Streamlining Delegate Method Generation with Macro Annotations
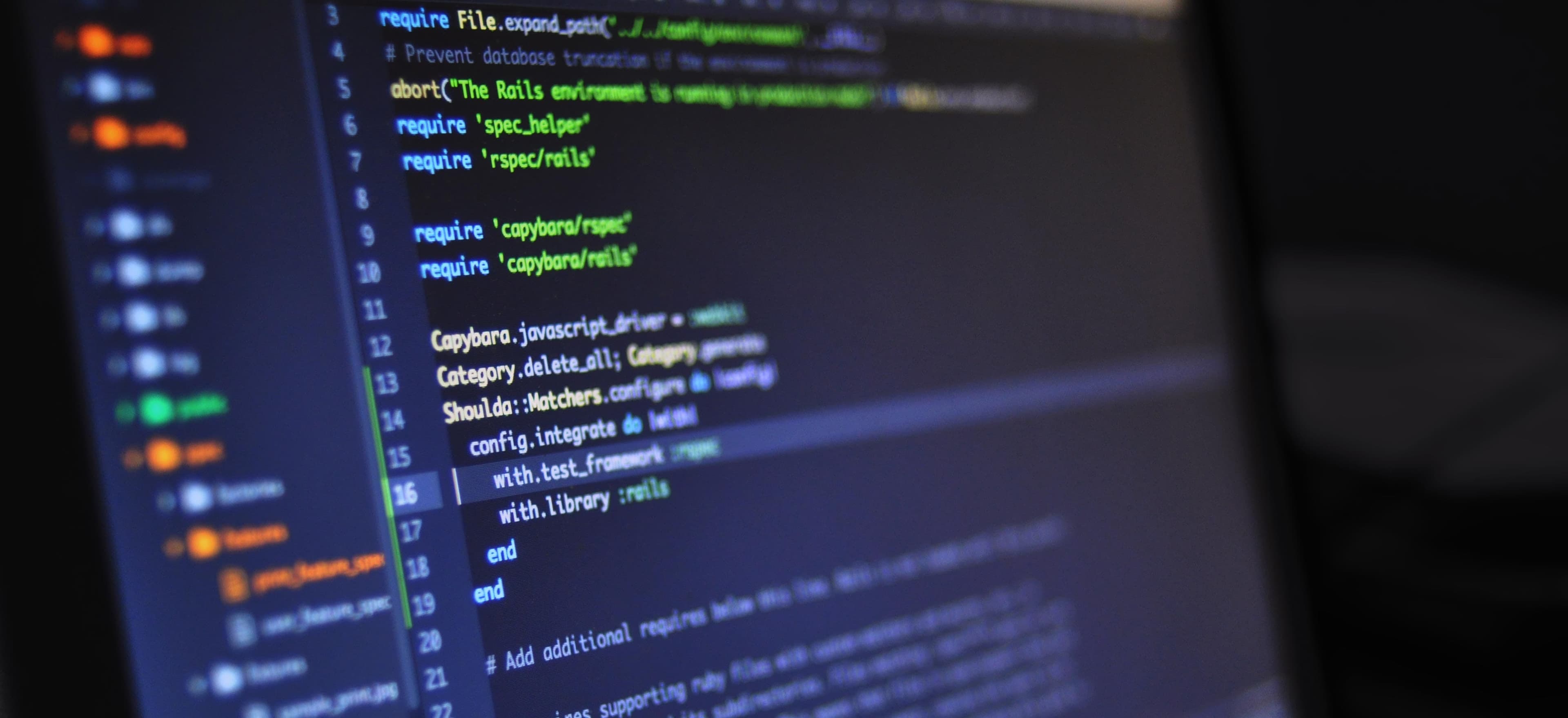
- Published on
Streamlining Java Delegate Method Generation with Macro Annotations
In the world of Java development, the generation of delegate methods for interfaces is a recurring task that often leads to boilerplate code. This inefficiency can be improved by leveraging the power of macro annotations. In this post, we will explore the concept of macro annotations, delve into their benefits, and demonstrate how they can be used to streamline the process of generating delegate methods in Java.
Understanding the Problem
Consider a scenario where we have an interface Vehicle
with several methods such as start
, stop
, and accelerate
. We then have a class Car
that implements this interface and delegates these methods to an instance of Engine
. This delegation requires us to write repetitive code for each method in the Car
class.
public interface Vehicle {
void start();
void stop();
void accelerate();
}
public class Car implements Vehicle {
private Engine engine;
// Delegate start method
public void start() {
engine.start();
}
// Delegate stop method
public void stop() {
engine.stop();
}
// Delegate accelerate method
public void accelerate() {
engine.accelerate();
}
}
As the number of methods in the Vehicle
interface grows, the amount of boilerplate code in the Car
class proportionally increases. This not only violates the DRY (Don’t Repeat Yourself) principle but also introduces potential errors when updating or adding new methods to the interface.
A Quick Look to Macro Annotations
Macro annotations are a feature that allows us to generate code during the compilation phase based on annotations placed on Java elements such as classes, methods, or fields. By using macro annotations, we can automate the generation of delegate methods, reducing the amount of boilerplate code and making the codebase more maintainable.
Implementing Macro Annotations for Delegate Method Generation
Let’s take a look at how we can use macro annotations to automate the generation of delegate methods for the Car
class.
Step 1: Define the Macro Annotation
We start by creating a custom macro annotation @DelegateMethods
. This annotation will be used to mark the fields that need delegate methods to be generated.
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.SOURCE)
@Target(ElementType.TYPE)
public @interface DelegateMethods {
}
The @Retention(RetentionPolicy.SOURCE)
annotation indicates that the @DelegateMethods
annotation should only be retained at the source level and will not be included in the compiled class files.
Step 2: Create the Processor for the Macro Annotation
We then create a processor that will process the @DelegateMethods
annotation and generate the delegate methods for the annotated class.
import java.util.Set;
import javax.annotation.processing.AbstractProcessor;
import javax.annotation.processing.RoundEnvironment;
import javax.lang.model.element.Element;
import javax.lang.model.element.TypeElement;
import javax.lang.model.type.DeclaredType;
import javax.lang.model.type.TypeMirror;
import javax.lang.model.util.Elements;
import javax.tools.Diagnostic;
public class DelegateMethodsProcessor extends AbstractProcessor {
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
for (Element element : roundEnv.getElementsAnnotatedWith(DelegateMethods.class)) {
if (element instanceof TypeElement) {
generateDelegateMethods((TypeElement) element);
} else {
processingEnv.getMessager().printMessage(Diagnostic.Kind.ERROR, "Only classes can be annotated with @DelegateMethods", element);
}
}
return true;
}
private void generateDelegateMethods(TypeElement element) {
Elements elementUtils = processingEnv.getElementUtils();
TypeMirror interfaceType = element.getInterfaces().get(0);
String interfaceName = interfaceType.toString();
// Generate delegate methods based on interface
// Implementation logic will go here
}
}
In the DelegateMethodsProcessor
class, we define the process
method to process the annotations and the generateDelegateMethods
method to generate the delegate methods based on the annotated interface.
Step 3: Implement the DelegateMethods Annotation Processor
Next, we need to implement the delegate methods generation logic within the generateDelegateMethods
method.
private void generateDelegateMethods(TypeElement element) {
Elements elementUtils = processingEnv.getElementUtils();
TypeMirror interfaceType = element.getInterfaces().get(0);
String interfaceName = interfaceType.toString();
for (Element enclosedElement : element.getEnclosedElements()) {
if (enclosedElement.getKind().isMethod()) {
String methodName = enclosedElement.getSimpleName().toString();
processingEnv.getMessager().printMessage(Diagnostic.Kind.NOTE, "Generating delegate method for: " + methodName);
// Generate delegate method
// Method generation logic will go here
}
}
}
Within the generateDelegateMethods
method, we iterate over the methods defined in the annotated interface and generate the corresponding delegate methods for the annotated class.
Step 4: Integration with Build Tool
Finally, we need to integrate the processor with the build tool (e.g., Maven or Gradle) by adding the necessary configuration to the build script.
For Maven, we would include the following plugin configuration:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
<configuration>
<annotationProcessors>
<annotationProcessor>com.example.DelegateMethodsProcessor</annotationProcessor>
</annotationProcessors>
</configuration>
</plugin>
</plugins>
</build>
For Gradle, we would include the following configuration:
dependencies {
annotationProcessor 'com.example:delegate-methods-processor:1.0.0'
}
Using the @DelegateMethods Annotation
With the setup in place, we can now apply the @DelegateMethods
annotation to our Car
class to automate the generation of delegate methods for the Vehicle
interface.
@DelegateMethods
public class Car implements Vehicle {
private Engine engine;
}
Upon compilation, the DelegateMethodsProcessor
will process the @DelegateMethods
annotation and generate the delegate methods for the Car
class.
My Closing Thoughts on the Matter
Macro annotations offer a powerful way to automate code generation in Java, reducing the need for boilerplate code and improving code maintainability. By using macro annotations, we can streamline the process of generating delegate methods, making our code more concise and easier to manage.
In this post, we explored the concept of macro annotations, implemented a custom macro annotation to automate the generation of delegate methods, and integrated the annotation processor with the build tool. We demonstrated how the @DelegateMethods
annotation can be used to streamline the process of generating delegate methods, ultimately improving the efficiency and maintainability of Java codebases.
By harnessing the capabilities of macro annotations, we can elevate our Java development practices and pave the way for more streamlined, efficient code generation processes.
For further reading and exploration on the topic of annotations and code generation in Java, consider checking out the following resources:
- Java Annotation Processing and Creating a Builder
- Understanding Java Custom Annotations
- Java Annotation Processors
With the knowledge gained from this post, you are now equipped to utilize macro annotations to streamline delegate method generation in your Java projects. Happy coding!
Checkout our other articles