Reactive Spring Data: Slow Streaming Live Updates
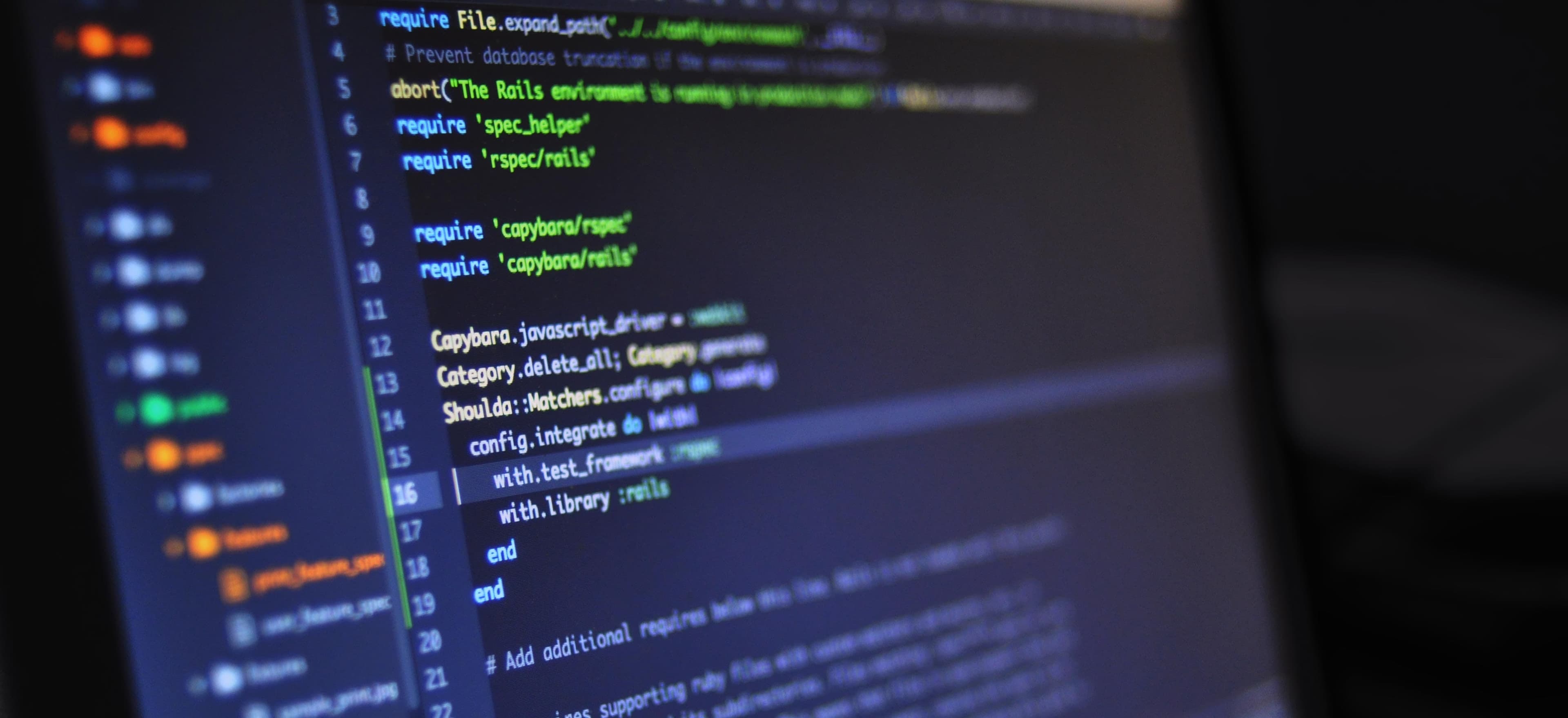
- Published on
Understanding Slow Streaming Live Updates in Reactive Spring Data
Reactive programming has gained immense popularity in recent years due to its ability to handle streams of data efficiently. When it comes to live updates in a reactive application, it's crucial to understand the potential bottlenecks, especially when dealing with slow streaming. In this article, we'll delve into the concept of slow streaming live updates in the context of Reactive Spring Data.
The Challenge of Slow Streaming Live Updates
In a reactive system, live updates are constantly flowing through the application, and at times, the speed of data processing may not match the speed of data arrival. This situation leads to a backlog of data, causing delays in processing live updates.
Understanding Reactive Streams
Reactive Streams, as implemented in the Spring Framework, provide a way to handle asynchronous, non-blocking streams of data while ensuring backpressure handling. Backpressure is the mechanism by which a fast data producer is signaled to slow down when the data consumer is unable to keep up.
Identifying Bottlenecks in Slow Streaming Live Updates
When dealing with slow streaming live updates in a Reactive Spring Data application, it's essential to identify the potential bottlenecks. Some common areas to look into include:
- Database Queries: Complex or inefficient database queries can slow down the processing of live updates.
- Network Latency: Slow network connections between the application and the data source can lead to delayed live updates.
- Resource Exhaustion: Inadequate system resources such as CPU, memory, or thread pools can hinder the efficient processing of live updates.
Overcoming Slow Streaming Live Updates
To address the challenge of slow streaming live updates in Reactive Spring Data, consider the following approaches:
-
Optimizing Database Queries: Utilize techniques such as indexing, query optimization, and caching to improve the performance of database queries.
// Example of using indexed field in Spring Data MongoDB @Indexed(unique = true) private String username;
By using indexed fields in the database, the query performance can be significantly improved, thus reducing the processing time for live updates.
-
Implementing Backpressure Strategies: Leverage backpressure strategies provided by Reactive Streams to control the flow of data between the publisher and the subscriber. This helps in managing the rate of live updates based on the processing capacity of the application.
// Example of using buffer strategy for backpressure in Project Reactor Flux<Data> liveUpdates = repository.getLiveUpdates() .onBackpressureBuffer(100, BufferOverflowStrategy.DROP_OLDEST);
Here, the
onBackpressureBuffer
operator is used to buffer the live updates and drop the oldest elements when the buffer overflows, preventing the application from being overwhelmed by a high volume of live updates. -
Monitoring and Scaling: Implement monitoring solutions to keep track of the application's performance and scale the system horizontally to distribute the load across multiple instances in case of heavy live update traffic.
To Wrap Things Up
In a Reactive Spring Data application, handling slow streaming live updates requires a thorough understanding of reactive streams, identification of potential bottlenecks, and the implementation of effective strategies to overcome delays in live update processing.
By optimizing database queries, implementing backpressure strategies, and monitoring the system for scaling, you can ensure that your Reactive Spring Data application is equipped to handle slow streaming live updates efficiently.
Reactive programming continues to evolve, and with the right approach and tools, developers can build robust and responsive applications that excel in handling live updates seamlessly.
For more in-depth insights into Reactive Spring Data, check out the official documentation and Reactive Streams API to explore the vast possibilities offered by reactive programming in the context of live data updates.