Understanding the Asynchronous EJB Communication
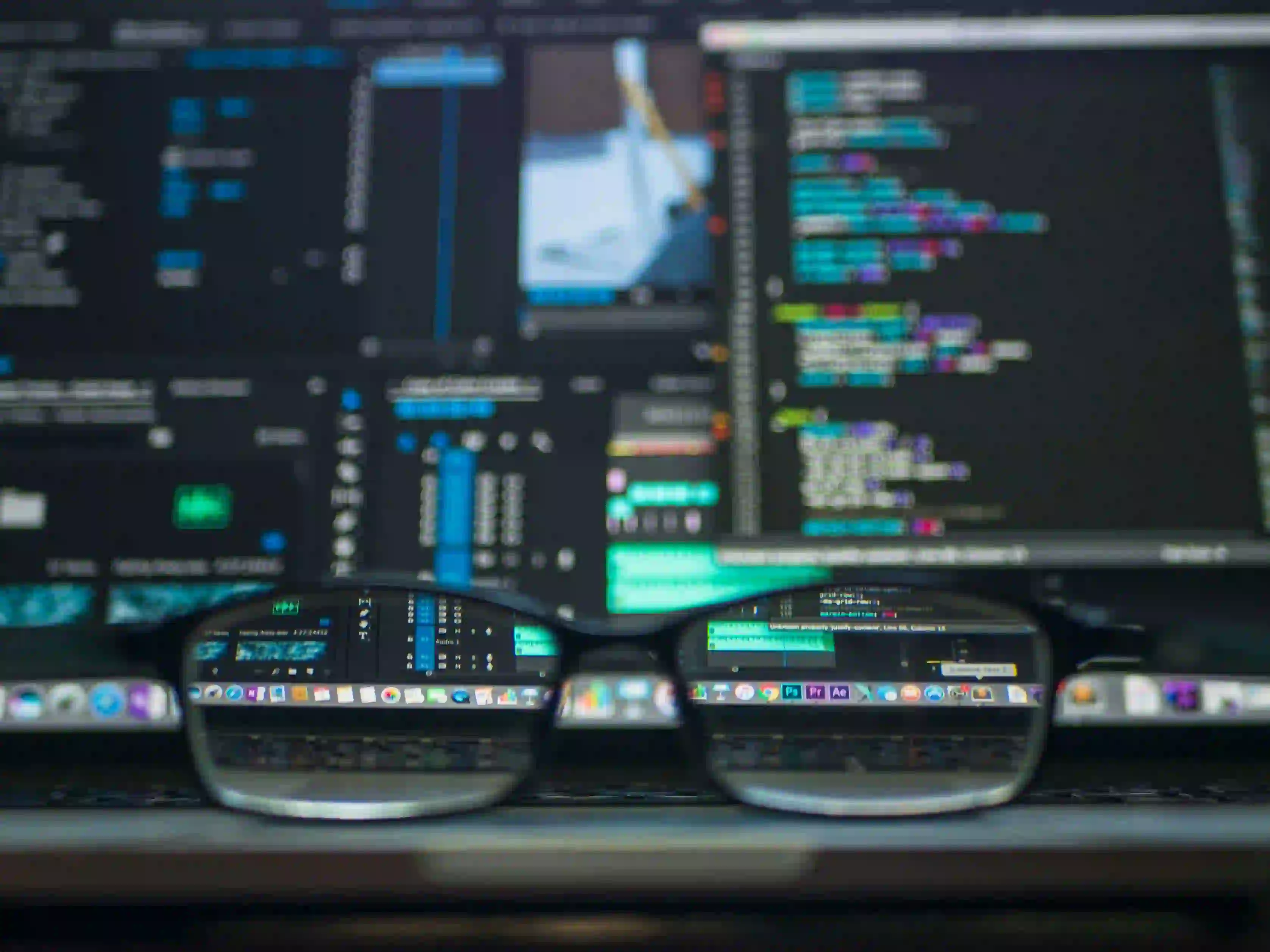
Understanding the Asynchronous EJB Communication
In Java EE, Enterprise JavaBeans (EJB) provide a powerful and efficient platform for building scalable, distributed applications. One of the key features of EJB is the ability to perform asynchronous communication, allowing for improved performance and responsiveness.
Asynchronous communication in EJB enables the server to process requests in a non-blocking manner, freeing up resources and improving overall system throughput. This blog post will explore the concept of asynchronous EJB communication, its benefits, and how to implement it in your Java applications.
What is Asynchronous Communication?
Asynchronous communication allows the sender to send a message without waiting for an immediate response. In the context of EJB, this means that a client can send a message to a bean and continue with other tasks without blocking and waiting for the result.
Asynchronous communication is particularly useful in scenarios where the operation may take a significant amount of time to complete, such as sending email notifications, processing large datasets, or performing scheduled tasks.
Benefits of Asynchronous Communication in EJB
Improved Performance
By using asynchronous communication, EJB can process requests in parallel, leading to improved performance and reduced response times. This is especially beneficial in high-traffic applications where responsiveness is crucial.
Resource Utilization
Asynchronous communication allows the server to handle a larger number of requests without blocking resources, leading to better resource utilization and overall system efficiency.
Enhanced Scalability
EJB's asynchronous communication capabilities enable applications to handle a larger number of concurrent users and perform resource-intensive tasks without impacting the overall application performance.
Implementing Asynchronous Communication in EJB
To implement asynchronous communication in EJB, you can use the @Asynchronous
annotation in combination with EJB methods. This annotation allows a method to be executed asynchronously, providing the benefits mentioned earlier.
Consider the following example:
@Stateless
public class EmailService {
@Asynchronous
public void sendEmail(String to, String subject, String body) {
// Code to send email
}
}
In this example, the sendEmail
method is annotated with @Asynchronous
, indicating that it can be executed asynchronously. When a client invokes this method, the EJB container will execute it in a separate thread, allowing the client to continue with other tasks without waiting for the email to be sent.
Exception Handling in Asynchronous EJB Methods
When working with asynchronous EJB methods, it's essential to handle exceptions properly to ensure robust error management. Since the client code does not block and wait for the method to complete, exceptions thrown during asynchronous execution need to be handled appropriately.
One way to handle exceptions in asynchronous EJB methods is by using the Future
interface. The Future
interface represents the result of an asynchronous computation and provides methods to check if the computation is complete and retrieve the result or handle any exception that occurred during execution.
Let's look at an example of using the Future
interface with asynchronous EJB methods:
@Stateless
public class DataProcessingService {
@Asynchronous
public Future<String> processLargeDataset(String dataset) {
// Process the dataset
try {
// Simulate processing time
Thread.sleep(5000);
return new AsyncResult<>("Processing completed");
} catch (InterruptedException e) {
return new AsyncResult<>("Processing interrupted");
}
}
}
In this example, the processLargeDataset
method returns a Future
object, allowing the client to retrieve the result or handle any exceptions that occurred during the asynchronous processing.
Asynchronous EJB and Transactions
When using asynchronous EJB methods, it's important to consider transaction management. By default, asynchronous methods do not inherit the client's transaction context, meaning they execute without any ongoing transaction. However, you can specify transaction attributes using annotations such as @TransactionAttribute
to control the transaction behavior of asynchronous EJB methods.
Here's an example of using @TransactionAttribute
with asynchronous EJB methods:
@Stateless
public class PaymentService {
@Asynchronous
@TransactionAttribute(TransactionAttributeType.REQUIRES_NEW)
public void processPayment(String accountId, BigDecimal amount) {
// Process the payment
}
}
In this example, the processPayment
method is annotated with @TransactionAttribute(TransactionAttributeType.REQUIRES_NEW)
, indicating that it should always run within a new transaction, independent of the client's transaction context.
The Bottom Line
Asynchronous communication in EJB is a powerful feature that enhances system performance, resource utilization, and scalability. By leveraging the @Asynchronous
annotation, developers can design responsive and efficient applications that can handle long-running and resource-intensive tasks without blocking the client.
Understanding how to implement asynchronous communication and effectively manage exceptions and transactions in EJB is crucial for building robust and efficient enterprise applications. By incorporating asynchronous EJB communication into your Java EE projects, you can optimize system performance and deliver a seamless user experience.
To delve deeper into EJB and asynchronous communication, you can explore the official Java EE documentation, which provides in-depth information and best practices for leveraging EJB's asynchronous capabilities.
In conclusion, mastering asynchronous EJB communication is a valuable skill for Java developers, enabling them to build high-performance, scalable, and responsive enterprise applications.
Remember, the key to successful asynchronous EJB communication lies in understanding its benefits, implementing it effectively, and managing exceptions and transactions with precision and care. Happy coding!