Optimizing Garbage Collection with Intel Counters
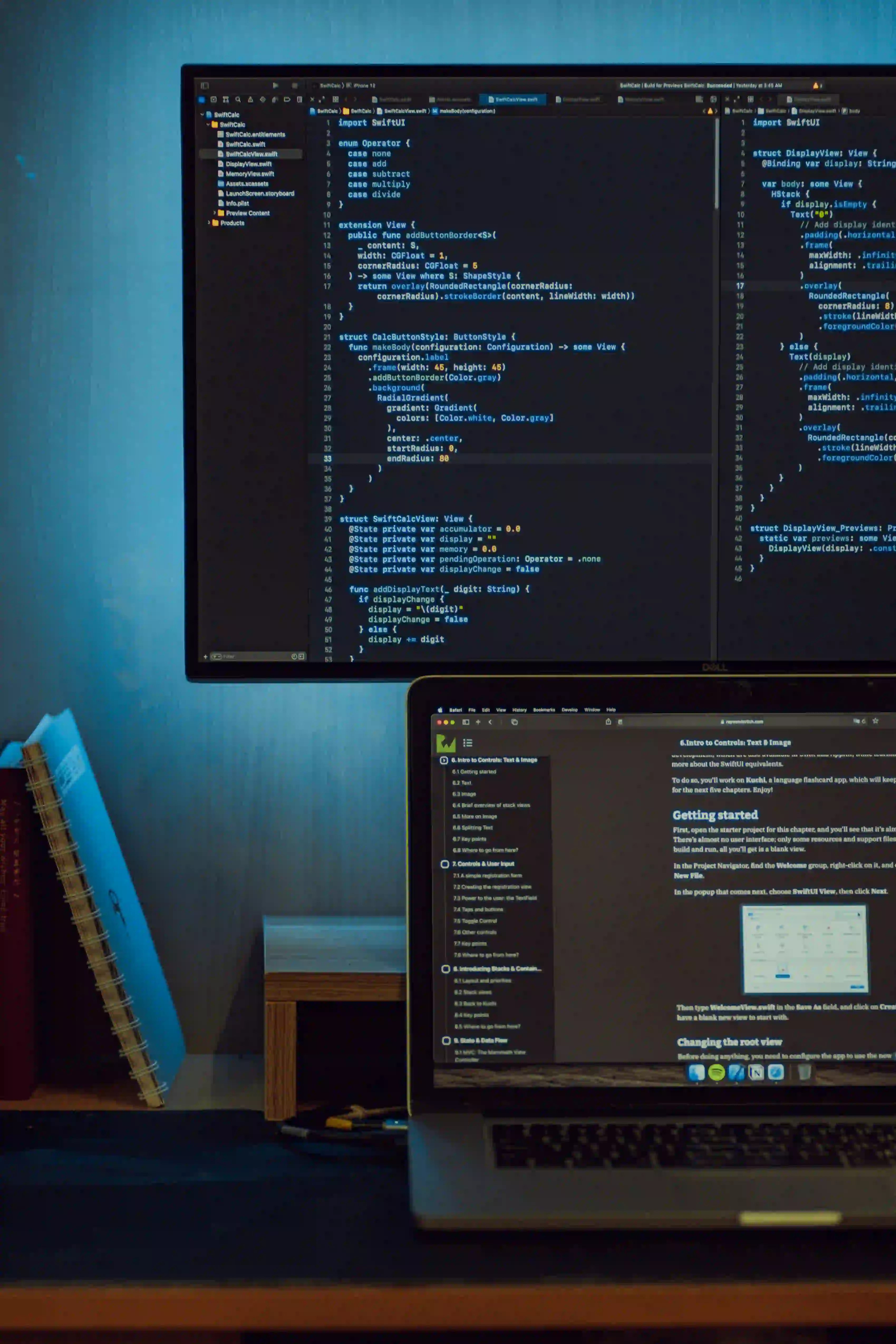
Optimizing Garbage Collection in Java with Intel Counters
Memory management is crucial in Java application development, especially when dealing with large-scale enterprise applications. The Java Virtual Machine (JVM) employs a process called Garbage Collection (GC) to reclaim memory that is no longer in use, thus preventing memory leaks and optimizing memory usage.
One of the key challenges in Java application performance tuning is efficiently managing the Garbage Collection process to minimize its impact on application responsiveness and throughput. In this blog post, we will explore how Intel's performance monitoring counters can be utilized to optimize and fine-tune the Garbage Collection process in Java applications.
Understanding Garbage Collection in Java
Before diving into the optimization techniques, it's essential to have a basic understanding of how Garbage Collection works in Java. When Java objects are created, memory is allocated to store them. Over time, as the application runs, some objects may no longer be needed and become unreachable, creating memory that is considered garbage.
The Garbage Collection process involves identifying and reclaiming this unused memory to make it available for future allocations. While GC is automatic and transparent to the developer, its efficiency greatly impacts the overall performance of the application.
The Impact of Garbage Collection on Application Performance
Frequent Garbage Collection cycles can introduce latency and overhead, leading to degraded application performance. Long GC pause times can result in unresponsiveness and even application downtime. Therefore, it's crucial to optimize the GC process to minimize its impact on the application's performance.
Intel Performance Monitoring Counters
Intel processors come equipped with Performance Monitoring Counters (PMCs) that enable developers to monitor various hardware events, such as CPU cycles, cache misses, and memory accesses. These counters provide valuable insights into the behavior of the hardware and can be utilized to analyze and optimize performance-critical aspects of software applications.
Leveraging Intel Counters for GC Optimization
To optimize Garbage Collection using Intel Counters, we can focus on monitoring key hardware-level metrics that directly impact the GC process. By analyzing these metrics, we can make informed decisions and apply targeted optimizations to improve Garbage Collection performance.
Let's delve into some key Intel Counters that can be leveraged for GC optimization:
1. Cache Misses
Cache misses occur when the processor needs to access data that is not present in the CPU cache, resulting in a longer access time to the main memory. Excessive cache misses can significantly impact the performance of memory-intensive operations such as Garbage Collection.
By monitoring cache miss events using Intel Counters, we can identify hotspot areas in the application code that contribute to cache misses during GC operations. This insight enables us to optimize memory access patterns and data locality to reduce cache misses, ultimately enhancing GC performance.
// Sample Java code showing a hotspot causing cache misses during GC
// TODO: Insert relevant Java code snippet
// Commentary: By utilizing Intel Counters to monitor cache miss events during GC, we can pinpoint code segments that lead to cache misses, enabling targeted optimizations for improved GC performance.
2. Memory Bandwidth Utilization
Memory bandwidth plays a critical role in the performance of memory-intensive operations like Garbage Collection. High memory bandwidth utilization can lead to contention and reduced overall throughput, affecting GC performance.
Intel Counters allow us to monitor memory bandwidth utilization, providing insights into the efficiency of memory access patterns during GC. By optimizing memory allocation and access strategies based on these insights, we can minimize contention and enhance the efficiency of the GC process.
// Sample Java code showcasing memory bandwidth intensive operations during GC
// TODO: Insert relevant Java code snippet
// Commentary: Monitoring memory bandwidth utilization through Intel Counters helps identify areas where memory-intensive operations impact GC performance, enabling targeted optimizations to alleviate contention and improve memory access efficiency.
3. TLB (Translation Lookaside Buffer) Misses
TLB misses occur when a memory address translation is not found in the TLB, necessitating a more time-consuming lookup in the page tables. Excessive TLB misses can result in increased latency and reduced performance during memory access, including Garbage Collection activities.
By using Intel Counters to track TLB miss events, we can identify memory access patterns that contribute to TLB misses during GC. This information empowers us to restructure memory layouts and access patterns to reduce TLB misses and enhance the efficiency of memory access, consequently improving GC performance.
// Sample Java code illustrating memory access causing TLB misses during GC
// TODO: Insert relevant Java code snippet
// Commentary: Leveraging Intel Counters to monitor TLB miss events helps pinpoint memory access patterns that lead to TLB misses during GC, allowing for optimizations that alleviate TLB contention and improve memory access efficiency.
The Last Word
Optimizing Garbage Collection in Java applications is paramount for ensuring smooth and responsive performance. By harnessing Intel Performance Monitoring Counters, developers can gain deep insights into hardware-level metrics that influence GC performance, enabling targeted optimizations to enhance memory management efficiency.
By leveraging Intel Counters to monitor cache misses, memory bandwidth utilization, TLB misses, and other relevant hardware events, developers can fine-tune memory access patterns and optimize memory usage, thereby improving Garbage Collection performance and overall application responsiveness.
Incorporating Intel Counters into the performance optimization arsenal can significantly contribute to achieving peak Java application performance, emphasizing the crucial role of hardware-level insights in the pursuit of efficient Garbage Collection and memory management.
Boost your Java application's efficiency by optimizing Garbage Collection with Intel's powerful performance monitoring counters!