Troubleshooting RxPreferences Integration with Dagger
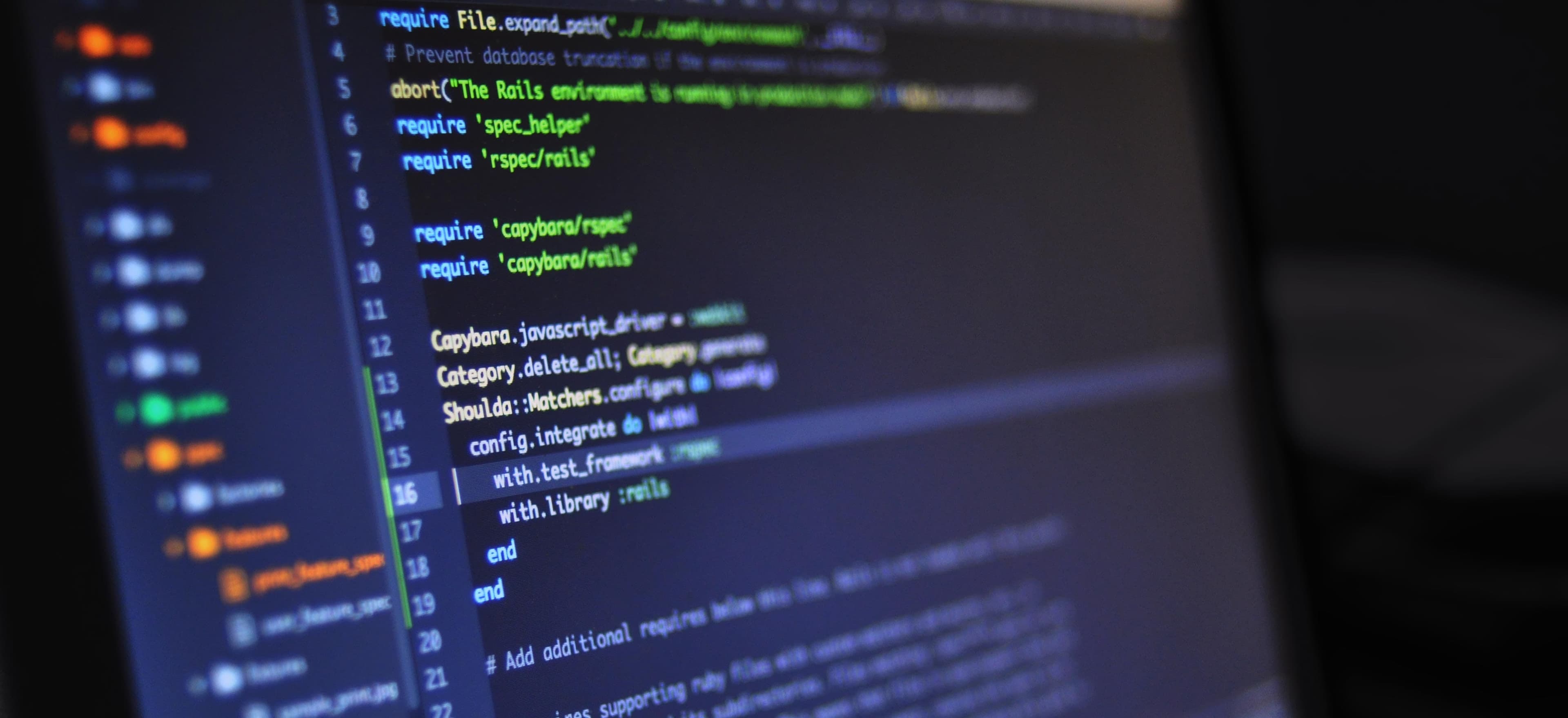
- Published on
Troubleshooting RxPreferences Integration with Dagger
RxPreferences is a powerful library that simplifies preference management in Android applications, especially when combined with RxJava's reactive programming model. Integrating RxPreferences with Dagger for dependency injection enhances scalability and testability. However, like any integration, there can be challenges. This blog post explores common issues encountered while using RxPreferences with Dagger and offers solutions to troubleshoot these problems.
Table of Contents
- What Are RxPreferences and Dagger?
- Common Integration Problems
- Dependencies Not Injected
- NullPointerExceptions
- Threading Issues
- Example Implementation
- Troubleshooting Tips
- Conclusion
What Are RxPreferences and Dagger?
RxPreferences
RxPreferences is a reactive approach to SharedPreferences in Android. It allows you to manage preferences using Observable
streams, which makes it easier to handle changes and updates throughout the application without tightly coupling components.
Dagger
Dagger is a popular dependency injection framework for Java and Android. It simplifies the process of providing dependencies to components and makes the code cleaner and more maintainable.
By combining RxPreferences with Dagger, we can easily manage preference states while keeping our components decoupled. However, challenges can arise during integration.
Common Integration Problems
Dependencies Not Injected
One of the common problems is when your Dagger components can't provide the RxPreferences dependencies properly. This can lead to unexpected null values in your code.
Solution:
- Ensure Proper Module Configuration: Make sure your Dagger Module is providing the RxPreferences instance correctly. Here’s an example:
@Module
public class PreferencesModule {
@Provides
@Singleton
public SharedPreferences provideSharedPreferences(Context context) {
return PreferenceManager.getDefaultSharedPreferences(context);
}
@Provides
@Singleton
public RxPreferences provideRxPreferences(SharedPreferences sharedPreferences) {
return RxPreferences.create(sharedPreferences);
}
}
In this code, we define a Dagger module that provides the SharedPreferences
and the RxPreferences
instance. Make sure that your application has the correct Context
injected.
- Check Your Component Setup: Ensure that your Dagger components are set up correctly to inject your dependencies:
@Singleton
@Component(modules = {PreferencesModule.class})
public interface AppComponent {
void inject(MyActivity myActivity);
}
NullPointerExceptions
Another common issue is facing NullPointerExceptions
when trying to use the injected RxPreferences. This typically happens due to a failure in the injection process.
Solution:
- Correct Injection Sequence: Ensure that you call the
inject()
function in the appropriate lifecycle method of your Activity or Fragment. For example:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
((MyApplication) getApplication()).getAppComponent().inject(this);
// Now it's safe to use the injected RxPreferences:
rxPreferences.getString("key", "default value").subscribe(value -> {
// Handle the received value
});
}
- Check the Lifecycle: Make sure that the
inject()
method is called before any other method that utilizes the injected variable.
Threading Issues
RxJava is asynchronous by nature, and combining it with Preferences could lead to threading issues, especially if synchronous calls manipulate the UI.
Solution:
- Schedulers: Make sure you are using appropriate Schedulers for your RxJava operations. Use
Schedulers.io()
for I/O operations andAndroidSchedulers.mainThread()
for working with UI components:
rxPreferences.getString("key", "default value")
.observeOn(AndroidSchedulers.mainThread())
.subscribeOn(Schedulers.io())
.subscribe(value -> {
// Update UI here
});
- Error Handling: Always handle errors using the
doOnError()
method or catching exceptions in your subscription. This helps in debugging:
rxPreferences.getString("key", "default value")
.observeOn(AndroidSchedulers.mainThread())
.subscribeOn(Schedulers.io())
.doOnError(e -> Log.e("Error", "Failed to retrieve preference", e))
.subscribe(value -> {
// Update UI here
});
Example Implementation
Let’s put everything together in a simple example. Below is a full working example of an Android Activity using Dagger and RxPreferences.
Build Gradle Dependencies
Make sure you have added dependencies for Dagger, RxPreferences, and RxJava in your build.gradle
file:
dependencies {
implementation "com.google.dagger:dagger:2.x"
implementation "com.google.dagger:dagger-compiler:2.x"
implementation "com.jakewharton.rxbinding2:rxbinding:2.x"
implementation "com.github.dbeattie.RxPreferences:rxpreferences:x.x"
}
Application Class
Set up your application class to initialize Dagger:
public class MyApplication extends Application {
private AppComponent appComponent;
@Override
public void onCreate() {
super.onCreate();
appComponent = DaggerAppComponent.builder()
.preferencesModule(new PreferencesModule())
.build();
}
public AppComponent getAppComponent() {
return appComponent;
}
}
Main Activity
Here is how you can use RxPreferences and Dagger in your Main Activity:
public class MainActivity extends AppCompatActivity {
@Inject
RxPreferences rxPreferences;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
((MyApplication) getApplication()).getAppComponent().inject(this);
// Save a preference
rxPreferences.getString("key", "default value")
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(value -> {
// Use the value
}, throwable -> {
// Handle error
Log.e("MainActivity", "Error fetching preference", throwable);
});
}
}
Troubleshooting Tips
- Check Logs: Always review logs for Dagger and RxJava-related issues. They can often provide direct hints about what's wrong.
- Ensure Correct Scopes: Make sure the scopes (like Singleton) are appropriately used to avoid lifecycle issues.
- Debug With Breakpoints: Utilize breakpoints in your IDE’s debugger to watch the flow of the injection process.
- Test Components Individually: Write unit tests for your Dagger modules and RxFunctions to isolate and identify issues.
To Wrap Things Up
Integrating RxPreferences with Dagger offers a powerful pattern for managing preferences reactively in Android applications. While it can introduce complexities, understanding common pitfalls and their solutions can significantly ease the development process. By following the guidelines above and applying best practices, you can make your integration robust and reliable.
If you are looking for further reading on the topic, consider checking out the official Dagger documentation and RxJava documentation. Happy coding!
Checkout our other articles