Improving Scalability of JAX-RS Applications
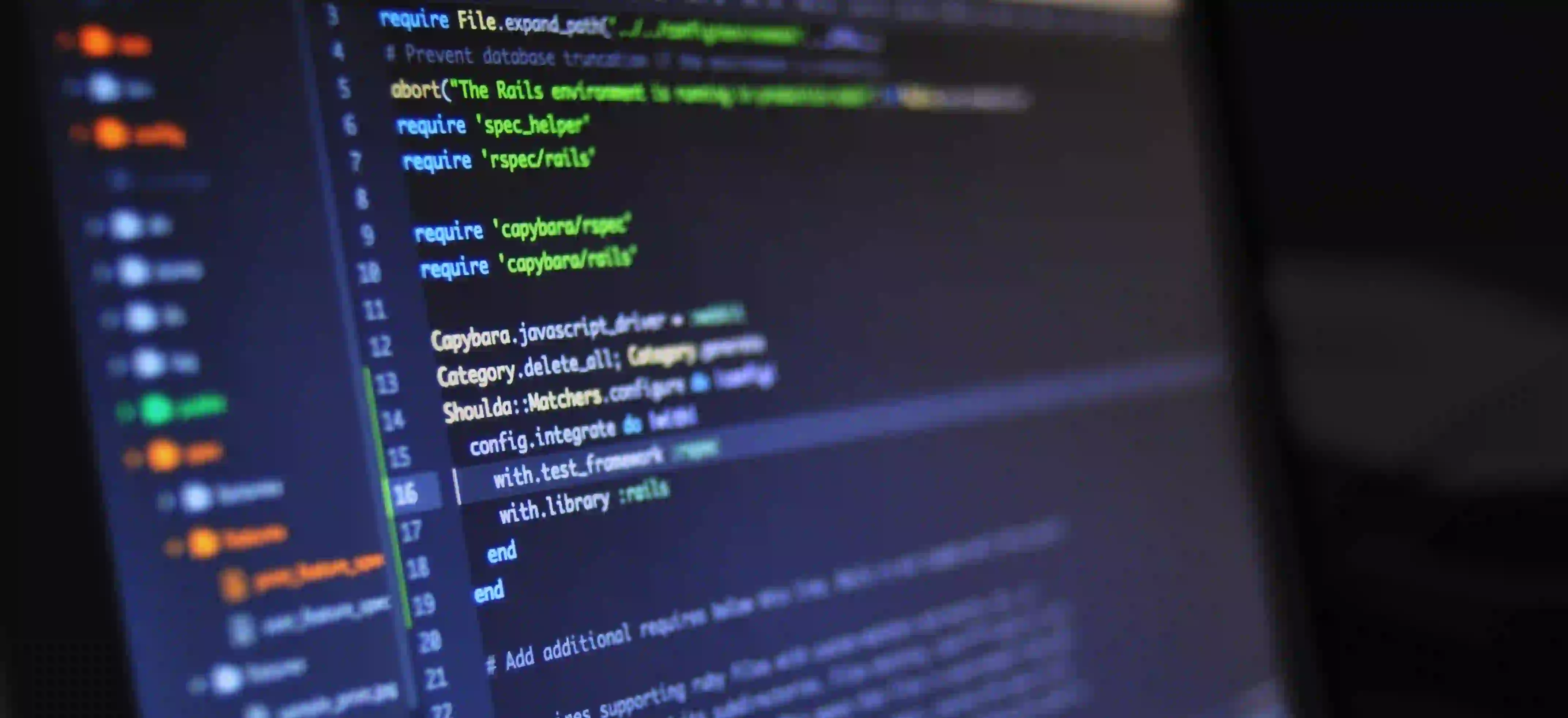
Improving Scalability of JAX-RS Applications
Scalability is a critical aspect of modern web applications, especially those built using Java. JAX-RS, the Java API for RESTful web services, is widely used for building web services and APIs. In this article, we'll explore key strategies to improve the scalability of JAX-RS applications, ensuring they can handle increasing loads and maintain high performance.
1. Efficient Resource Management
When designing a JAX-RS application, efficient resource management is crucial for scalability. Resources should be stateless wherever possible to allow them to be easily distributed across multiple servers. By avoiding server-side state, you enable requests to be load balanced across multiple instances without concern for session affinity.
@Path("/books")
@Produces("application/json")
@Consumes("application/json")
public class BookResource {
@GET
@Path("/{id}")
public Response getBook(@PathParam("id") int id) {
// Retrieve book from the database
// ...
return Response.ok(book).build();
}
}
In the above code snippet, the BookResource
is stateless, allowing it to scale horizontally across multiple server instances without the risk of inconsistent states between instances.
2. Asynchronous Processing
Utilizing asynchronous processing in JAX-RS can significantly improve the scalability of the application by allowing it to handle more concurrent requests. Java EE 7 introduced asynchronous support in JAX-RS, enabling resource methods to return CompletionStage
or Response
with a CompletionStage
entity, indicating that processing will be completed at a later time.
@GET
@Produces("application/json")
public void getAllBooks(@Suspended AsyncResponse asyncResponse) {
// Asynchronously fetch all books
CompletableFuture<List<Book>> future = CompletableFuture.supplyAsync(() -> bookService.getAllBooks());
future.thenAccept(asyncResponse::resume);
}
The above example demonstrates how the getAllBooks
method returns immediately after dispatching the asynchronous task, allowing the server to handle other requests while the long-running operation completes in the background, thus improving overall scalability.
3. Caching
Integrating caching mechanisms into JAX-RS applications can significantly reduce server load and improve scalability by serving frequently requested data from an in-memory cache. Resources that are not frequently updated are ideal candidates for caching, as they can reduce the load on the database and improve response times.
@GET
@Produces("application/json")
@Path("/popular-books")
public Response getPopularBooks() {
// Check if the data is available in the cache
List<Book> popularBooks = cache.get("popularBooks");
if (popularBooks == null) {
// If not in cache, fetch from the database and cache it
popularBooks = bookService.getPopularBooks();
cache.put("popularBooks", popularBooks);
}
return Response.ok(popularBooks).build();
}
In the above example, the getPopularBooks
method first checks the cache for the list of popular books. If the data is not present, it is fetched from the database and then stored in the cache for future requests.
4. Horizontal Scaling
Horizontal scaling involves adding more machines or nodes to the existing infrastructure to distribute the load. In a JAX-RS application, this can be achieved by deploying multiple instances of the application across different servers or using container orchestration platforms like Kubernetes to manage the scalability of the application.
public class Application extends ResourceConfig {
public Application() {
packages("com.example.resources");
}
}
When deploying JAX-RS applications to a containerization platform, such as Docker or Kubernetes, each instance of the application can be scaled independently to meet the changing demand. This approach allows for efficient utilization of resources and ensures continuous availability even during high loads.
Key Takeaways
Improving the scalability of JAX-RS applications is essential for ensuring they can handle increasing user loads and maintain high performance. By focusing on efficient resource management, asynchronous processing, caching, and horizontal scaling, developers can build robust and scalable JAX-RS applications that meet the demands of modern web services.
In summary, adhering to best practices and utilizing the available tools and techniques in JAX-RS allows developers to create scalable applications that can handle the challenges posed by growing user bases and increasing workloads.
Do you want to learn more about advanced JAX-RS concepts? Check out this comprehensive resource on JAX-RS 2.0 for further insights.