Getting Started with MicroProfile: Overcoming Configuration Challenges
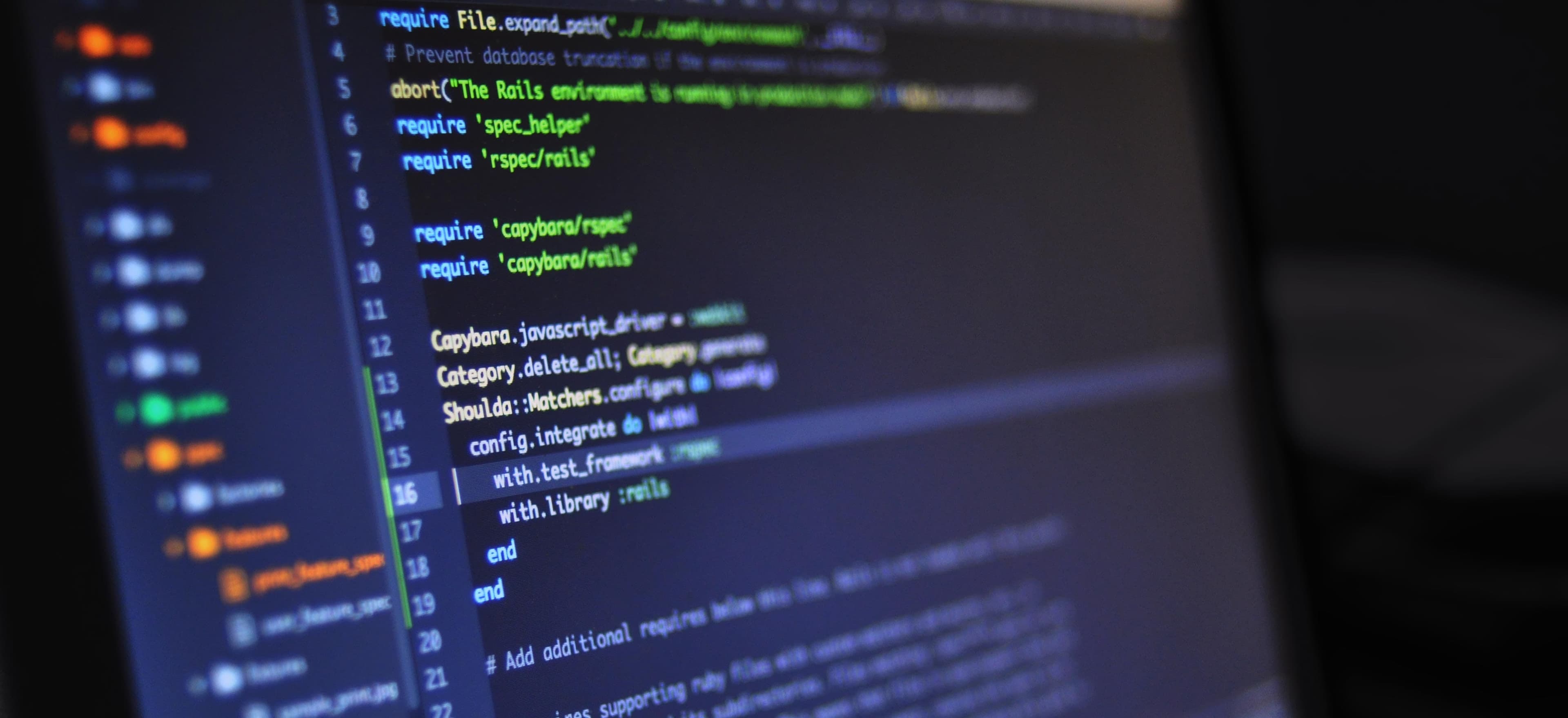
- Published on
The Starting Line
In the world of enterprise Java development, Microservices architecture has become increasingly popular due to its ability to create scalable and flexible applications. MicroProfile, a project within the Eclipse Foundation, aims to optimize Enterprise Java for a microservices architecture. One of the challenges developers often face when building microservices is handling configurations efficiently. In this article, we will explore how MicroProfile helps in overcoming configuration challenges in microservices.
What are MicroProfile Configurations?
Configuration management is one of the critical aspects of microservices. MicroProfile Config provides a straightforward way to externalize configuration from microservices applications. It allows developers to use a common way to access configuration properties and values. MicroProfile Config provides a simple and uniform way to handle configuration from various sources such as environment variables, system properties, properties files, JSON files, and Kubernetes ConfigMaps.
Getting Started with MicroProfile Config
Let's dive into the practical aspects of MicroProfile Config. We'll start with setting up a simple microservice with the help of MicroProfile Config.
Setting up the Project
First, we need to set up a Maven or Gradle project with the necessary dependencies for MicroProfile. We'll use the MicroProfile Starter, which provides an easy way to generate a new project with all the required dependencies. Refer to the MicroProfile Starter to generate a new project with MicroProfile Config dependency.
Once the project is generated, we can start by adding the MicroProfile Config dependency to the pom.xml
(for Maven) or build.gradle
(for Gradle) file.
Creating a Configuration Property
In our microservice, we want to retrieve the configuration value for the maxItemCount
property. We can define it in a microprofile-config.properties
file:
maxItemCount=100
Injecting Configuration Property
Now, let's inject and use the maxItemCount
property in our microservice code. We can use the @Inject
and @ConfigProperty
annotations to achieve this:
import org.eclipse.microprofile.config.inject.ConfigProperty;
import javax.inject.Inject;
public class ItemService {
@Inject
@ConfigProperty(name = "maxItemCount")
private int maxItemCount;
// ... rest of the code
}
In the above code snippet, we have injected the maxItemCount
configuration property using the @Inject
and @ConfigProperty
annotations. This allows us to directly access the value of the property in our code.
Default Values and Optional Properties
MicroProfile Config also provides support for specifying default values for configuration properties. If the property is not set, the default value will be used. Additionally, you can mark properties as optional, which means that if a property is not found, it will not cause an error.
@Inject
@ConfigProperty(name = "defaultItemCount", defaultValue = "50")
private int defaultItemCount;
@Inject
@ConfigProperty(name = "optionalProperty", defaultValue = "N/A", required = false)
private String optionalProperty;
In the above example, defaultItemCount
has a default value of 50, and optionalProperty
is marked as optional with a default value of "N/A".
Using Multiple Configuration Sources
MicroProfile Config allows you to use multiple configuration sources and specify the order in which they should be used. By default, the sources are queried in a predefined order, but you can override this order by setting the ordinal
attribute.
For instance, if you want to prioritize system properties over environment variables, you can use the ordinal
attribute to define the order:
@ConfigProperty(name = "databaseUrl", defaultValue = "jdbc:postgresql://localhost:5432/mydb", ordinal = 200)
private String databaseUrl;
In the example above, the system property source will be prioritized over other sources due to the higher ordinal value.
Dynamic Configuration Updates
One of the powerful features of MicroProfile Config is the ability to handle dynamic configuration updates without restarting the microservice. This is extremely useful as it allows you to modify configuration values at runtime without any downtime.
To demonstrate this, let's consider a scenario where we want to dynamically update the maxItemCount
property. We can achieve this by using a ConfigProvider
to access the configuration and then updating the value as needed:
import org.eclipse.microprofile.config.ConfigProvider;
public class ConfigurationUpdater {
public void updateMaxItemCount(int newMaxItemCount) {
ConfigProvider.getConfig()
.getOptionalValue("maxItemCount", Integer.class)
.ifPresent(currentValue -> {
// Perform validation if needed
// Update the config value
// This change will reflect immediately without restarting the microservice
});
}
}
In the above example, ConfigProvider.getConfig()
is used to access the current configuration and update the maxItemCount
property without requiring a restart.
My Closing Thoughts on the Matter
In this article, we've explored how MicroProfile Config helps in overcoming configuration challenges in microservices. We've learned how to effectively use MicroProfile Config to externalize configuration, inject configuration properties, handle default values and optional properties, use multiple configuration sources, and handle dynamic configuration updates.
MicroProfile Config provides a powerful and flexible way to manage configurations in microservices, making it easier to build and maintain scalable and resilient applications. By externalizing configurations, microservices become more adaptable to different environments and can be easily fine-tuned without redeploying the application.
MicroProfile Config is an essential tool for building microservices that can adapt to changing requirements and environments, and it significantly simplifies the management of configurations in a microservices architecture.
For further exploration, you can refer to the MicroProfile Config documentation and experiment with more advanced features and use cases.
By incorporating MicroProfile Config into your microservices architecture, you can streamline the configuration management process, making your applications more dynamic and adaptable. Whether you're a seasoned developer or new to microservices, optimizing configuration management through MicroProfile can unlock new possibilities for your projects.