Building Scalable Web Applications with Spring MVC and AngularJS
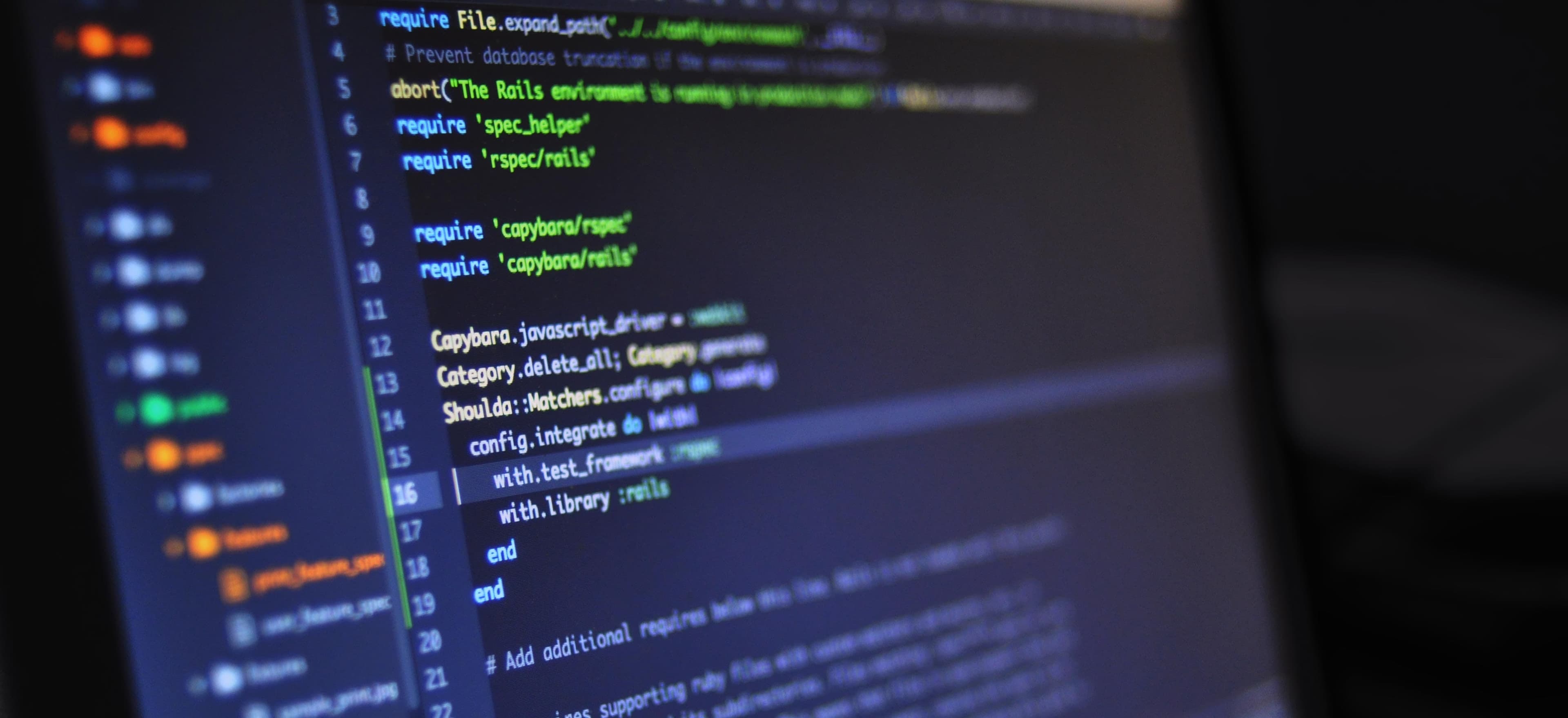
- Published on
Building Scalable Web Applications with Spring MVC and AngularJS
In today's fast-paced world, building scalable web applications is essential for businesses to meet the growing demands of users. To tackle this challenge, developers often turn to robust frameworks like Spring MVC and AngularJS to create efficient, scalable, and maintainable web applications. In this blog post, we'll explore how to leverage the power of Spring MVC and AngularJS to build scalable web applications that can handle a large number of users and data without sacrificing performance.
The Power of Spring MVC
Spring MVC is a well-established Java framework that provides a comprehensive model-view-controller architecture for building web applications. It offers features such as robust security, dependency injection, and easy integration with other Spring frameworks, making it a popular choice for enterprise-level applications.
Creating a Controller in Spring MVC
Let's start by creating a simple controller in Spring MVC that handles incoming HTTP requests. Below is an example of a basic controller that returns a "Hello, World!" message when a GET request is made to the '/hello' endpoint:
@Controller
public class HelloController {
@RequestMapping(value = "/hello", method = RequestMethod.GET)
@ResponseBody
public String helloWorld() {
return "Hello, World!";
}
}
In this example, we use the @Controller
annotation to mark the class as a Spring MVC controller. The @RequestMapping
annotation specifies the URL endpoint and HTTP method that this method should handle. The @ResponseBody
annotation tells Spring MVC to bind the return value of the method to the web response body.
By using Spring MVC's annotations, we can create clean, concise, and easily maintainable controllers that handle incoming requests efficiently.
AngularJS for Dynamic Front-End Development
AngularJS is a popular JavaScript framework for building dynamic and responsive single-page web applications. It provides features like two-way data binding, modular design, and dependency injection, making it an excellent choice for developing scalable front-end solutions.
Creating a Simple AngularJS Controller
Let's create a simple AngularJS controller that fetches a list of items from a backend API and displays them in the UI. The following code snippet demonstrates a basic AngularJS controller that makes an HTTP request to retrieve a list of items and assigns it to the scope for rendering:
angular.module('app', [])
.controller('ItemListController', function($http, $scope) {
$http.get('/api/items')
.then(function(response) {
$scope.items = response.data;
});
});
In this example, we define an AngularJS module named 'app' and create a controller called 'ItemListController'. Within the controller, we use the $http
service to make an HTTP GET request to '/api/items' and assign the response data to the $scope.items
variable for rendering in the UI.
By using AngularJS, we can create dynamic and interactive front-end components that seamlessly interact with backend services, providing users with a rich and engaging experience.
Integrating Spring MVC with AngularJS for Scalable Web Applications
Now that we have a basic understanding of Spring MVC and AngularJS, let's explore how to integrate the two frameworks to build scalable web applications.
RESTful API Development with Spring MVC
To create a scalable backend for our web application, we can leverage Spring MVC to develop RESTful APIs that provide data to the front-end. By following RESTful principles, we can design our APIs to be stateless, cacheable, and easily scalable, allowing for efficient communication between the client and the server.
Here's a simplified example of a Spring MVC controller that serves a list of items as JSON data for the AngularJS front-end:
@RestController
@RequestMapping("/api/items")
public class ItemController {
@Autowired
private ItemService itemService;
@GetMapping
public List<Item> getAllItems() {
return itemService.getAllItems();
}
}
In this example, we use the @RestController
annotation to indicate that this controller will handle RESTful requests and automatically serialize the return value to JSON. The @RequestMapping
annotation specifies the base endpoint for all handler methods in the controller. The @Autowired
annotation is used to inject an instance of the ItemService
into the controller for fetching the list of items.
By creating RESTful APIs with Spring MVC, we can ensure that our backend is scalable, flexible, and easily consumable by the AngularJS front-end.
Consuming RESTful APIs with AngularJS
With our RESTful APIs in place, we can now consume them in our AngularJS front-end to retrieve and display data to the user. AngularJS provides robust support for making HTTP requests and handling asynchronous operations, making it a breeze to connect to backend services.
Let's revisit our previous AngularJS controller and modify it to consume the '/api/items' endpoint to fetch and display a list of items:
angular.module('app', [])
.controller('ItemListController', function($http, $scope) {
$http.get('/api/items')
.then(function(response) {
$scope.items = response.data;
});
});
In this modified example, we make a GET request to '/api/items' to retrieve the list of items from our backend API and assign it to the $scope.items
variable for rendering.
By integrating Spring MVC with AngularJS, we can create scalable web applications that efficiently communicate and serve data between the backend and the front-end, resulting in a seamless user experience.
A Final Look
In this post, we've explored how to build scalable web applications with Spring MVC and AngularJS. By leveraging the power of Spring MVC's robust backend capabilities and AngularJS's dynamic front-end development features, developers can create efficient, scalable, and maintainable web applications that meet the demands of modern users.
To learn more about Spring MVC and AngularJS, check out the official documentation for Spring MVC and AngularJS. These resources provide in-depth information and best practices for building scalable web applications using these powerful frameworks.
Start building your next scalable web application with Spring MVC and AngularJS, and deliver a seamless and engaging user experience that can handle the ever-growing demands of the digital world.