Why Your Throwable in Java Could Cause Runtime Headaches
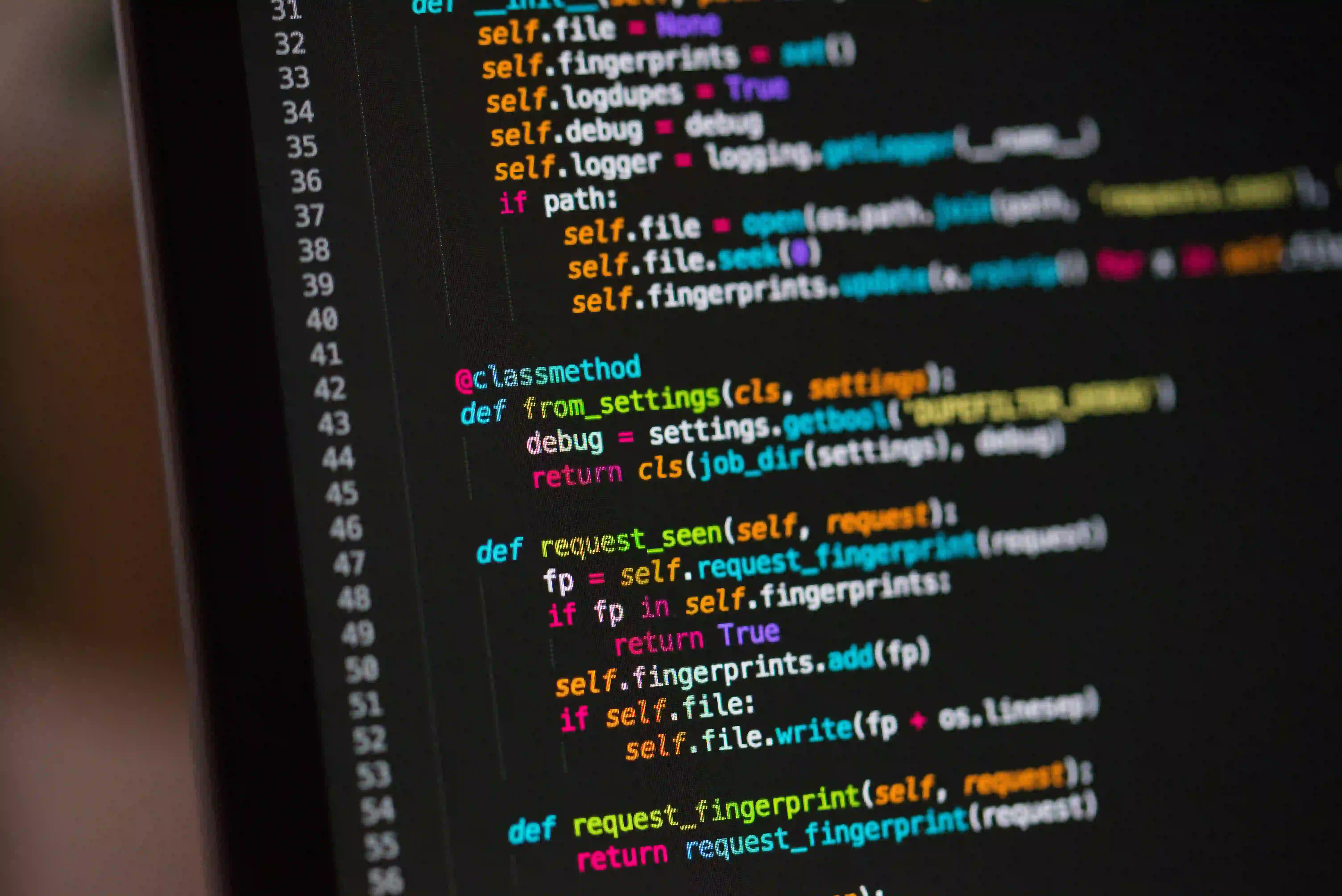
Why Your Throwable in Java Could Cause Runtime Headaches
Java's exception handling framework is a crucial feature of the language, enabling developers to write robust and error-resistant code. Among various exception classes in Java, Throwable
is the superclass of all errors and exceptions. While it provides a fundamental building block for error handling, misusing it can lead to serious runtime headaches. In this blog post, we will delve into the nuances of Throwable
in Java, understand its implications, and explore best practices.
Understanding Throwable
Throwable
is the root class of the exception hierarchy in Java. It has two direct subclasses:
- Error: Represents serious issues that a reasonable application should not try to catch. These are typically related to the Java Virtual Machine (JVM) or system resources.
- Exception: Represents conditions that a user program can catch and recover from.
The decision to catch Throwable
rather than more specific exceptions can lead to undesirable consequences. Let’s explore some of these issues further.
The Catch-All Problem
When you catch Throwable
, you may unknowingly suppress exceptions that should not be handled. For instance, consider a situation where you're trying to handle both exceptions and errors:
try {
// Some operation that might throw an exception
} catch (Throwable t) {
// Handle everything
System.out.println("Caught throwable: " + t.getMessage());
}
Why This is Problematic:
- Suppression of Critical Errors: Catching
Error
can mask serious threats likeOutOfMemoryError
, leading to applications behaving unpredictably or crashing silently. - Misleading Debugging Information: When you catch everything, debugging becomes difficult as you lose track of what went wrong.
Best Practices for Exception Handling
To maintain clarity and robustness in your Java applications, follow these best practices regarding exception handling:
1. Catch Specific Exceptions
Instead of catching Throwable
, aim to catch specific exceptions. This practice helps you manage different error types appropriately.
try {
// Some operation that might throw an exception
} catch (IOException ioException) {
System.out.println("Handled IOException: " + ioException.getMessage());
} catch (SQLException sqlException) {
System.out.println("Handled SQLException: " + sqlException.getMessage());
}
By catching specific exceptions, you can handle them contextually. This approach leads to better error handling and allows you to take appropriate action tailored to the exception type.
2. Use Finally and Try-With-Resources
In Java, resources need to be managed. The finally
block or the Try-With-Resources statement can help manage resources like files or database connections:
Using Finally
FileInputStream fis = null;
try {
fis = new FileInputStream("file.txt");
// Process the file
} catch (FileNotFoundException fnfe) {
System.out.println("File not found: " + fnfe.getMessage());
} finally {
if (fis != null) {
try {
fis.close();
} catch (IOException e) {
System.out.println("Failed to close file: " + e.getMessage());
}
}
}
Using Try-With-Resources
try (FileInputStream fis = new FileInputStream("file.txt")) {
// Process the file
} catch (IOException ioException) {
System.out.println("Handled IOException: " + ioException.getMessage());
}
// No need for a finally block to close input stream
Using the Try-With-Resources statement simplifies resource management and enhances code readability.
3. Log Significant Exceptions
Logging is crucial when you’re dealing with unexpected exceptions. Utilizing logging libraries such as SLF4J or Log4j allows you to configure different log levels. Here's a quick example of logging an exception:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class Example {
private static final Logger logger = LoggerFactory.getLogger(Example.class);
public void performOperation() {
try {
// Some operation that might throw an exception
} catch (Exception e) {
logger.error("An error occurred: ", e);
}
}
}
Why Logging Matters:
- Provides insight into runtime failures.
- Helps trace errors and improves debugging.
- Makes maintaining code easier by allowing you to monitor application behavior in production environments.
4. Create Custom Exceptions
For scenarios where built-in exceptions don't suffice, creating custom exceptions can be beneficial. This adds more contextual information and allows for clearer error handling.
public class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
public class Example {
public void performOperation() throws CustomException {
// Some condition for throwing custom exception
throw new CustomException("This is a custom exception!");
}
}
Creating custom exceptions improves clarity in error handling and allows for more granular control of exception management.
Exception Inheritance
Another common pitfall is misunderstanding how exceptions inherit from one another. Catching a broader exception type might not always catch the specific types of exceptions. Let's see the visibility of exceptions:
try {
// Some operation that might throw an SQLException
} catch (Exception e) {
// Catch-all, including SQLException
} catch (SQLException sqlException) {
// Will never be hit because Exception is caught above
}
The Rule: Always catch the most specific exception first. Be mindful of the order of catch blocks, as Java evaluates them from top to bottom.
Bringing It All Together
While Throwable
serves as a foundational component of Java's exception handling system, over-relying on it can result in significant headaches at runtime. To ensure your application remains robust, utilize specific exception types, employ effective resource management, and leverage logging to diagnose issues.
By adopting these best practices, you not only improve the resilience of your code but also make it easier to maintain and debug in the long run. For further reading, consider exploring the official Java Tutorials on Exceptions for a deeper understanding.
Further Reading
- Effective Java, 3rd Edition by Joshua Bloch
- Java Exceptions - W3Schools
By following these guidelines and insights, you can effectively navigate the complexity of Java's exception handling and elevate the quality of your software development processes.