Deploying Docker Containers on AWS Fargate
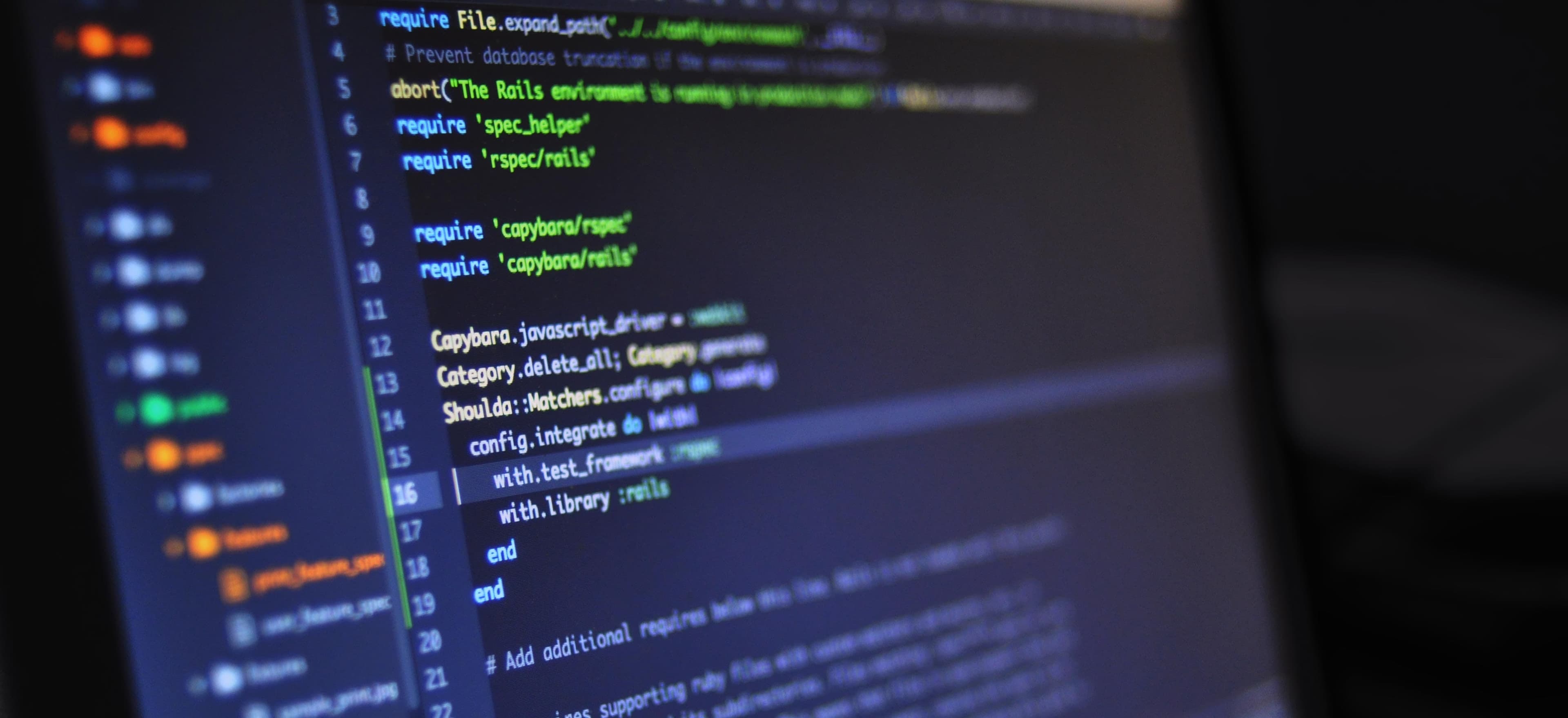
- Published on
Leveraging AWS Fargate to Deploy Docker Containers
In today's fast-paced and dynamic environment, deploying applications rapidly and efficiently has become essential. This is where AWS Fargate, a serverless compute engine for containers, comes into play. Fargate allows you to focus on designing and building applications without thinking about the infrastructure needed to run them. In this article, we will take a deep dive into using AWS Fargate to deploy Docker containers, exploring its key benefits and providing practical examples.
Understanding AWS Fargate
AWS Fargate is a compute engine that allows you to run containers without having to manage the underlying infrastructure. It is designed to work seamlessly with Amazon Elastic Container Service (ECS) and Amazon Elastic Kubernetes Service (EKS), making it an ideal choice for containerized workloads. By leveraging Fargate, you can concentrate on managing and scaling your applications without worrying about provisioning or configuring the infrastructure.
Prerequisites
Before getting started, you need to have an AWS account and the AWS Command Line Interface (CLI) installed and configured with proper access credentials. Additionally, you should have Docker installed locally to build and manage container images.
Building a Docker Image
Let's start by creating a simple web application using Spring Boot and containerizing it with Docker. Here's a basic example of a Spring Boot application that exposes a REST API endpoint:
@RestController
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, from AWS Fargate!";
}
}
We then create a Dockerfile to package the application into a container image:
FROM openjdk:11
COPY target/myapp.jar /app.jar
CMD ["java", "-jar", "/app.jar"]
After creating the Dockerfile, you can build the Docker image using the following command:
docker build -t myapp:latest .
Pushing the Docker Image to Amazon ECR
Amazon Elastic Container Registry (ECR) is a fully-managed Docker container registry that makes it easy to store, manage, and deploy Docker container images. We can push the Docker image we built to ECR by following these steps:
- Log in to your AWS account and navigate to the Amazon ECR console.
- Create a new repository for your Docker image.
- Follow the instructions to log in, tag the image, and push it to ECR.
Creating an ECS Task Definition
With the Docker image in ECR, the next step is to define an ECS task that specifies how the Docker container should run within Fargate. Here's an example of an ECS task definition in JSON format:
{
"family": "myapp-task",
"containerDefinitions": [
{
"name": "myapp-container",
"image": "your_account_id.dkr.ecr.your_region.amazonaws.com/myapp:latest",
"portMappings": [
{
"containerPort": 8080
}
]
}
],
"networkMode": "awsvpc",
"executionRoleArn": "arn:aws:iam::your_account_id:role/ecsTaskExecutionRole",
"cpu": "256",
"memory": "512"
}
In this task definition, we specify the Docker image's location in ECR, the container port to map, the networking mode, and the resource allocation for the task.
Running the ECS Task on Fargate
Now that we have the task definition, we can create an ECS service that will run our task on Fargate. Here's how to do it using the AWS CLI:
aws ecs create-cluster --cluster-name my-cluster
aws ecs create-service --cluster my-cluster --service-name myapp-service --task-definition myapp-task --launch-type FARGATE --network-configuration "awsvpcConfiguration={subnets=[your_subnets],securityGroups=[your_security_groups],assignPublicIp=ENABLED}"
Replace "your_cluster", "your_service", "your_task_definition", "your_subnets", and "your_security_groups" with your specific values.
Verifying the Deployment
Once the service is created, you can verify the deployment by accessing the public IP or DNS provided by the Fargate service, along with the mapped port (in this example, it's port 8080). You should see the "Hello, from AWS Fargate!" message returned by the Spring Boot application.
My Closing Thoughts on the Matter
In this article, we explored how to leverage AWS Fargate to deploy Docker containers, from building a Docker image and pushing it to ECR to defining an ECS task and running it on Fargate. By utilizing Fargate, you can focus on building and scaling your applications without the complexities of managing infrastructure. This serverless approach to container orchestration simplifies the deployment process and allows for greater agility and efficiency in delivering your applications.
Now that you have a foundational understanding of deploying Docker containers on AWS Fargate, you can further explore its capabilities and integrate it into your development and deployment workflows for seamless and scalable containerized applications.
Start your journey with AWS Fargate today and elevate your container deployment experience!