Optimizing Concurrent Job Execution in Spring and Quartz
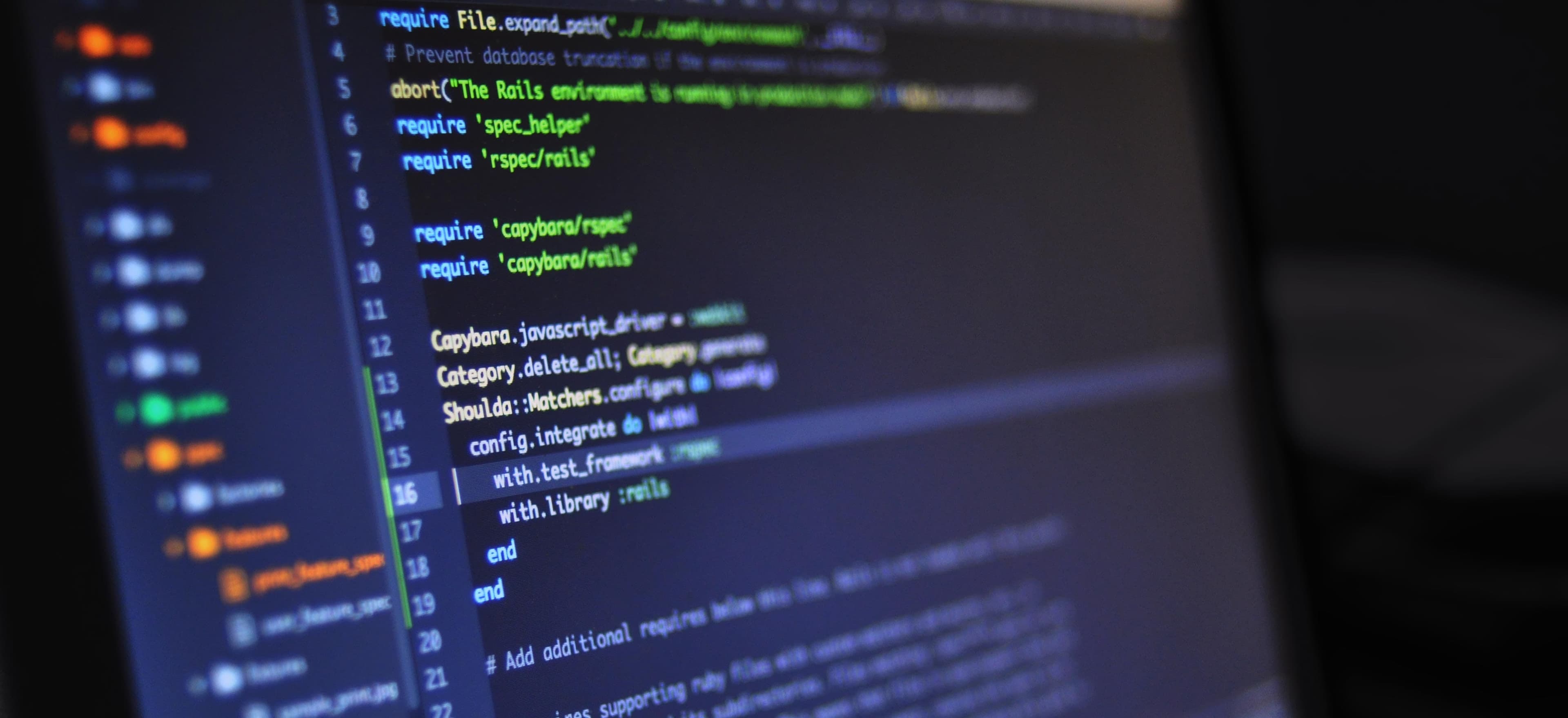
- Published on
Optimizing Concurrent Job Execution in Spring and Quartz
In modern web applications, the need for scheduling and executing concurrent jobs is becoming increasingly common. From processing data in the background to sending out notifications, there are numerous tasks that require efficient and reliable job execution. Luckily, frameworks like Spring and Quartz provide powerful tools for managing and executing scheduled jobs. In this blog post, we will delve into strategies for optimizing concurrent job execution using Spring and Quartz.
Understanding the Need for Optimization
Before we dive into optimizations, let's understand why it's important. In a typical application, there can be multiple jobs running concurrently. Without proper optimization, these jobs can contend for resources, leading to inefficiencies, resource exhaustion, and potential performance bottlenecks. Optimizing concurrent job execution ensures that jobs run efficiently, utilize resources effectively, and do not interfere with each other.
Leveraging Thread Pools in Quartz Scheduler
When it comes to concurrent job execution, properly configuring thread pools in Quartz Scheduler is vital. Quartz uses a set of worker threads to execute jobs. By default, it creates a new thread for each job, which can lead to resource contention and high thread creation overhead.
To optimize concurrent job execution in Quartz, we can configure a thread pool to control the number of concurrent jobs executing at any given time. This prevents resource exhaustion and provides better control over job execution. Here's an example of configuring a thread pool in Quartz using Spring:
@Bean
public SchedulerFactoryBean schedulerFactoryBean() {
SchedulerFactoryBean scheduler = new SchedulerFactoryBean();
scheduler.setTaskExecutor(taskExecutor());
// Other configurations
return scheduler;
}
@Bean
public TaskExecutor taskExecutor() {
ThreadPoolTaskExecutor taskExecutor = new ThreadPoolTaskExecutor();
taskExecutor.setCorePoolSize(10);
taskExecutor.setMaxPoolSize(20);
taskExecutor.setQueueCapacity(30);
taskExecutor.afterPropertiesSet();
return taskExecutor;
}
In this example, we configure a ThreadPoolTaskExecutor as the task executor for the Quartz Scheduler. We set the core pool size, maximum pool size, and queue capacity to control the number of concurrent jobs that can be executed.
Using Spring's @Async for Asynchronous Job Execution
Spring provides the @Async
annotation, which allows methods to be executed asynchronously in a separate thread. This can be beneficial for offloading long-running tasks from the main application thread, thereby optimizing concurrent job execution. By combining @Async
with Quartz Scheduler, we can achieve efficient concurrent job execution.
Here's an example of using @Async
with Quartz jobs in Spring:
@Service
public class MyJobService {
@Async
public void executeJob() {
// Job execution logic
}
}
In this example, the executeJob
method is annotated with @Async
, allowing it to be executed asynchronously. When used in conjunction with Quartz Scheduler, this can help optimize concurrent job execution by offloading tasks to separate threads, preventing the main thread from being blocked.
Implementing Job Data Persistence
In a scenario where multiple instances of an application are running, Quartz's default behavior of storing job data in memory may lead to inconsistencies and issues with concurrent job execution. To address this, it's essential to leverage job data persistence to ensure that job details and states are consistently maintained across multiple instances of the application.
By configuring Quartz to use a database for job data persistence, we can ensure that job details are synchronized and resilient to application restarts and failures. Spring provides seamless integration with Quartz and databases, making it straightforward to implement job data persistence.
Optimizing Job Scheduling and Triggers
Efficient scheduling and trigger configurations are crucial for optimizing concurrent job execution. When scheduling jobs, it's essential to consider factors such as job dependencies, execution frequency, and concurrent job limitations. With Quartz Scheduler, we can optimize job scheduling by defining dependencies between jobs, setting trigger priorities, and controlling concurrent execution.
JobDetail job1 = JobBuilder.newJob(Job1.class).withIdentity("job1").build();
JobDetail job2 = JobBuilder.newJob(Job2.class).withIdentity("job2").build();
Trigger trigger1 = TriggerBuilder.newTrigger().withIdentity("trigger1").startNow().withPriority(1).build();
Trigger trigger2 = TriggerBuilder.newTrigger().withIdentity("trigger2").startNow().withPriority(2).build();
scheduler.scheduleJob(job1, trigger1);
scheduler.scheduleJob(job2, trigger2);
In this example, we define two jobs (job1
and job2
) with corresponding triggers, setting different trigger priorities. By prioritizing triggers, we can influence the order in which jobs are executed and optimize concurrent job scheduling.
Monitoring and Logging
Optimizing concurrent job execution does not stop at configuration and scheduling. It's crucial to monitor job execution and log relevant information for troubleshooting and performance analysis. Spring and Quartz provide various monitoring and logging capabilities that can be utilized to track job execution, identify bottlenecks, and optimize performance.
When monitoring concurrent job execution, consider leveraging tools like Spring's Actuator for monitoring application health and Quartz's built-in logging capabilities for tracking job execution details.
The Last Word
Optimizing concurrent job execution in Spring and Quartz is essential for ensuring the efficiency, reliability, and performance of scheduled jobs in modern applications. By leveraging thread pools, using @Async
for asynchronous job execution, implementing job data persistence, optimizing job scheduling and triggers, and monitoring job execution, we can achieve efficient and robust concurrent job execution.
In conclusion, with proper optimization strategies and best practices, Spring and Quartz provide powerful tools for managing and executing concurrent jobs, ensuring that they run efficiently and reliably in a concurrent environment.
By following these optimization techniques, developers can enhance the concurrent job execution in their applications, leading to improved performance and better resource utilization.
Incorporate these optimization techniques and take your concurrent job execution to the next level with Spring and Quartz!
Remember, optimizing concurrent job execution is not a one-time task. It's an ongoing process that requires continuous evaluation, monitoring, and refinement to ensure that your application's scheduled jobs are running at their best.
Start optimizing your concurrent job execution today and unleash the full potential of your application's scheduled tasks through Spring and Quartz!