EJB3 Connection Pooling Best Practices
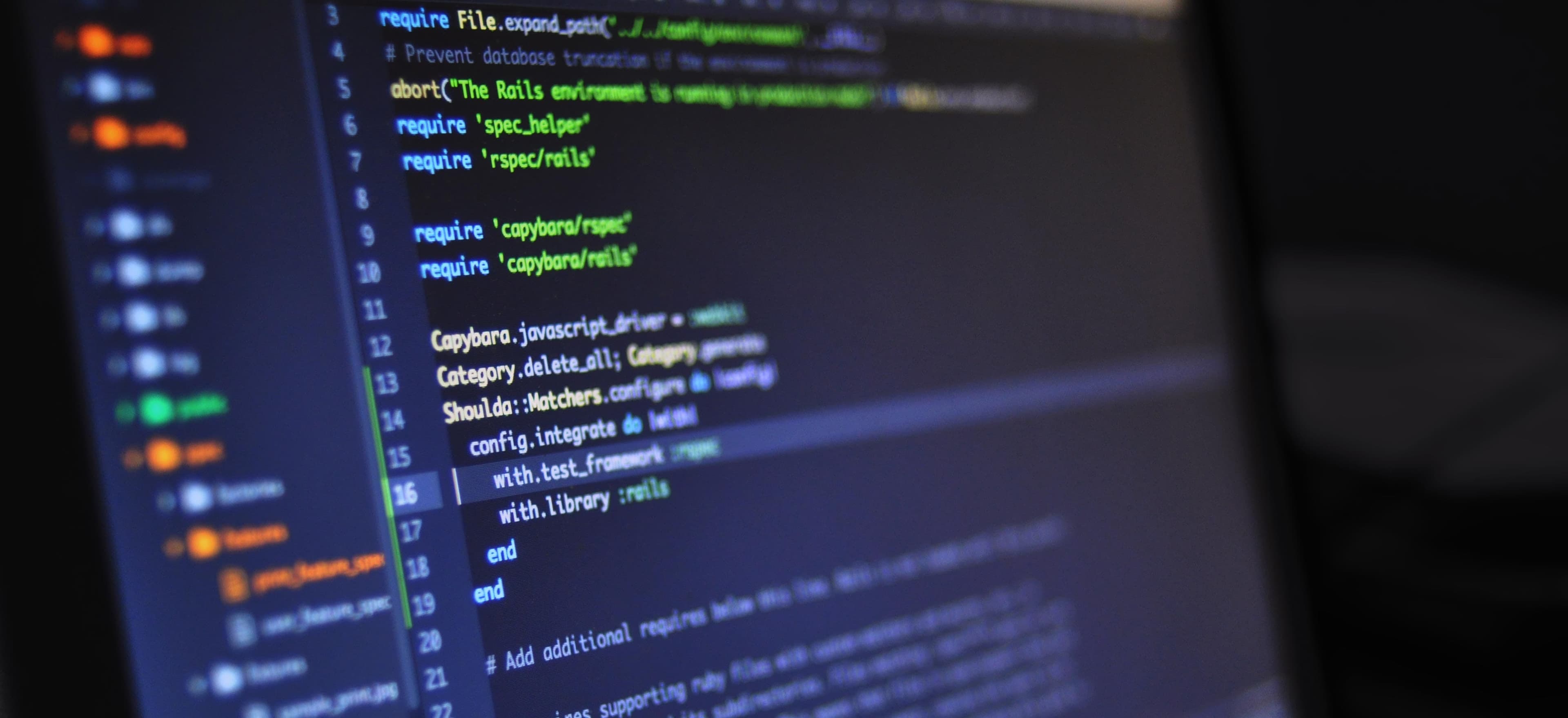
- Published on
The Ultimate Guide to EJB3 Connection Pooling Best Practices
In the world of enterprise Java development, effective use of connection pooling is crucial for optimizing database access and ensuring scalable, high-performance applications. When it comes to EJB3 (Enterprise JavaBeans 3), the right connection pooling strategies can greatly enhance the efficiency and reliability of your applications. In this comprehensive guide, we'll explore the best practices for EJB3 connection pooling, covering everything from the fundamentals to advanced techniques.
Understanding EJB3 and Connection Pooling
Before delving into connection pooling best practices, let's briefly review EJB3 and its relationship with database connection management. EJB3 is a server-side component model for Java EE applications, providing a standardized way to build enterprise-level, scalable, and transactional components. With EJB3, developers can focus on business logic while delegating the management of various concerns, including database interactions, to the EJB container.
One critical aspect of efficient database interaction in EJB3 applications is connection pooling. Connection pooling allows the application to reuse existing database connections, minimizing the overhead of creating and destroying connections for each database operation. This not only improves performance but also prevents resource exhaustion by limiting the number of simultaneous connections to the database server.
Best Practices for EJB3 Connection Pooling
1. Use a Reliable Connection Pooling Provider
Selecting a robust connection pooling provider is the cornerstone of efficient EJB3 connection pooling. While Java EE servers like WildFly, Payara, or GlassFish come with built-in connection pool implementations, it's essential to evaluate and choose the right provider based on your application's specific requirements. For instance, HikariCP and Apache DBCP are popular choices known for their performance and reliability.
2. Tune Connection Pool Size
Carefully tuning the connection pool size is vital for achieving optimal performance. Setting the pool size too low can lead to contention and performance degradation, especially in high-concurrency scenarios. On the other hand, an excessively large pool size may strain the database server and waste resources. Conduct thorough load testing to determine the ideal pool size for your application's workload.
Code Example: Tuning Connection Pool Size with HikariCP
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("username");
config.setPassword("password");
config.setMaximumPoolSize(10); // Set the maximum pool size
config.setMinimumIdle(2); // Set the minimum number of idle connections
HikariDataSource ds = new HikariDataSource(config);
In this example, the MaximumPoolSize
and MinimumIdle
parameters are crucial for fine-tuning the connection pool size with HikariCP, ensuring both performance and resource efficiency.
3. Leverage Connection Pool Validation
Enabling connection pool validation mechanisms can significantly enhance the reliability of database connections. By validating connections before handing them over to the application, potential issues such as stale connections or network failures can be detected early, preventing runtime errors and improving overall robustness.
4. Implement Connection Pool Timeout Handling
Integrating proper timeout handling in your connection pool configuration is essential for preventing deadlock situations and ensuring graceful degradation under heavy loads. Setting appropriate timeout values for acquiring and releasing connections helps maintain application responsiveness and prevents resource contention.
5. Utilize JPA Batch Processing and Fetch Size Optimization
When using Java Persistence API (JPA) for database interactions in EJB3 applications, leveraging batch processing and optimizing fetch size can significantly reduce the number of round trips to the database, improving overall performance. Proper utilization of batch processing and fetch size parameters in JPA can also complement connection pooling efforts by minimizing connection use and maximizing data retrieval efficiency.
Advanced Techniques for EJB3 Connection Pooling
Beyond the fundamental best practices, advanced techniques can further elevate the effectiveness of EJB3 connection pooling. Techniques such as connection leasing, connection partitioning, and dynamic pool resizing are valuable strategies for fine-tuning connection management based on dynamic workload requirements.
Final Considerations
Effective EJB3 connection pooling is indispensable for building high-performance, scalable enterprise Java applications. By adhering to best practices such as selecting a reliable pooling provider, fine-tuning pool size, leveraging validation and timeout handling, and optimizing database interactions, developers can ensure that their EJB3 applications interact with databases efficiently and reliably.
In summary, mastering EJB3 connection pooling best practices involves a combination of selecting the right tools, configuring optimal parameters, and adopting advanced strategies to cater to varying application demands. By embracing these best practices, developers can unlock the full potential of EJB3 in delivering robust, performant, and scalable enterprise applications.
For further reading, the Oracle documentation on Configuring Connection Pool Parameters offers a comprehensive resource for understanding and fine-tuning connection pooling in Java EE environments.