Maximizing Efficiency: The Pitfalls of Thread Pool Misconfigurations
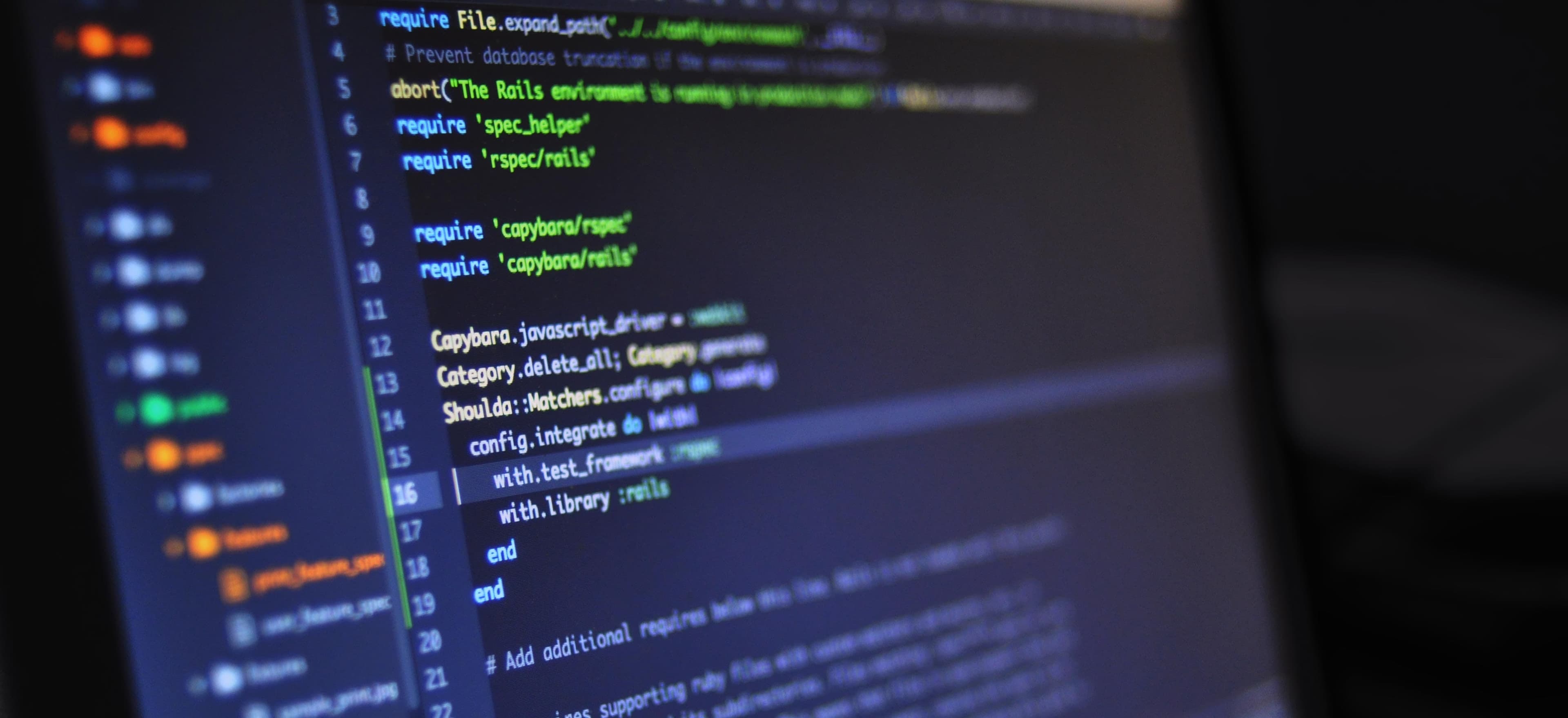
- Published on
Maximizing Efficiency: The Pitfalls of Thread Pool Misconfigurations
In the world of concurrent programming, thread pools are a crucial feature in managing task execution efficiently. However, misunderstandings and misconfigurations related to thread pools can lead to suboptimal performance and even application failures. In this blog post, we will dissect the importance of thread pools in Java, delve into common misconfigurations, and provide you with practical guidelines to maximize efficiency.
What is a Thread Pool?
A thread pool is a collection of threads that can be reused to perform multiple tasks. Instead of creating a new thread for every task, a thread pool creates a fixed number of threads and provides them for executing tasks on demand. This reduces the overhead of thread creation and destruction, allowing systems to handle more concurrent tasks more efficiently.
In Java, the ExecutorService
interface and its implementations such as ThreadPoolExecutor
are predominantly used to manage thread pools.
Benefits of Using Thread Pools
- Resource Management: Prevents resource exhaustion by limiting the number of concurrent threads.
- Performance Optimization: Reduces the overhead associated with thread creation.
- Task Reusability: Threads can be reused to execute multiple tasks, improving response times.
Common Misconfigurations
While using thread pools simplifies concurrency management, improper configuration can lead to various performance bottlenecks. Let's explore some frequent pitfalls and how to avoid them.
1. Inadequate Core Pool Size
Setting a core pool size that is too low will lead to thread starvation. When the workload spikes, the thread pool will struggle to handle the incoming tasks, leading to increased latency. On the other hand, an excessively high core pool size can lead to oversubscription of CPU resources.
ExecutorService executor = Executors.newFixedThreadPool(4);
Why this matters:
Choosing the right core pool size is essential for balancing load. A good starting point is the number of available processors, accessible through Runtime.getRuntime().availableProcessors()
.
2. Incorrect Maximum Pool Size
The maximum pool size is another critical parameter. Setting it too low can create bottlenecks under heavy loads, while a high value may lead to increased memory consumption and context switching overhead.
ThreadPoolExecutor executor = new ThreadPoolExecutor(
4, // Core pool size
10, // Max pool size
60, // Keep alive time
TimeUnit.SECONDS,
new LinkedBlockingQueue<Runnable>()
);
Best Practice: Monitor the workload and respond to patterns. For CPU-intensive tasks, keep the maximum size close to the number of available processors. For I/O-bound tasks, you may consider higher values.
3. Using Synchronous Queue without Capacity
Using a SynchronousQueue
without setting a bounded capacity can cause an application to block if all threads in the pool are busy, as it requires a producer-consumer relationship before allowing a task to proceed.
ThreadPoolExecutor executor = new ThreadPoolExecutor(
4,
10,
60,
TimeUnit.SECONDS,
new SynchronousQueue<Runnable>() // This can lead to deadlocks
);
Recommendation: Utilize bounded queues like LinkedBlockingQueue
to decouple tasks from immediate execution, allowing your application to handle burst workloads effectively.
4. Neglecting Keep-Alive Time
The keep-alive time determines how long excess idle threads are kept alive in the pool. If this time is too short, threads may frequently be terminated and recreated, generating unnecessary overhead.
ThreadPoolExecutor executor = new ThreadPoolExecutor(
4,
10,
10, // Too short keep-alive time
TimeUnit.SECONDS,
new LinkedBlockingQueue<Runnable>()
);
Guideline: Investigate your workload's nature. Longer keep-alive times may be more suited for applications with bursty workloads.
5. Ignoring Exception Handling
Thread pool tasks can throw unchecked exceptions that, if not handled, can terminate the executing thread. Not only can this create silent failures, but it can also lead to resource leaks.
executor.submit(() -> {
try {
// Task execution
} catch (Exception ex) {
// log exceptions
}
});
Catch-All: Always ensure adequate exception handling in tasks. This prevents catastrophic thread failures from disrupting your application.
Configuration Tips for Different Workloads
For CPU-Intensive Tasks
- Set the core pool size to the number of available processors.
- Set the maximum pool size to the same as the core pool size or slightly higher, depending on the workload's nature.
For I/O-Bound Tasks
- A higher core pool size is acceptable, often 2-4 times the number of available processors.
- Allow some leeway in the maximum pool size to handle high concurrency, adjusting based on performance findings.
Monitor and Adjust
Implement monitoring tools like Java VisualVM or JConsole to analyze your thread pools in real-time. Adjust pool sizes based on observed workload patterns.
Closing the Chapter
Thread pools are an invaluable tool in Java for managing concurrent tasks effectively. However, the small details of their configuration play a significant role in overall application performance. By understanding the pitfalls of thread pool misconfigurations and implementing the right strategies, you can maximize the efficiency of your Java applications.
Don't forget that tuning your thread pool isn’t a one-time task; it’s an ongoing process driven by careful observation of system performance as workloads evolve. For more insights on concurrency in Java, check out Java Concurrency in Practice by Brian Goetz, which serves as an excellent resource.
As you set out to configure and optimize your thread pools, keep these considerations in mind. The efficiency you achieve will not only enhance user experience but will also contribute to the robustness of your application during peak loads. Happy coding!
Checkout our other articles