Escape Callback Hell: Mastering Async Code Practices
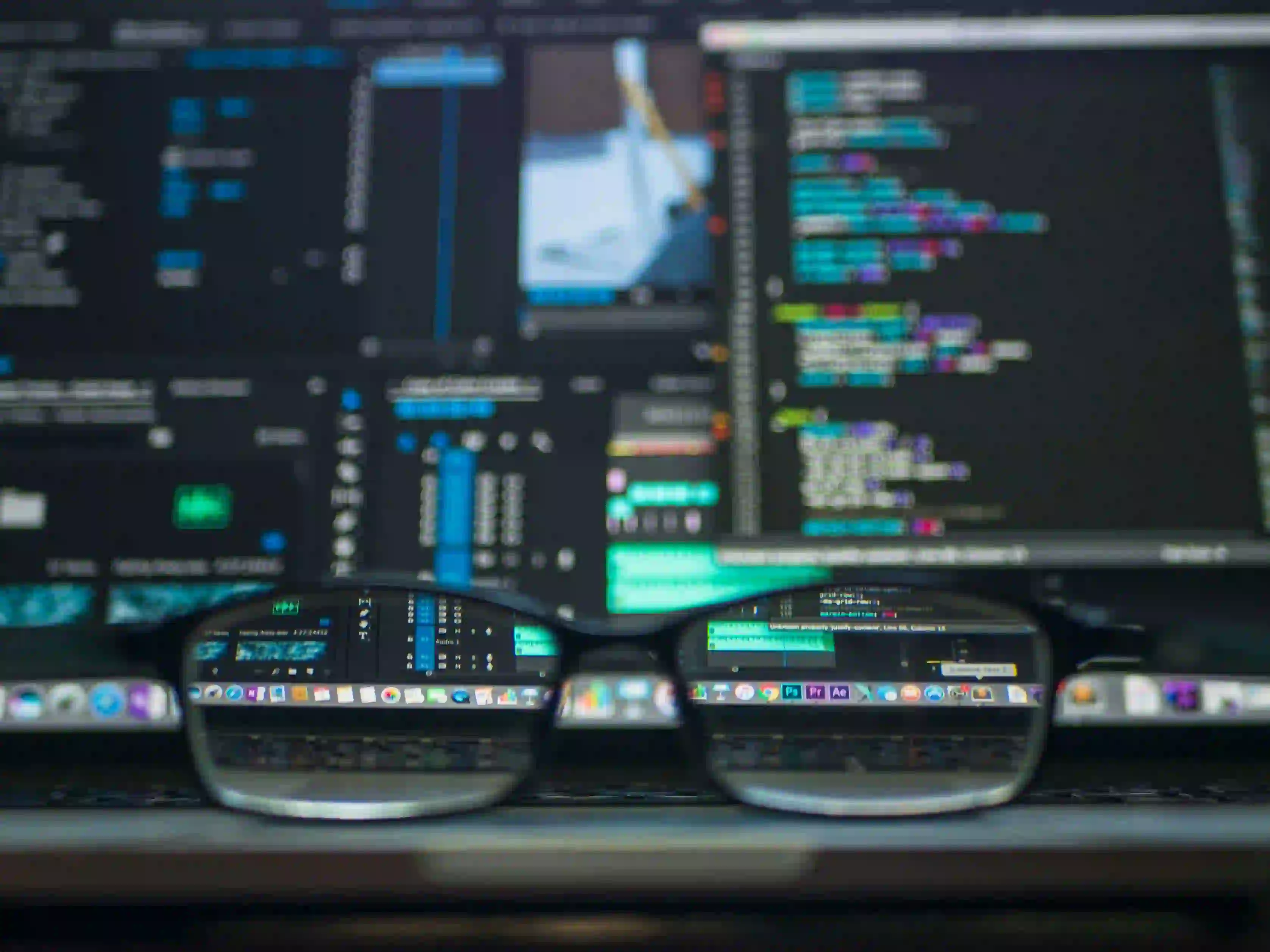
Escape Callback Hell: Mastering Async Code Practices in Java
As a Java developer, you've likely encountered a problem that perplexes many: the infamous Callback Hell. This occurs when you have multiple nested callbacks, leading to difficult-to-read and hard-to-maintain code. Thankfully, Java offers numerous techniques and libraries to help you tame this beast. In this blog post, we will discuss ways to master asynchronous programming in Java, enabling you to write cleaner, more maintainable code.
Why Callbacks Can Lead to Hellish Code
Callback Hell arises when we rely on multiple nested asynchronous calls, leading to an unflattering pyramid structure in our code. This structure not only affects readability but also leads to more complex error handling. Here's an exaggerated scenario that illustrates this problem:
public void fetchDataAsync() {
fetchUserData(userId, (userData) -> {
fetchOrderData(userData.getId(), (orderData) -> {
fetchPaymentData(orderData.getId(), (paymentData) -> {
// and so on...
processData(paymentData); // This is where the readability plummets!
});
});
});
}
In this nested structure, you can see how quickly the layers of callbacks stack up, creating confusion and difficulty in managing your flow of logic.
Alternatives to Callbacks: Promises, CompletableFutures, and Beyond
Java provides several powerful tools for asynchronous programming, the most notable being CompletableFuture
.
What is CompletableFuture?
Introduced in Java 8, CompletableFuture
represents a future result of an asynchronous computation. It allows you to write non-blocking code in a more readable way, helping you avoid the deep nesting associated with callback hell. It helps in providing a clean and fluent API for handling asynchronous programming.
Creating a CompletableFuture
Here's how you can implement CompletableFuture
in Java:
import java.util.concurrent.CompletableFuture;
public CompletableFuture<UserData> fetchUserDataAsync(String userId) {
return CompletableFuture.supplyAsync(() -> {
// Simulate a blocking call to fetch user data
return fetchUserData(userId);
});
}
In this snippet, we define a method that returns a CompletableFuture<UserData>
. The supplyAsync
method allows us to execute a task asynchronously, keeping the main thread free from blocking.
Chaining CompletableFutures
Once we have our CompletableFuture
, we can chain it with other asynchronous tasks, preventing deep nesting.
fetchUserDataAsync(userId)
.thenCompose(userData -> fetchOrderDataAsync(userData.getId()))
.thenCompose(orderData -> fetchPaymentDataAsync(orderData.getId()))
.thenAccept(paymentData -> processData(paymentData))
.exceptionally(ex -> {
handleError(ex);
return null;
});
Why Use thenCompose
?
thenCompose
is particularly powerful because it allows you to handle asynchronous tasks that depend on previous results without the callback nesting you'd typically encounter.
Error Handling
Error handling in asynchronous programming can be tricky. The exceptionally
method provides a straightforward approach to manage exceptions:
.exceptionally(ex -> {
// Log the error and recover or rethrow
System.err.println("Error occurred: " + ex.getMessage());
return null; // Or some default value
});
With exceptionally
, you can gracefully manage errors as they arise, maintaining the flow of your application.
Benefits of Using CompletableFuture
- Improved Readability: Code is easier to read due to its linear flow.
- Error Handling: Built-in mechanisms to handle exceptions in a more manageable way.
- Performance: Non-blocking I/O allows for enhanced performance.
Using Third-Party Libraries
In addition to CompletableFuture, you may also consider other libraries like RxJava or Project Reactor. Both libraries offer robust tools for reactive programming and can further simplify asynchronous code management.
RxJava Example
RxJava is a popular library for composing asynchronous and event-based programs using observable sequences.
import io.reactivex.Observable;
Observable<UserData> userObservable = fetchUserDataObservable(userId);
userObservable
.flatMap(userData -> fetchOrderDataObservable(userData.getId()))
.flatMap(orderData -> fetchPaymentDataObservable(orderData.getId()))
.subscribe(paymentData -> {
processData(paymentData);
}, this::handleError);
Why Reactive Programming?
Reactive Programming allows you to handle data streams in a functional style. With observables, managing state and side effects becomes intuitive while also improving performance.
Additional Resources
For those interested in diving deeper into async programming in Java, I recommend the following resources:
- Java 8 in Action
- Reactive Programming with RxJava
- Modern Java in Action
Key Takeaways
Callback Hell can be a daunting challenge for any Java developer. By leveraging CompletableFuture
and exploring libraries like RxJava, you can structure your asynchronous code in a way that is clear, concise, and easier to maintain.
Remember, code should be written for others to read, not just for the computer to execute. So, ditch the callbacks and embrace the power of asynchronous programming through modern techniques provided by Java's evolving ecosystem.
With these strategies at your disposal, you'll not only escape Callback Hell but also elevate your coding practices to a new standard of excellence. Happy coding!