Troubleshooting Spring Boot Undertow Apps on OpenShift
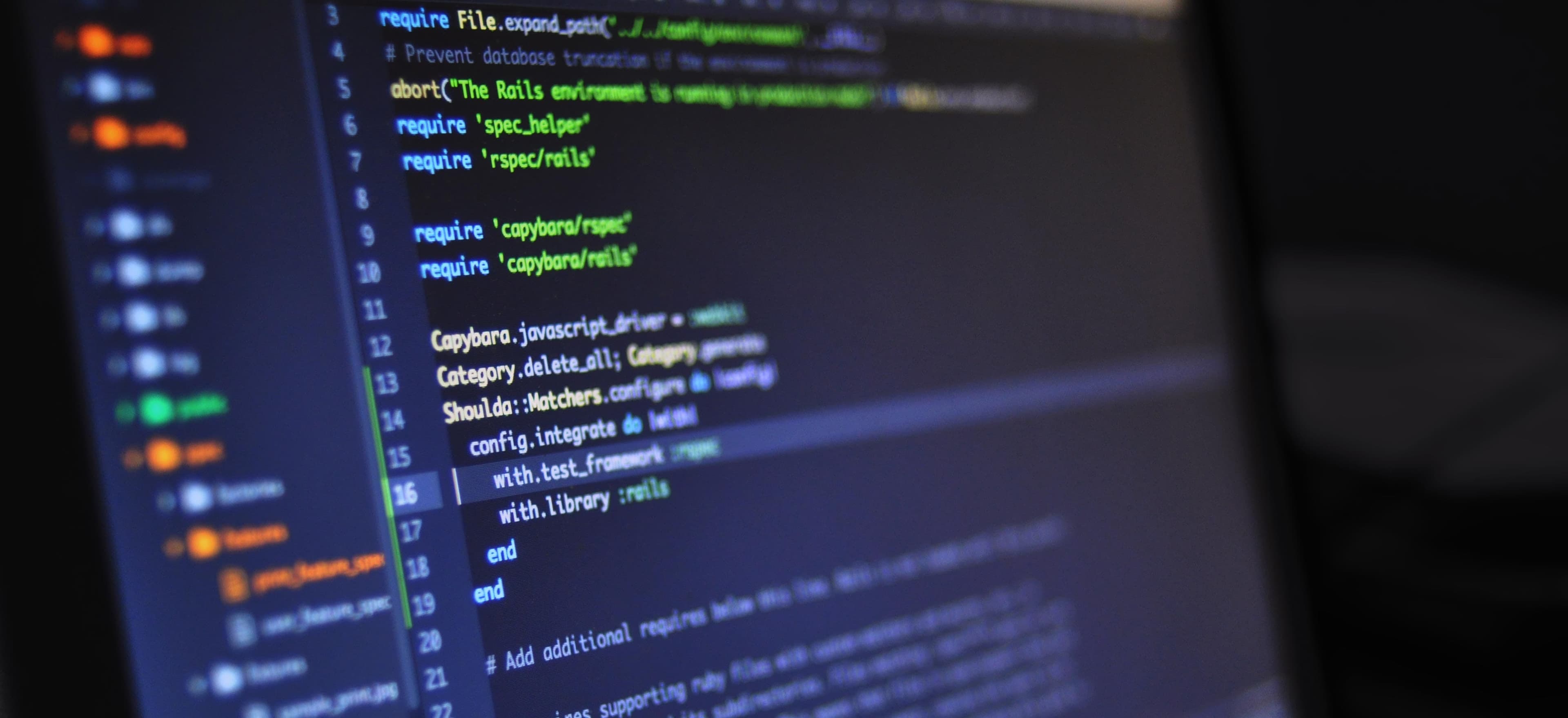
- Published on
Troubleshooting Spring Boot Undertow Apps on OpenShift
Deploying Spring Boot applications using Undertow on OpenShift can sometimes lead to numerous issues. Understanding the best practices for troubleshooting these applications is crucial for maintaining reliability and performance. In this blog post, we will explore key troubleshooting techniques, common issues, and provide examples to help guide you in resolving these challenges.
Table of Contents
- Understanding Spring Boot and Undertow
- Common Issues with Spring Boot Undertow Apps
- Troubleshooting Techniques
- Monitoring Tools
- Conclusion
1. Understanding Spring Boot and Undertow
Spring Boot is a framework that simplifies the development of Java applications, enabling developers to create stand-alone applications with minimal configurations. Undertow is a flexible and performant web server that can handle both blocking and non-blocking APIs. Together, they provide a powerful combination for building high-performance web applications.
Basic Example of a Spring Boot Application with Undertow
The first step is to ensure that your Spring Boot application is properly configured with Undertow. Here’s a simple example:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.embedded.undertow.UndertowServletWebServerFactory;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class MySpringBootApplication {
public static void main(String[] args) {
SpringApplication.run(MySpringBootApplication.class, args);
}
@Bean
public UndertowServletWebServerFactory servletContainer() {
UndertowServletWebServerFactory factory = new UndertowServletWebServerFactory();
factory.setPort(8080);
return factory;
}
}
In this snippet, we define a Spring Boot application that uses Undertow as its embedded web server. The application will listen on port 8080 by default.
2. Common Issues with Spring Boot Undertow Apps
As with any technology stack, certain issues are more prevalent. Being aware of these can speed up your troubleshooting.
Resource Limitations
In OpenShift, containers have defined resource limits. If your application consumes more memory than what is allocated, it can cause the pod to crash.
Startup Failures
Applications might fail to startup if there are misconfigurations in your application.yml
or application.properties
. Make sure your configurations align with the settings of Undertow.
Connectivity Issues
When deploying in a cloud environment like OpenShift, your application may experience connectivity issues with the database or other services due to security protocols and networking configurations.
3. Troubleshooting Techniques
Let’s discuss some troubleshooting techniques that can aid in identifying the problems.
Checking Logs
The first step in troubleshooting is usually checking the application logs. OpenShift provides various ways to do this. You can retrieve logs using the command:
oc logs <pod-name>
This will give you insights into startup errors, unhandled exceptions, or any other runtime issues.
Example:
If you see an exception like java.lang.OutOfMemoryError
, this indicates that your application is consuming more memory than allocated, prompting an adjustment in resource limits in your OpenShift deployment configuration.
Verify Pod Status
Check the status of your pods using:
oc get pods
Each pod will display its current state. If the pod is in a CrashLoopBackOff
state, this indicates that the application keeps crashing on startup.
Health Checks
Inspect your health checks. Spring Boot’s Actuator module can help you expose health metrics. A missing or misconfigured health check can lead to automatic restarts.
You can enable it in your application.properties
like this:
management.endpoint.health.show-details=always
management.endpoints.web.exposure.include=*
This will give you detailed health information, which you can access at:
http://<your-service-url>/actuator/health
Test Locally
Testing your Spring Boot application locally before deploying to OpenShift can also save debug time. Use Maven to run your application:
mvn spring-boot:run
This will help you identify issues that are not specific to the OpenShift environment.
4. Monitoring Tools
Monitoring your application after deployment is crucial. Here are some popular tools available:
OpenShift Monitoring
OpenShift provides built-in monitoring through Prometheus and Grafana. You can configure these tools to collect metrics about your application, which can highlight performance bottlenecks.
Spring Boot Actuator
Utilizing Spring Boot Actuator is an effective way to monitor key application metrics. It exposes endpoints that provide insights into health, metrics, info, and more.
You can add dependencies to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
ELK Stack for Logging
For robust logging, consider using the ELK stack (Elasticsearch, Logstash, and Kibana). This enables centralized logging and powerful search capabilities, making it easier to search through logs across multiple instances.
5. Conclusion
Troubleshooting Spring Boot Undertow applications on OpenShift can be complex, but following a systematic approach will help mitigate common issues.
- Always check your logs and pod statuses first.
- Be aware of the resource limitations imposed by OpenShift.
- Utilize the powerful monitoring tools available.
With the techniques and tools outlined in this post, you should be better prepared to address any challenges you encounter. For further reading, consider checking the Spring Boot Documentation and OpenShift Documentation for more in-depth information.
Remember: Effective troubleshooting not only resolves issues but also leads to better architecture and more robust applications in the long run. Happy coding!
Checkout our other articles