Overcoming Challenges in Java DSL Implementation
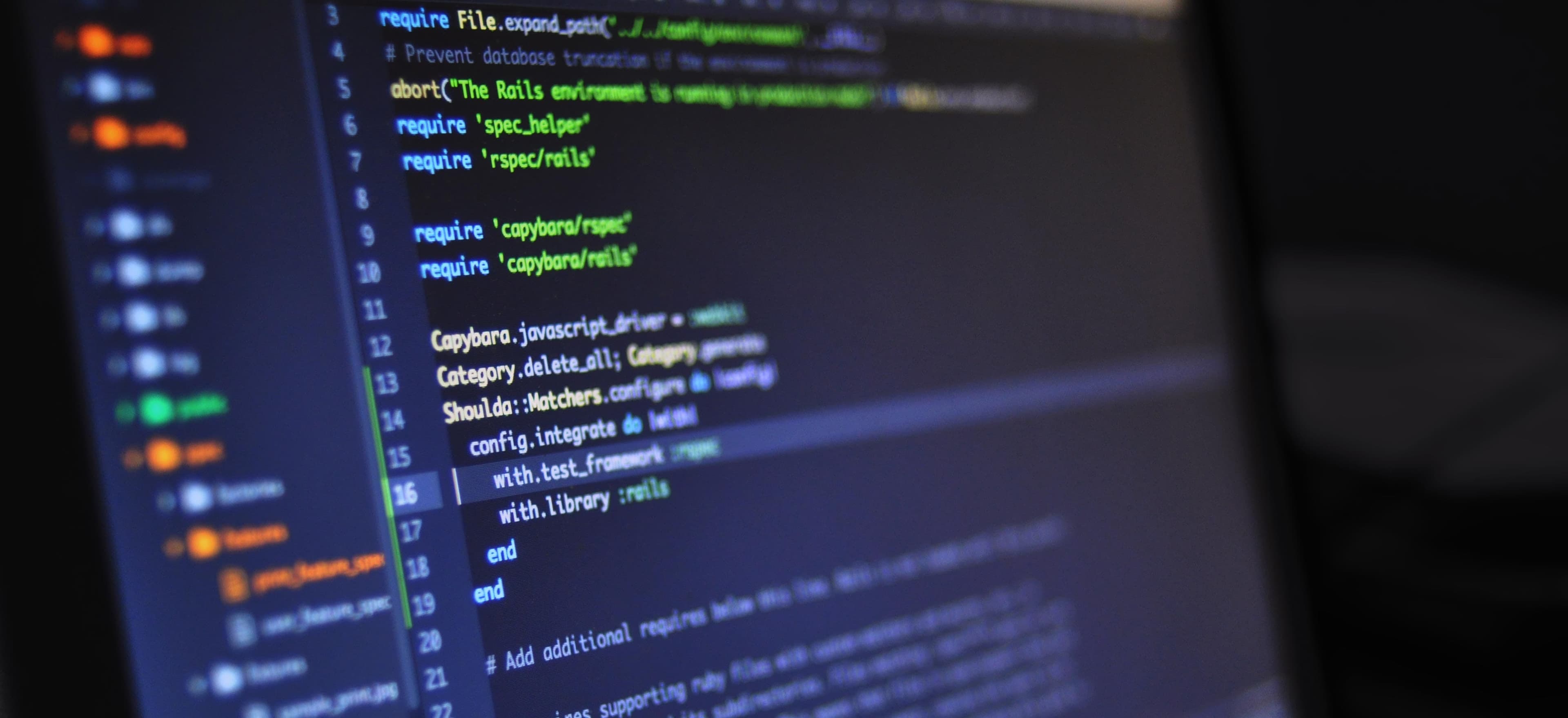
- Published on
Overcoming Challenges in Java DSL Implementation
Domain-Specific Languages (DSLs) are powerful tools that allow developers to create tailored languages specific to a particular problem domain. Implementing a DSL in Java can offer significant advantages, including enhanced readability, maintainability, and usability. However, several challenges arise during the implementation process. This blog post will explore these hurdles and provide strategies to overcome them, along with code examples to illustrate best practices.
Understanding DSLs
Before diving into challenges, let's establish what we mean by DSL. A DSL is a programming language designed for a specific set of tasks, offering constructs that a general-purpose language does not. While general-purpose languages like Java, Python, or C++ serve broad applications, DSLs focus on a limited area, aiming to simplify coding for that domain.
For instance, SQL is a DSL for database queries, whereas CSS is a DSL for styling web pages. In Java, creating a DSL could facilitate tasks in fields like data processing, configuration management, or even business rules.
When Implementing DSLs in Java, Consider:
- Internal vs. External DSLs: An internal DSL leverages the syntax of an existing language, while an external DSL has its unique syntax.
- Parsing: Handling the conversion of DSL code into executable Java code.
- Error Handling: Providing meaningful error messages to end-users.
- IDE Support: Ensuring compatibility with Integrated Development Environments (IDEs) for syntax highlighting and other development benefits.
Common Challenges Facing Java DSL Implementation
1. Designing the Language Syntax
When creating a DSL, one of the first steps is designing the syntax—how users will write code in your DSL. Keeping it intuitive while powerful is a challenge.
Solution
Use fluent interfaces to design an easily readable and writeable DSL. Fluent interfaces allow for method chaining, which mirrors natural language and enhances readability.
public class ReportBuilder {
private StringBuilder report = new StringBuilder();
public ReportBuilder title(String title) {
report.append("Title: ").append(title).append("\n");
return this;
}
public ReportBuilder addSection(String section) {
report.append("Section: ").append(section).append("\n");
return this;
}
public String build() {
return report.toString();
}
}
// Usage
String report = new ReportBuilder()
.title("Monthly Report")
.addSection("Sales Overview")
.addSection("Market Analysis")
.build();
Here, the ReportBuilder
class provides a fluent interface that makes constructing reports straightforward. Users can chain methods for clarity and improved expressiveness while coding.
2. Creating a Robust Parsing Mechanism
A pivotal aspect of developing a DSL is parsing—the transformation of DSL expressions into Java constructs. The disparity between the DSL syntax and Java can introduce complexity.
Solution
Utilize existing libraries such as ANTLR or JavaCC to help you build a parser. These libraries simplify the parsing process, allowing you to focus on your DSL's specific features.
Example
Here's a simple example using ANTLR to parse a simple expression language.
- Define a Grammar:
grammar Expr;
expr : expr ('+'|'-') expr
| expr ('*'|'/') expr
| INT
;
INT : [0-9]+ ;
WS : [ \t\r\n]+ -> skip ;
This grammar defines basic arithmetic expressions. RUN ANTLR on this grammar to generate the parser and lexer.
- Implement the Visitor:
Using the visitor pattern, you can evaluate the parsed expressions:
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.tree.*;
public class EvalVisitor extends ExprBaseVisitor<Integer> {
@Override
public Integer visitAddSub(ExprParser.AddSubContext ctx) {
Integer left = visit(ctx.expr(0));
Integer right = visit(ctx.expr(1));
return ctx.op.getType() == ExprParser.ADD ? left + right : left - right;
}
@Override
public Integer visitMulDiv(ExprParser.MulDivContext ctx) {
Integer left = visit(ctx.expr(0));
Integer right = visit(ctx.expr(1));
return ctx.op.getType() == ExprParser.MUL ? left * right : left / right;
}
@Override
public Integer visitInt(ExprParser.IntContext ctx) {
return Integer.valueOf(ctx.INT().getText());
}
}
3. Providing Error Feedback
A compelling feature of any DSL is meaningful error messages. Users should understand what went wrong and why, especially if they encounter errors during development.
Solution
Build a robust error-handling mechanism that captures and reports syntax errors. You can leverage ANTLR’s error mechanisms for syntax feedback, but you can also build custom error messages for semantics.
@Override
public Integer visitAddSub(ExprParser.AddSubContext ctx) {
try {
Integer left = visit(ctx.expr(0));
Integer right = visit(ctx.expr(1));
return ctx.op.getType() == ExprParser.ADD ? left + right : left - right;
} catch (Exception e) {
System.err.println("Error parsing expression: " + e.getMessage());
return null; // or an appropriate error value
}
}
In this example, we capture exceptions that may occur while evaluating an expression, providing the user with a helpful error message.
4. IDE and Tooling Support
While you may have designed a powerful DSL, if it doesn’t have adequate tooling (like syntax highlighting or auto-completion), developers may face a steep learning curve.
Solution
Use existing libraries or tools to generate IDE plugins. Tools like JetBrains MPS or Xtext can greatly simplify this process. For example, Xtext can allow you to create DSLs with rich IDE tooling support like syntax highlighting and refactoring.
5. Performance Considerations
Finally, performance can be a consideration. The additional overhead of a DSL may lead to performance risks that can impact application behavior.
Solution
Once you have a working DSL, profile its performance. Utilize Java profiling tools such as VisualVM or YourKit to identify bottlenecks in your implementation. Add optimizations where necessary, ensuring that the language remains effective and responsive.
Lessons Learned
Implementing a DSL in Java can bring about numerous advantages for developers and organizations alike. With careful consideration of the challenges—such as syntax design, parsing mechanisms, error handling, tooling support, and performance considerations—companies can leverage DSLs effectively.
Whether you're building a DSL for a specific domain or to target unique business problems, overcoming these challenges can lead you towards success. By utilizing Java's robustness, you can create a tailored language that enhances the productivity and satisfaction of your developers.
For more information on DSL design principles, consider checking out resources such as Martin Fowler's Domain-Specific Languages.
Feel free to share your thoughts or experiences with Java DSL implementation in the comments below! Have you faced any unique challenges? What strategies did you find helpful?
Checkout our other articles