The Hidden Dangers of Not Testing Your Data Access Code
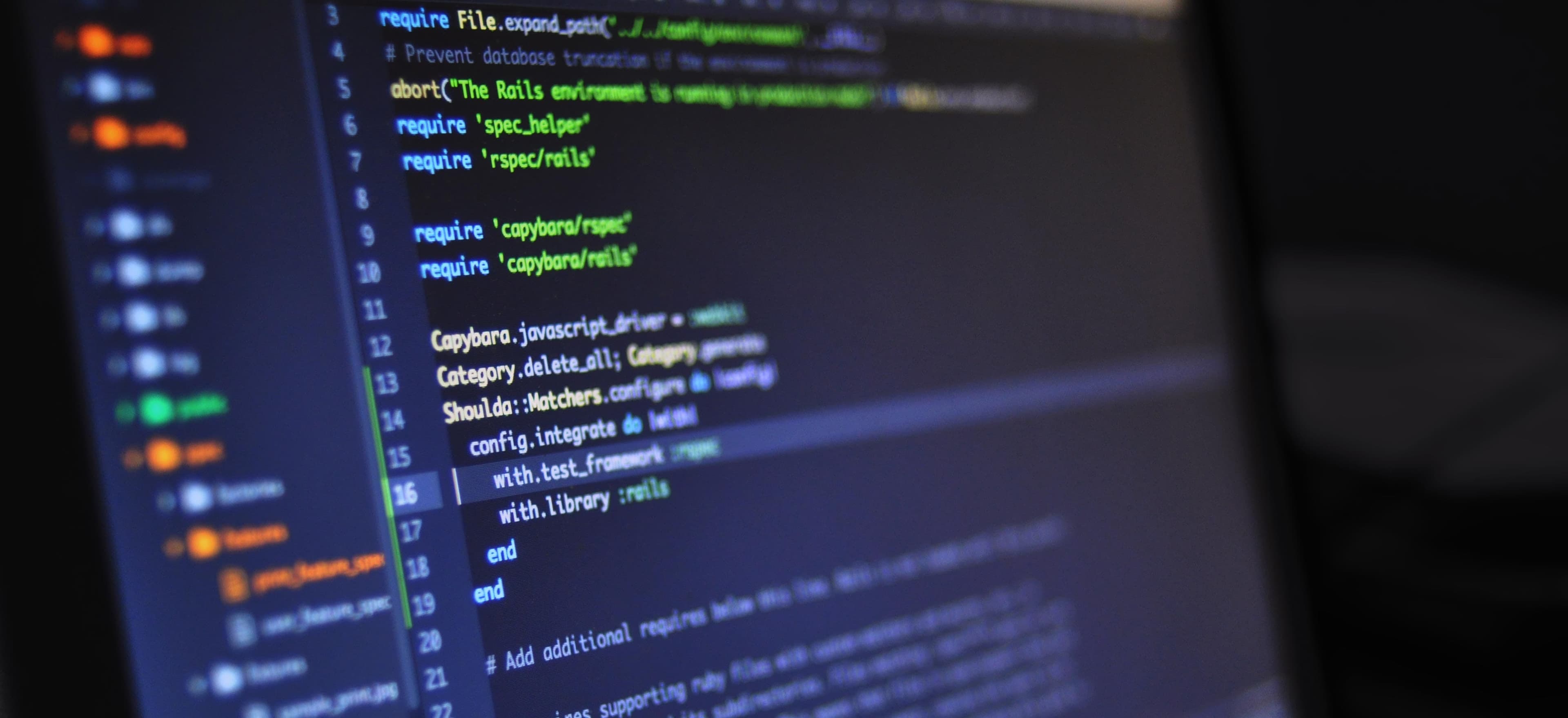
- Published on
The Hidden Dangers of Not Testing Your Data Access Code
In today’s software landscape, where data drives decisions and informs user experiences, robust data access code is vital. However, it’s common for developers to underestimate the importance of testing this code comprehensively. This oversight can lead to various problems, including data corruption, security vulnerabilities, and performance issues. In this blog post, we will explore the hidden dangers of skipping tests for your data access code and present best practices for ensuring reliability and integrity in your systems.
Understanding Data Access Code
Before diving deep, let’s define what data access code is. In Java applications, this typically refers to the sections of code that handle interactions with a database or data source, often performed through Java Database Connectivity (JDBC) or using Object-Relational Mapping (ORM) frameworks like Hibernate.
Consider the following simple JDBC code snippet for fetching user details from a database:
public User getUserById(int userId) throws SQLException {
String sql = "SELECT * FROM users WHERE id = ?";
User user = null;
try (Connection conn = DriverManager.getConnection(DB_URL, USER, PASS);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, userId);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
user = new User();
user.setId(rs.getInt("id"));
user.setName(rs.getString("name"));
// populate other user fields
}
}
return user;
}
Why Testing is Crucial
-
Ensure Correctness
The primary purpose of testing any code is to ensure that it behaves as expected. For data access code, incorrect SQL queries or improper handling of results can lead to missing data or corrupt data. Imagine a situation where a user’s record isn’t retrieved correctly, causing confusion for both the user and support teams.
-
Catch SQL Exceptions Early
Failing to validate input to your data access layer can lead to
SQLExceptions
. These can stem from typographical errors, wrong inputs, or even database changes that go unnoticed during development. By introducing comprehensive tests, you can intercept potential SQL issues before they reach production.Example of handling exceptions:
try { User user = getUserById(userId); if (user == null) { throw new UserNotFoundException("User not found for ID: " + userId); } } catch (SQLException e) { // Log the exception and handle it System.err.println("SQL exception occurred: " + e.getMessage()); }
-
Prevent Security Vulnerabilities
Data access code is susceptible to SQL injection attacks if input is not properly sanitized. Testing your data access code can help you recognize and mitigate potential vulnerabilities. Utilizing prepared statements, like shown above, is a good practice in this regard.
-
Maintainability and Refactoring
As your application evolves, the data model may change. Without tests, refactoring data access code becomes risky. Broken features may go unnoticed until they are reported by users. Comprehensive unit tests ensure that changes in the codebase do not inadvertently break existing functionality.
Types of Tests for Data Access Code
To effectively test your data access code, you should consider the following types of tests:
1. Unit Tests
Unit tests focus on individual components of your code, testing them in isolation to ensure they behave correctly. These are typically written using frameworks like JUnit.
Example of a Unit Test:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
import java.sql.SQLException;
public class UserDaoTest {
@Test
public void testGetUserById() throws SQLException {
UserDao dao = new UserDao();
User user = dao.getUserById(1);
assertNotNull(user);
assertEquals("John Doe", user.getName());
}
}
2. Integration Tests
Integration tests cover interactions between units, such as the connection between your data access layer and the actual database. Tools like Testcontainers can be instrumental for spinning up a lightweight database for tests.
Example using Testcontainers:
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.testcontainers.containers.PostgreSQLContainer;
public class UserDaoIntegrationTest {
static PostgreSQLContainer<?> postgresContainer = new PostgreSQLContainer<>("postgres:latest");
@BeforeEach
void setUp() {
postgresContainer.start();
// Initialize data for testing
}
@Test
public void testDatabaseIntegration() {
UserDao dao = new UserDao();
User user = dao.getUserById(1);
assertNotNull(user);
}
}
Best Practices for Testing Data Access Code
-
Use Version Control
Always version control your testing code. This enables you to trace back tests alongside the changes in your data access code.
-
Keep Tests Independent
Tests should be independent of each other. This means that running one test should not affect or require the state of another.
-
Mock External Services
Use mocking frameworks like Mockito to simulate interactions with external dependencies, reducing flakiness in tests.
-
Test for Performance
Performance tests ensure that your data access code can handle the expected load. Long-running queries can slow down your application, so it’s essential to test query performance, especially under heavy load.
Closing Remarks
Neglecting to test your data access code carries hidden dangers that can severely impact your application's reliability, security, and performance. Implementing various testing practices—unit tests, integration tests, and performance tests—is vital in maintaining quality.
By incorporating testing into your development lifecycle, you create a safety net that not only helps catch errors early but also fosters maintainability and scalability. In the long run, the investment you make in testing pays off as you build a more resilient and trustworthy application.
For further reading about best practices in unit testing with Java, consider checking out Java Testing with JUnit 5 or dive deeper into performance testing with JMeter. Happy coding!
Checkout our other articles