Analyzing S&P 500 Oil Stock Prices Using Apache Spark SQL
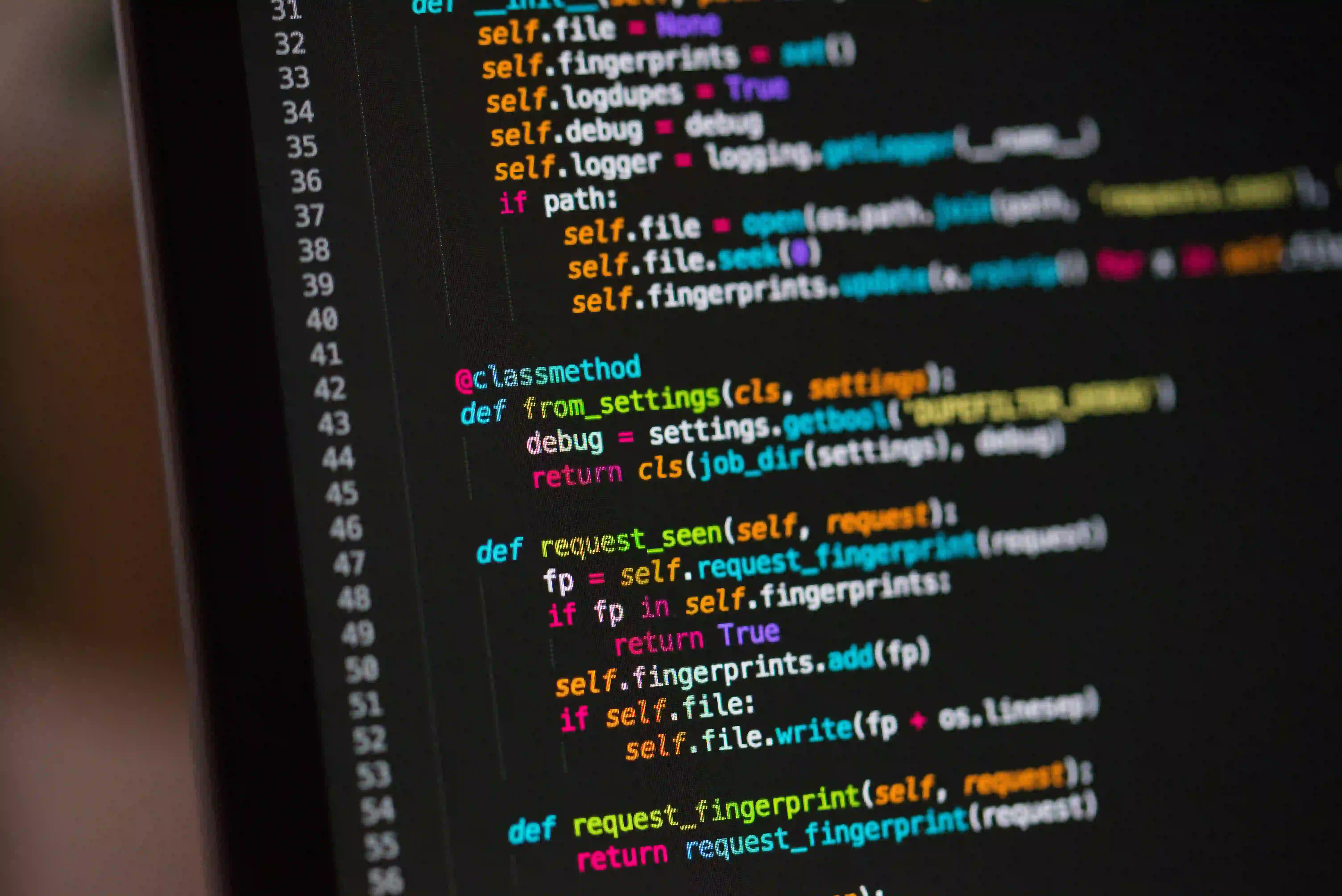
Analyzing S&P 500 Oil Stock Prices Using Apache Spark SQL
Analyzing stock prices is a crucial aspect of financial analysis, as it provides valuable insights for investment decisions. In this blog post, we will explore how to use Apache Spark SQL to analyze the stock prices of S&P 500 oil companies. Apache Spark SQL provides a powerful platform for processing large-scale datasets and running SQL queries across distributed data.
Setting Up the Environment
First, let's set up our environment by ensuring that we have Apache Spark installed. If you don't have it installed, you can follow the official Apache Spark installation guide. Additionally, we'll need access to the stock price data for S&P 500 oil companies. You can find this data on financial data providers such as Yahoo Finance or Quandl.
Once the environment is set up, we can proceed with loading the stock price data into Apache Spark and start analyzing it using Spark SQL.
Loading the Data
We'll start by loading the stock price data into a Spark DataFrame. For this example, let's assume that we have a CSV file containing the historical stock prices of S&P 500 oil companies. We can use the spark.read.csv
method to load the data into a DataFrame.
// Import necessary Spark classes
import org.apache.spark.sql.SparkSession;
// Create a Spark session
SparkSession spark = SparkSession.builder()
.appName("OilStockAnalysis")
.config("spark.some.config.option", "some-value")
.getOrCreate();
// Load the CSV data into a DataFrame
Dataset<Row> stockPrices = spark.read()
.option("header", "true")
.csv("s3://your-bucket-name/path/to/stock_prices.csv");
When working with large datasets, it's essential to utilize Apache Spark's distributed computing capabilities, which can efficiently handle massive amounts of data.
Exploring the Data
Once the data is loaded into the DataFrame, we can start exploring and analyzing it using Spark SQL. We can register the DataFrame as a temporary SQL view and then run SQL queries against it.
// Register the DataFrame as a SQL temporary view
stockPrices.createOrReplaceTempView("stock_prices");
// Run SQL queries using Spark SQL
Dataset<Row> result = spark.sql("SELECT * FROM stock_prices WHERE symbol = 'XOM' ORDER BY date DESC");
// Show the results
result.show();
In the above example, we're running a SQL query to retrieve the stock prices for the company with the symbol 'XOM' and ordering the results by date in descending order. This demonstrates the simplicity and familiarity of using SQL syntax to analyze data within Apache Spark.
Aggregating and Summarizing Data
One of the key benefits of using Spark SQL is the ability to perform aggregations and summarizations of large datasets. Let's calculate the average closing prices of oil stocks grouped by symbol.
Dataset<Row> avgClosingPrices = spark.sql("SELECT symbol, AVG(close) as avg_close FROM stock_prices GROUP BY symbol");
avgClosingPrices.show();
This query calculates the average closing price for each oil company and presents the results. Such aggregations are essential for gaining insights into the overall performance of different stocks within the oil industry.
Joining Data with External Datasets
In real-world scenarios, it's common to join the stock price data with external datasets to enrich the analysis. Let's join the stock price data with a dataset containing additional information about the S&P 500 oil companies, such as their market cap and sector.
// Load additional company data from a CSV file
Dataset<Row> companyData = spark.read()
.option("header", "true")
.csv("s3://your-bucket-name/path/to/company_data.csv");
companyData.createOrReplaceTempView("company_data");
// Join stock prices with company data
Dataset<Row> joinedData = spark.sql("SELECT sp.symbol, sp.close, cd.market_cap, cd.sector " +
"FROM stock_prices sp " +
"JOIN company_data cd ON sp.symbol = cd.symbol");
joinedData.show();
By joining the stock price data with additional company information, we can gain a more comprehensive understanding of the oil industry's stock performance.
Writing the Results
Once we have performed the analysis, it's important to store or write the results for further processing or reporting. We can write the results back to our preferred storage, such as Amazon S3 or HDFS.
// Write the joined data to a Parquet file
joinedData.write().parquet("s3://your-bucket-name/path/to/output/stock_analysis_results.parquet");
Writing the results allows us to preserve the analyzed data for future use or share it with other stakeholders.
The Bottom Line
In this blog post, we have explored how to use Apache Spark SQL for analyzing the stock prices of S&P 500 oil companies. We covered loading data, running SQL queries, aggregating data, joining with external datasets, and writing the results. Apache Spark SQL provides a powerful and familiar interface for analyzing large-scale financial datasets, making it an invaluable tool for financial analysis and decision-making.
By mastering Apache Spark SQL, financial analysts and data scientists can gain deeper insights into stock market trends and make informed investment decisions. With the ability to process vast amounts of data efficiently, Spark SQL is a game-changer for analyzing financial markets.
If you're interested in learning more about Apache Spark and its capabilities, check out the official Apache Spark documentation for in-depth resources and tutorials.