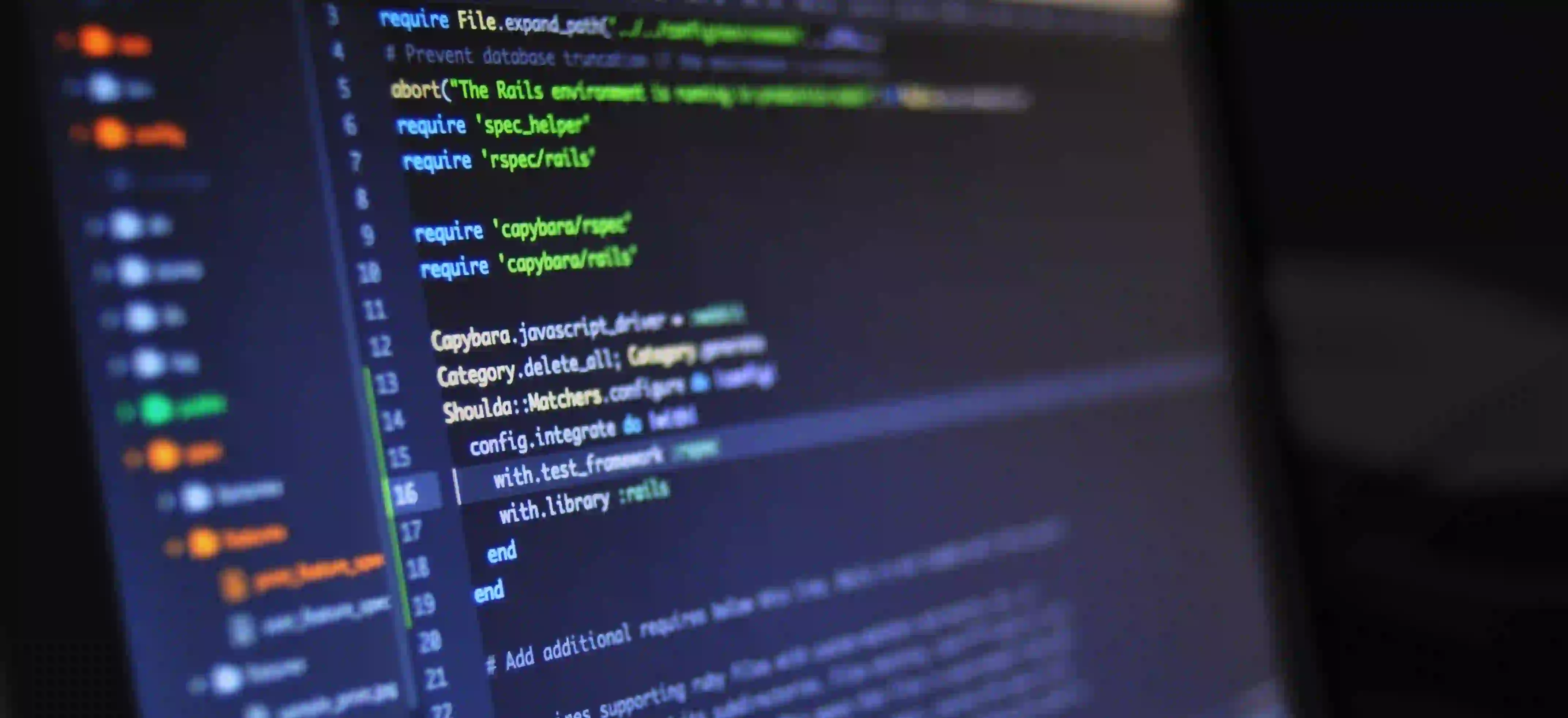
Form Validation in Angular 6: A Complete Guide
When developing web applications, form validation is an essential part of ensuring data integrity and a positive user experience. In this article, we will delve into form validation in Angular 6, exploring the tools and techniques available to validate user input effectively.
Why Form Validation is Crucial
Form validation is crucial as it helps in ensuring that the data submitted by users is accurate and secure. It also provides a way to give feedback to users about their input, thereby improving the overall user experience. In Angular applications, form validation can be achieved seamlessly using the framework’s built-in features and functionalities.
Setting Up a Basic Form in Angular 6
Before diving into form validation, let's start by setting up a basic form in an Angular 6 application.
// app.component.html
<form>
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" ngModel required>
</div>
<button type="submit">Submit</button>
</form>
In this basic form, we have a single input field for the username, with the ngModel
and required
directives provided by Angular for data binding and validation.
Template-Driven Form Validation
Angular 6 supports template-driven form validation, which allows us to add validation rules directly to the HTML template. Let's take a look at how we can perform validation on the username field.
Required Validation
The required
attribute in the input field ensures that the username is mandatory. If the user tries to submit the form without entering a username, an error message will be displayed.
Pattern Validation
We can also apply pattern validation to the input field to enforce specific input formats, such as a valid email address.
// app.component.html
<input type="text" id="email" name="email" ngModel required pattern="[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,}$">
In this example, the pattern
attribute specifies a regular expression for validating email addresses.
Reactive Form Validation
In addition to template-driven form validation, Angular 6 also provides support for reactive form validation, which is more suitable for complex scenarios and offers greater flexibility.
Setting Up a Reactive Form
To get started with reactive form validation, we need to import the necessary modules and set up the form group in the component class.
// app.component.ts
import { FormControl, FormGroup, Validators } from '@angular/forms';
// Inside the component class
userForm = new FormGroup({
username: new FormControl('', Validators.required),
email: new FormControl('', [Validators.required, Validators.email]),
});
In this example, we define a form group userForm
containing form controls for the username and email fields, along with their respective validation rules.
Using Validators
Angular provides a range of built-in validators such as Validators.required
and Validators.email
that can be applied to form controls to perform validation. Additionally, custom validators can be created to fulfill specific validation requirements.
// app.component.ts
function forbiddenNameValidator(nameRe: RegExp): ValidatorFn {
return (control: AbstractControl): ValidationErrors | null => {
const forbidden = nameRe.test(control.value);
return forbidden ? { forbiddenName: { value: control.value } } : null;
};
}
// Inside the form group definition
username: new FormControl('', [Validators.required, forbiddenNameValidator(/admin/)]),
In this example, we define a custom validator forbiddenNameValidator
to ensure that the username does not contain the word "admin". This illustrates the flexibility of reactive form validation in Angular.
Displaying Validation Errors
When it comes to providing feedback to users about form validation errors, Angular offers several options for displaying error messages in the UI.
Showing Validation Messages
We can use Angular's template syntax along with error properties to display validation messages next to the form fields.
// app.component.html
<div *ngIf="userForm.get('username').errors.required && userForm.get('username').touched">
Username is required
</div>
<div *ngIf="userForm.get('username').errors.forbiddenName">
Username cannot contain the word 'admin'
</div>
In this example, the *ngIf
directive is used to conditionally display error messages based on the form control's validation state.
Key Takeaways
Form validation is an integral part of building robust web applications, and Angular 6 provides powerful tools for implementing both template-driven and reactive form validation. By leveraging the frameworks' built-in features and flexibility, developers can ensure that user input is accurate and consistent, leading to a seamless user experience.
In conclusion, mastering form validation in Angular 6 empowers developers to create secure, user-friendly applications that meet the highest standards of data integrity and usability.
For more in-depth knowledge on Angular 6 form validation, you can refer to the official Angular documentation and Reactive Forms in Angular.
Start implementing form validation in your Angular 6 projects to deliver compelling user experiences with robust data integrity!