Beware: How Java 8 Default Methods Can Break Your Code!
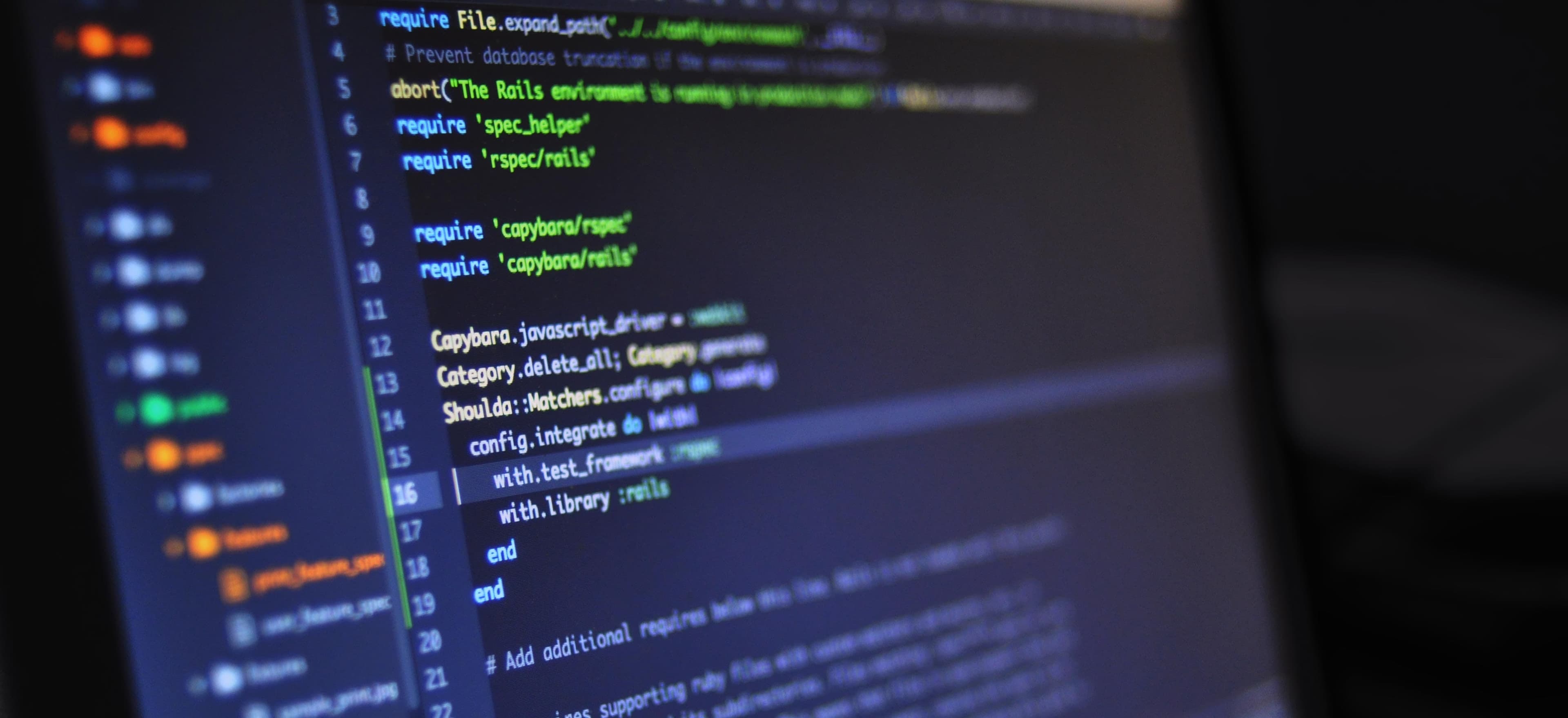
- Published on
Beware: How Java 8 Default Methods Can Break Your Code!
Java 8 introduced many exciting features, one of which is default methods in interfaces. While default methods provide a convenient way to add new functionalities to interfaces without breaking existing implementations, they can also lead to unexpected behaviors and code complexities. In this blog post, we will discuss the ramifications of using default methods in Java, offer insightful code snippets, and share best practices to avoid pitfalls.
Understanding Default Methods
Before we plunge into the potential issues, let’s clarify what default methods are. A default method in an interface is a method that can have an implementation. It provides a way to add a method to an interface while preserving backward compatibility with classes that already implement that interface.
Example of a Default Method
Here's a simple example of a default method:
public interface Vehicle {
void start();
default void honk() {
System.out.println("Honk! Honk!");
}
}
class Car implements Vehicle {
public void start() {
System.out.println("Car is starting.");
}
}
In this example, the Vehicle
interface has a default method honk()
. The Car
class implements Vehicle
and provides its own implementation of the start()
method. However, Car
can still use the default honk()
method without any modifications.
Why Use Default Methods?
- Backward Compatibility: Default methods enable developers to add new methods to interfaces without forcing all implementing classes to provide an implementation immediately.
- Code Reusability: They can reduce code duplication by allowing common behavior to be defined once in the interface.
- Enhanced Interfaces: They lead to more expressive and flexible APIs.
Beware of Method Conflict
One of the most significant issues with default methods arises when there is a method conflict. Consider a scenario where you have multiple interfaces with default methods that have the same name:
interface ElectricVehicle {
default void charge() {
System.out.println("Charging Electric Vehicle");
}
}
interface HybridVehicle {
default void charge() {
System.out.println("Charging Hybrid Vehicle");
}
}
class Tesla implements ElectricVehicle, HybridVehicle {
@Override
public void charge() {
// Choose which default to override or provide a new implementation
ElectricVehicle.super.charge(); // Calls ElectricVehicle's charge()
// HybridVehicle.super.charge(); // Uncommenting this calls HybridVehicle's charge()
}
}
Method Resolution
In the Tesla
class, both ElectricVehicle
and HybridVehicle
provide a charge()
default method. If Tesla
does not override the charge()
method, the compiler will throw an error about ambiguous method call. The resolution requires either:
- Providing a concrete implementation in
Tesla
. - Explicitly calling the desired default method using the
super
keyword.
This conflict emphasizes the necessity of careful consideration when implementing interfaces with same-named default methods across multiple inheritance.
The Diamond Problem
When utilizing multiple interfaces, you might encounter the so-called Diamond Problem. This situation arises when a class inherits from two interfaces that each define a default method with the same signature.
interface A {
default void display() {
System.out.println("Display from A");
}
}
interface B {
default void display() {
System.out.println("Display from B");
}
}
class C implements A, B {
@Override
public void display() {
// Must override to resolve conflict
A.super.display(); // Calls A's display
}
}
Why Is This Important?
The Diamond Problem showcases how default methods can create ambiguity which may lead to maintenance difficulties and potential bugs in your codebase. When overriding methods from multiple interfaces, always ensure clarity on which method is being called to avoid confusion for yourself and other developers in your team.
Considering Future Compatibility
When designing interfaces with default methods, consider the future impact on your codebase. For instance, if you decide to add a new default method to an interface, it could inadvertently affect all classes that implement that interface.
Example of Future Issues
Consider an interface for a user account:
interface UserAccount {
default void deactivate() {
System.out.println("Deactivating User Account");
}
}
class AdminAccount implements UserAccount {
// AdminAccount might have a specific deactivation process
}
If later, you decide that every user should receive a notification email when deactivated:
interface UserAccount {
default void deactivate() {
System.out.println("Deactivating User Account");
// New logic to notify user
}
}
This change will impact every implementing class, potentially breaking assumptions made in their original logic or leading to unwanted behaviors.
Best Practices to Avoid Pitfalls
-
Limit Use of Default Methods: Use default methods sparingly. They are best suited for scenarios where you require backward compatibility and shared functionality.
-
Explicitly Override: In cases of method conflicts, always explicitly override the methods in your implementing class. This ensures you understand what implementation is being executed.
-
Document Interface Changes: Maintain clear documentation for interfaces. When adding default methods, outline the expected behavior and usage.
-
Patterns and Guidelines: Implement design patterns like the Strategy Pattern where specific behavior can be defined in separate classes rather than sprawling across multiple default implementations.
-
Consider Composition: Favoring composition over inheritance can sometimes be a better approach to achieve shared behavior, thereby avoiding the complications of multiple inheritance.
My Closing Thoughts on the Matter
While Java 8’s default methods offer compelling features for interface development, they also introduce complexities that necessitate caution. Understanding the nuances of method resolution, potential conflicts, and forward compatibility will help you take advantage of this feature without endangering your codebase's stability.
To deepen your understanding of Java interfaces, consider diving into the official Java documentation and the more complex aspects of design patterns and the object-oriented design paradigm.
By applying the best practices outlined in this post, you can ensure your use of default methods in Java enhances your code rather than complicates it. Happy coding!
Checkout our other articles