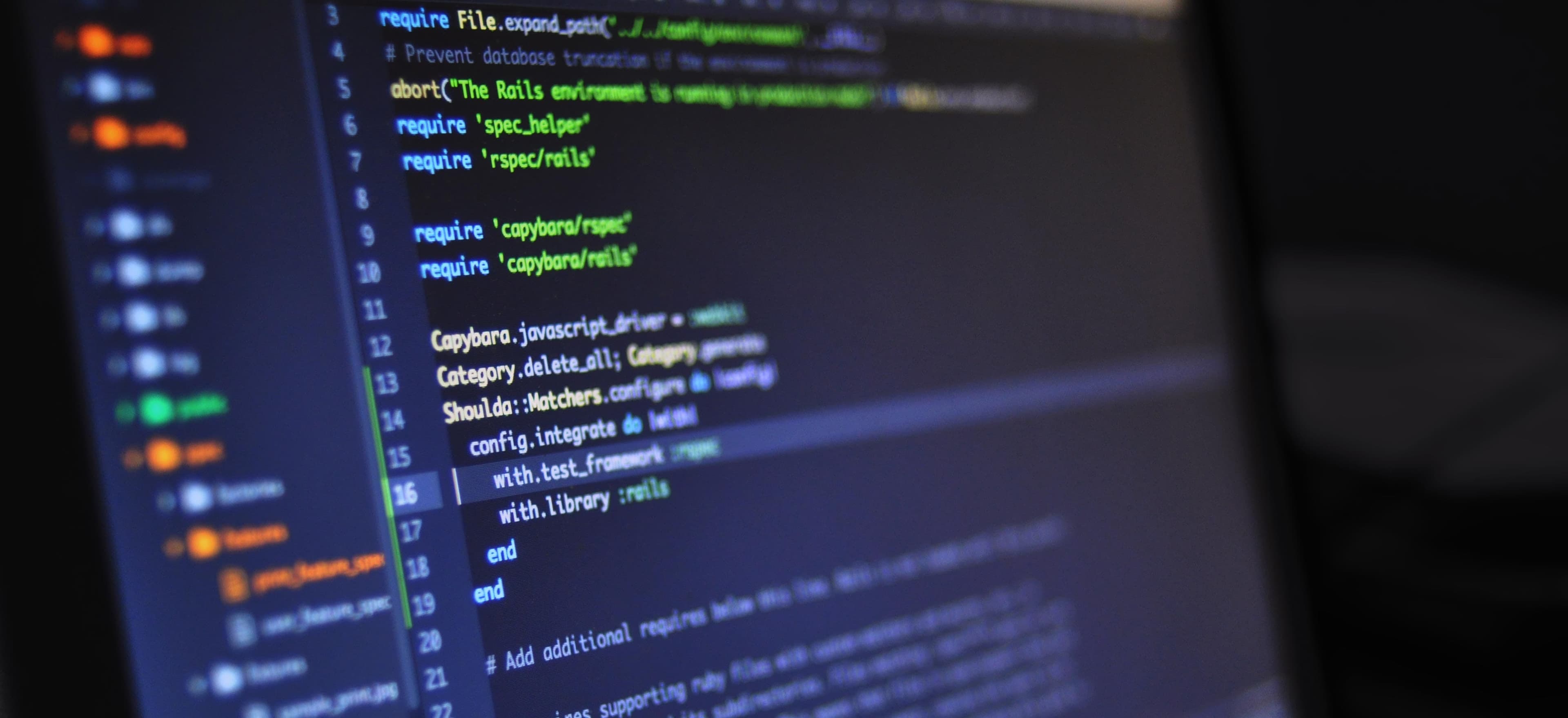
- Published on
Maximizing Java Performance with ConstantDynamic
When it comes to optimizing the performance of Java applications, developers often focus on efficient algorithms, data structures, and runtime optimizations. However, one often overlooked aspect of performance tuning is the use of ConstantDynamic
introduced in Java 11. In this article, we will explore how ConstantDynamic
can be leveraged to improve the performance of Java applications.
Understanding ConstantDynamic
Before delving into the performance benefits of ConstantDynamic
, let's take a moment to understand what it actually is. ConstantDynamic
is a new constant-pool form introduced in Java 11 that allows for more efficient representation of dynamically-computed constants.
In the past, static constants were added into the constant pool, which could lead to unnecessary memory consumption and hinder performance. With ConstantDynamic
, the constant pool can now hold references to dynamically-computed constants, avoiding the need to precompute and store them in the constant pool.
Why ConstantDynamic?
The use of ConstantDynamic
brings several advantages when it comes to enhancing Java application performance:
-
Reduced Memory Consumption: By allowing dynamically-computed constants to be represented more efficiently,
ConstantDynamic
reduces the memory footprint of Java applications. -
Improved Startup Time: The avoidance of precomputing and storing constants in the constant pool can lead to faster application startup times, especially in scenarios where a large number of constants are involved.
-
Enhanced Flexibility: With
ConstantDynamic
, developers have more flexibility in representing constants, enabling them to adapt to dynamic changes more effectively.
Leveraging ConstantDynamic for Performance
Now that we understand the benefits of ConstantDynamic
, let's explore how we can leverage it to enhance the performance of Java applications. Here are a few scenarios where ConstantDynamic
can make a significant impact:
Dynamic Configuration Loading
In many applications, configuration values are loaded from external sources such as property files, databases, or environment variables. Traditionally, these configuration values are loaded and stored as static constants, potentially bloating the constant pool with values that are only known at runtime.
By using ConstantDynamic
, we can represent these dynamically-loaded configuration values more efficiently, reducing the memory overhead and improving the overall performance of configuration loading.
public class AppConfig {
public static final Object CONFIG_VALUE =
// Load configuration value dynamically
// Using ConstantDynamic to store dynamically-computed constants
}
Lazy Initialization and Caching
In scenarios where lazy initialization and caching are employed, computed constants are often stored statically, adding unnecessary overhead to the constant pool. By utilizing ConstantDynamic
, the dynamically-computed constants can be represented more efficiently, reducing the memory footprint and potentially improving the performance of lazy initialization and caching mechanisms.
public class Cache {
public static final Object CACHED_VALUE =
// Compute and cache value dynamically
// Utilizing ConstantDynamic for dynamic constant representation
}
Dynamic Feature Flags
Feature flags are commonly used to enable or disable certain features in an application. These flags are often represented as static constants, adding to the size of the constant pool. By leveraging ConstantDynamic
, dynamically-computed feature flag values can be represented more efficiently, leading to reduced memory consumption and potentially faster runtime evaluation of feature flags.
public class FeatureFlags {
public static final boolean NEW_FEATURE_ENABLED =
// Determine feature flag dynamically
// Using ConstantDynamic for efficient representation of feature flag constants
}
In Conclusion, Here is What Matters
In conclusion, the introduction of ConstantDynamic
in Java 11 provides an effective mechanism for representing dynamically-computed constants more efficiently. By leveraging ConstantDynamic
, developers can reduce memory consumption, improve startup times, and enhance the flexibility of constant representation in Java applications.
When it comes to maximizing the performance of Java applications, considering the use of ConstantDynamic
for dynamically-computed constants can lead to significant gains in memory efficiency and overall application performance.
Incorporating ConstantDynamic
into your codebase can pave the way for a more optimized and efficient Java application, contributing to a better user experience and improved resource utilization.
For further information on Java performance optimization and dynamic constant representation, refer to the official Java documentation.
Start leveraging ConstantDynamic
today to unlock the full potential of Java performance optimization!