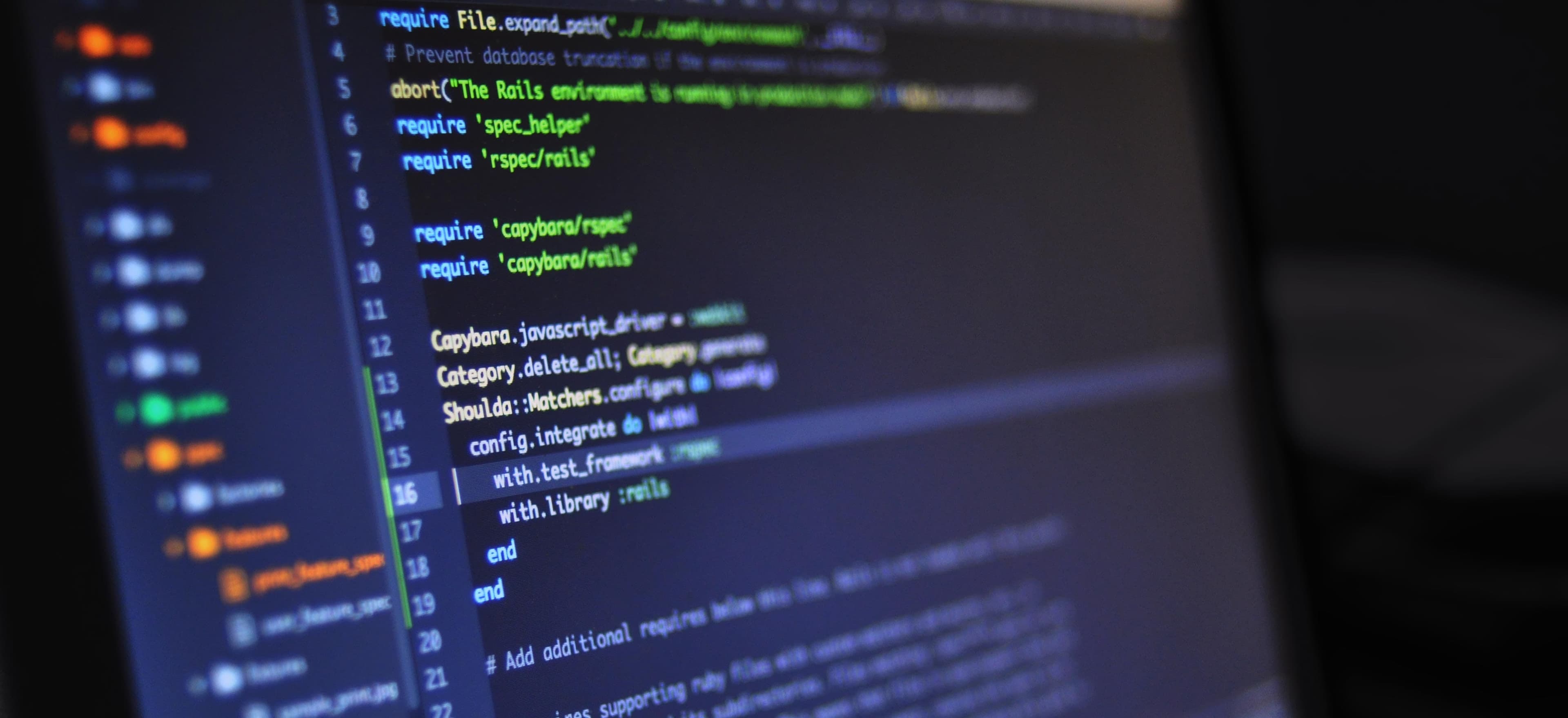
- Published on
Creating Impactful Architecture Diagrams
When it comes to developing software, having a well-designed architecture is crucial. It acts as a blueprint for the system, guiding developers and ensuring that the application is scalable, maintainable, and robust. One of the most effective ways to communicate a system's architecture is through architecture diagrams. These visual representations provide a high-level overview of the system's components and how they interact, making them essential for both technical and non-technical stakeholders.
In this article, we will explore the importance of creating impactful architecture diagrams and the best practices for doing so. We will also leverage the power of Java and various tools to generate these diagrams efficiently.
The Importance of Architecture Diagrams
Architecture diagrams serve several essential purposes in the software development process:
-
Communication: They act as a visual aid for communicating the system's design to both technical and non-technical stakeholders, enabling better understanding and alignment among team members.
-
Documentation: They serve as a form of documentation, capturing the system's structure, components, and interactions in a single, easy-to-understand visual representation.
-
Analysis: They help in analyzing the system's design, identifying potential flaws, and making informed decisions about improvements or optimizations.
-
Onboarding: They aid in onboarding new team members by providing a comprehensive view of the system's architecture, making it easier for them to grasp the system's design and functionality.
Given their importance, it's crucial to ensure that architecture diagrams are well-crafted, accurate, and easily understandable. This is where best practices for creating impactful architecture diagrams come into play.
Best Practices for Creating Impactful Architecture Diagrams
1. Understand Your Audience
Before creating an architecture diagram, it's essential to understand the audience that will be interpreting it. Different stakeholders may have different levels of technical expertise, so the level of detail and the choice of visual representation should align with their understanding.
For instance, a high-level overview may be suitable for non-technical stakeholders, while detailed component-level diagrams may be more appropriate for the development team.
2. Keep It Simple and Clear
Clarity is paramount when creating architecture diagrams. Use clear and standard notation to represent components, connections, and relationships. Avoid cluttering the diagram with excessive details that may hinder understanding. Remember, the goal is to communicate the essence of the architecture effectively.
3. Use Consistent Naming and Labeling
Consistency in naming and labeling components across diagrams and documentation is crucial for avoiding confusion. Use clear and meaningful names for components and ensure that the same names are used consistently throughout the documentation and codebase.
4. Leverage Tooling for Automation
While it's possible to create architecture diagrams manually, leveraging tools for automation can significantly improve the efficiency and accuracy of the process. In the Java ecosystem, several tools offer the ability to generate architecture diagrams from code, making use of annotations and configuration to extract the necessary information.
Generating Architecture Diagrams with Java
Java, being a prevalent language in enterprise software development, has a range of tools and libraries that facilitate the generation of architecture diagrams. Let's explore some of the popular tools and how they can be used to automate the creation of impactful architecture diagrams.
1. PlantUML
PlantUML is a popular open-source tool that uses a simple textual language to describe and generate various types of UML (Unified Modeling Language) diagrams, including component diagrams, class diagrams, and sequence diagrams.
Here's an example of using PlantUML to define a simple component diagram in Java:
public class PlantUMLDemo {
/**
* @startuml
* [Client] --> [REST Controller] : HTTP Request
* [REST Controller] --> [Service] : Process Request
* [Service] --> [Repository] : Data Access
* @enduml
*/
public void generateComponentDiagram() {
// Sample Java code with PlantUML annotations
}
}
In this example, the @startuml
and @enduml
annotations delineate the beginning and end of the PlantUML component diagram definition, using simple syntax to define the components and their relationships. This textual representation can then be processed by PlantUML to generate a visual component diagram.
Using PlantUML allows for the creation of architecture diagrams as code, which can be version-controlled and integrated into the development workflow seamlessly.
2. Javadoc
Javadoc is a widely used documentation tool for generating API documentation in HTML format from Java source code. While its primary purpose is to document APIs, it can also be leveraged to include architecture diagrams within the generated documentation.
By incorporating UML tags in Javadoc comments, developers can include UML diagrams directly in the code, which will then be processed by Javadoc to produce visual representations in the generated documentation.
Here's an example of using Javadoc to include a class diagram within Java code:
/**
* Represents a user entity in the system.
*
* @startuml
* class User {
* -id: int
* -username: String
* -email: String
* +getUserById(int id): User
* +saveUser(User user): void
* }
* @enduml
*/
public class User {
// Class code here
}
In this example, the @startuml
and @enduml
tags enclose the definition of the UML class diagram, including class attributes and methods. When Javadoc processes this code, it will incorporate the generated class diagram into the documentation.
By integrating architecture diagrams directly into the code using Javadoc, developers can ensure that the diagrams are always in sync with the codebase and readily available within the generated documentation.
3. Structurizr
Structurizr is a comprehensive tool that enables software teams to create architecture diagrams based on code and configuration. It allows for the creation of system context, container, component, and deployment diagrams, providing a holistic view of the system's architecture.
Using Structurizr involves defining the system's architecture using a dedicated DSL (Domain-Specific Language) or Java code, and then using the tool to generate visual representations of the architecture.
Here's a simplified example of defining a component diagram using Structurizr's DSL in Java:
Workspace workspace = new Workspace("My Software System", "This is a description of my software system.");
Model model = workspace.getModel();
SoftwareSystem softwareSystem = model.addSoftwareSystem("Software System", "Description");
Person user = model.addPerson("User", "A user of my software system.");
user.uses(softwareSystem, "Uses");
// Add components and their relationships
Component controller = softwareSystem.addContainer("Controller", "Description", "Spring MVC Controller");
Component service = softwareChanel.addContainer("Service", "Description", "Spring Service");
controller.uses(service, "Uses");
// Create a component diagram view and add it to the workspace
ComponentView componentView = viewSet.createComponentView(softwareSystem, "Components", "The component diagram for the software system.");
componentView.addAllComponents();
In this example, a Workspace
is created to contain the software system's architecture, and components and their relationships are added to the model. The ComponentView
is then used to create a visual representation of the components and their relationships.
Structurizr offers a powerful way to define and maintain architecture diagrams in code, enabling teams to keep the diagrams in sync with the evolving architecture and generate visual representations as needed.
Final Thoughts
Creating impactful architecture diagrams is essential for effective communication, documentation, and analysis of software systems. By following best practices and leveraging tools that enable the generation of architecture diagrams from Java code, teams can ensure that their architecture documentation remains up-to-date and aligned with the evolving system.
Remember, the goal of these diagrams is to provide valuable insights into the system's design and aid in decision-making, so investing in creating impactful architecture diagrams is a worthwhile endeavor for any software development team.