Secure Your Data: JPA Type Converter Encryption Dilemma
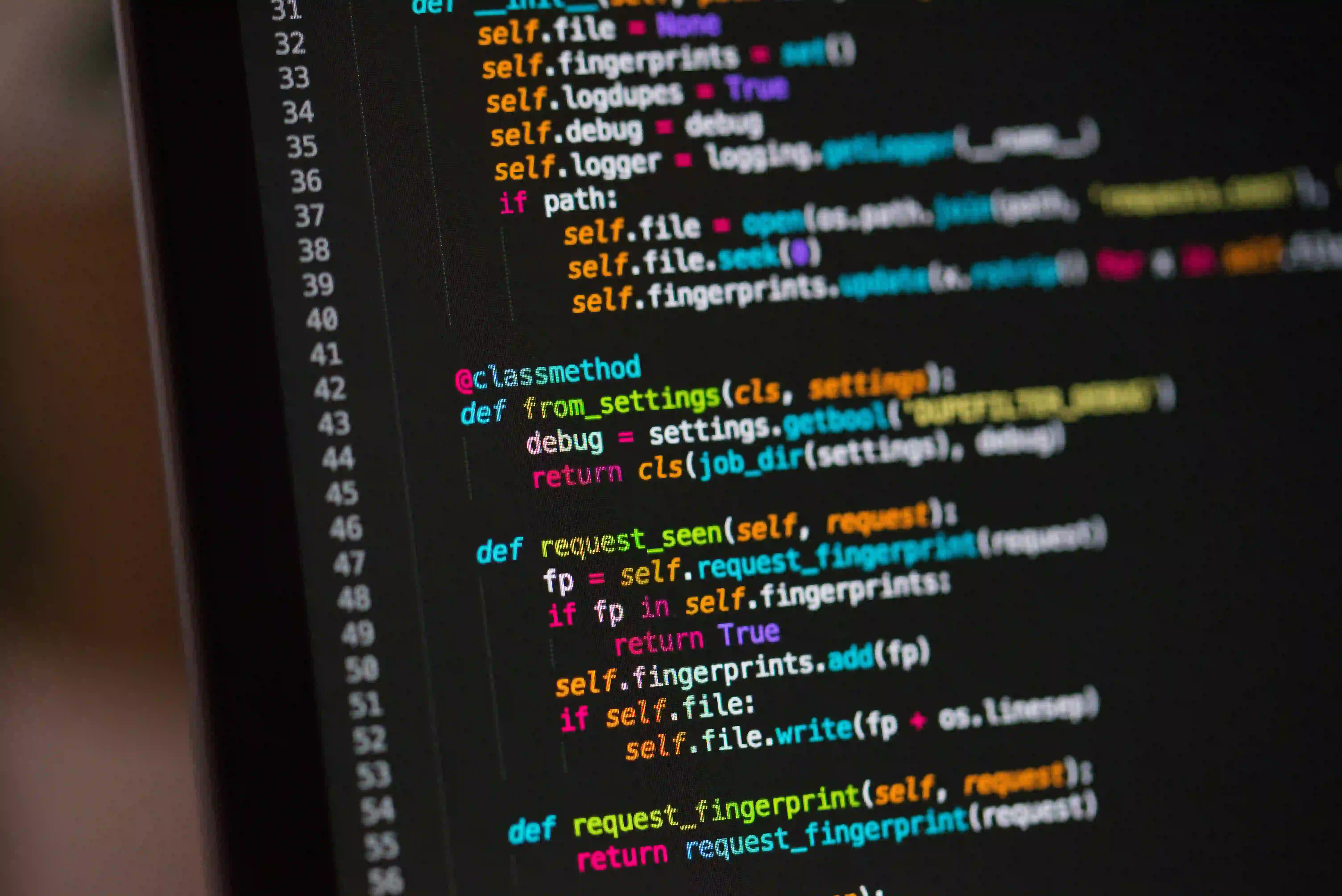
Secure Your Data: JPA Type Converter Encryption Dilemma
In the realm of modern application development, securing sensitive data is a fundamental requirement. With increasing threats and vulnerabilities, it's essential to utilize best practices that ensure data protection, especially when using databases. In this context, Java Persistence API (JPA) Type Converters emerge as a powerful feature that can help encrypt sensitive data before it's stored in databases. However, the implementation of encryption and decryption mechanisms using JPA Type Converters often leads to challenges and dilemmas.
In this blog post, we'll explore the use of JPA Type Converters for data encryption, addressing common pitfalls and providing practical code snippets to help you enhance data security.
Understanding JPA Type Converters
Before diving into data encryption, it's crucial to understand what JPA Type Converters are. A JPA Type Converter is a mechanism that allows developers to define custom behavior for the transformation of entity attributes. They provide a way to convert attributes into a different database type or format without changing the database schema.
Creating a Simple Type Converter
Here's a simple example of a JPA Type Converter that converts a String
attribute to a String
database column.
import javax.persistence.AttributeConverter;
import javax.persistence.Converter;
@Converter(autoApply = true) // Auto apply this converter to all String fields
public class StringToUpperCaseConverter implements AttributeConverter<String, String> {
@Override
public String convertToDatabaseColumn(String attribute) {
if (attribute == null) {
return null; // Handle null values
}
return attribute.toUpperCase(); // Convert to uppercase before saving
}
@Override
public String convertToEntityAttribute(String dbData) {
return dbData; // No transformation when reading back
}
}
This converter takes a String
, converts it to uppercase before storing it in the database, and reverts it back upon retrieval. Notice the importance of handling null values, which is essential in any conversion logic.
The Need for Data Encryption
While type converters offer many benefits, simply converting data is often not enough, especially when dealing with sensitive information like passwords, credit card numbers, or personal identification numbers. This is where encryption comes into play.
Encryption transforms plaintext data into ciphertext, rendering it unreadable to unauthorized users. However, implementing encryption effectively requires careful consideration of various factors, such as encryption algorithms, key management, and performance overhead.
JPA Type Converter for Encryption
Let's extend our earlier Type Converter example to implement data encryption. We'll use the Java Cryptography Extension (JCE) for this purpose. For simplicity, we will demonstrate symmetric encryption with AES (Advanced Encryption Standard).
Steps for Implementing Encryption
-
Define the Encryption Utility Class: This class will perform the encryption and decryption operations.
-
Create the JPA Type Converter: The converter will utilize the encryption utility for data transformations.
-
Integrate with an Entity: Finally, we'll showcase how to use the Type Converter with a JPA entity.
Encryption Utility Class
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class EncryptionUtil {
private static SecretKey secretKey;
static {
try {
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(128); // 128-bit AES
secretKey = keyGen.generateKey();
} catch (Exception e) {
throw new RuntimeException("Error initializing key generator", e);
}
}
public static String encrypt(String data) {
try {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encrypted = cipher.doFinal(data.getBytes());
return Base64.getEncoder().encodeToString(encrypted); // Encode to Base64 for storage
} catch (Exception e) {
throw new RuntimeException("Error encrypting data", e);
}
}
public static String decrypt(String encryptedData) {
try {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decoded = Base64.getDecoder().decode(encryptedData);
byte[] decrypted = cipher.doFinal(decoded);
return new String(decrypted);
} catch (Exception e) {
throw new RuntimeException("Error decrypting data", e);
}
}
// Provide a method to get the secret key securely if needed
public static SecretKey getSecretKey() {
return secretKey;
}
}
In this utility class, we create an AES key and define methods for encrypting and decrypting data. The encrypted output is Base64-encoded to ensure compatibility with storage types in databases.
JPA Type Converter for Encryption
import javax.persistence.AttributeConverter;
import javax.persistence.Converter;
@Converter(autoApply = true) // Apply to fields that need encryption
public class EncryptedStringConverter implements AttributeConverter<String, String> {
@Override
public String convertToDatabaseColumn(String attribute) {
if (attribute == null) {
return null;
}
return EncryptionUtil.encrypt(attribute); // Encrypt before saving
}
@Override
public String convertToEntityAttribute(String dbData) {
if (dbData == null) {
return null;
}
return EncryptionUtil.decrypt(dbData); // Decrypt when reading back
}
}
Integrating with an Entity
With our converter in place, we can now utilize it within a JPA entity.
import javax.persistence.*;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username")
private String username;
@Convert(converter = EncryptedStringConverter.class)
@Column(name = "password") // Store encrypted passwords
private String password;
// Getters and setters
}
With this setup, the user's password is automatically encrypted when saved and decrypted when retrieved, providing an additional layer of security.
Performance Dilemma
While using a JPA Type Converter for encryption has its advantages, it also introduces performance considerations. Cryptographic operations can be computationally expensive, leading to slower database transactions, especially for large datasets. Here are some aspects to keep in mind:
-
Database Lookups: For highly sensitive data, you may want to limit the number of times you read/write to the database. Consider caching decrypted values in memory when appropriate.
-
Batch Processing: If you're handling bulk data, think about processing records in batches to mitigate the performance hit.
-
Connection Management: Ensure efficient connection management to avoid bottlenecks when performing encryption and decryption.
Key Management
One of the most critical challenges in implementing encryption is key management. Hardcoding your encryption keys in your application is highly discouraged, as it can lead to vulnerabilities. Instead, consider these strategies:
- Environment-Specific Configuration: Store your keys in environment variables or secure vault services, such as AWS Secrets Manager or HashiCorp Vault.
- Key Rotation: Regularly rotate your encryption keys to limit the exposure of data in case of a breach. Implement a key rotation mechanism that allows old keys to decrypt data until all dependencies are migrated to new keys.
The Closing Argument
Utilizing JPA Type Converters for data encryption presents an effective means to safeguard sensitive information in your Java applications. While the implementation may introduce complexities, the benefits far outweigh the costs when aligned with best practices.
Implementing encryption not only aids in regulatory compliance but also builds trust with users and clients who rely on your application to safeguard their data. As threats evolve, taking a proactive stance on data security will set your applications apart.
For further reading, check out Java Cryptography Documentation and JPA Documentation for more insights into security practices and database management.
By employing encryption correctly, you can navigate the complexities of data security with confidence.