Common Mistakes When Implementing reCAPTCHA in Spring MVC
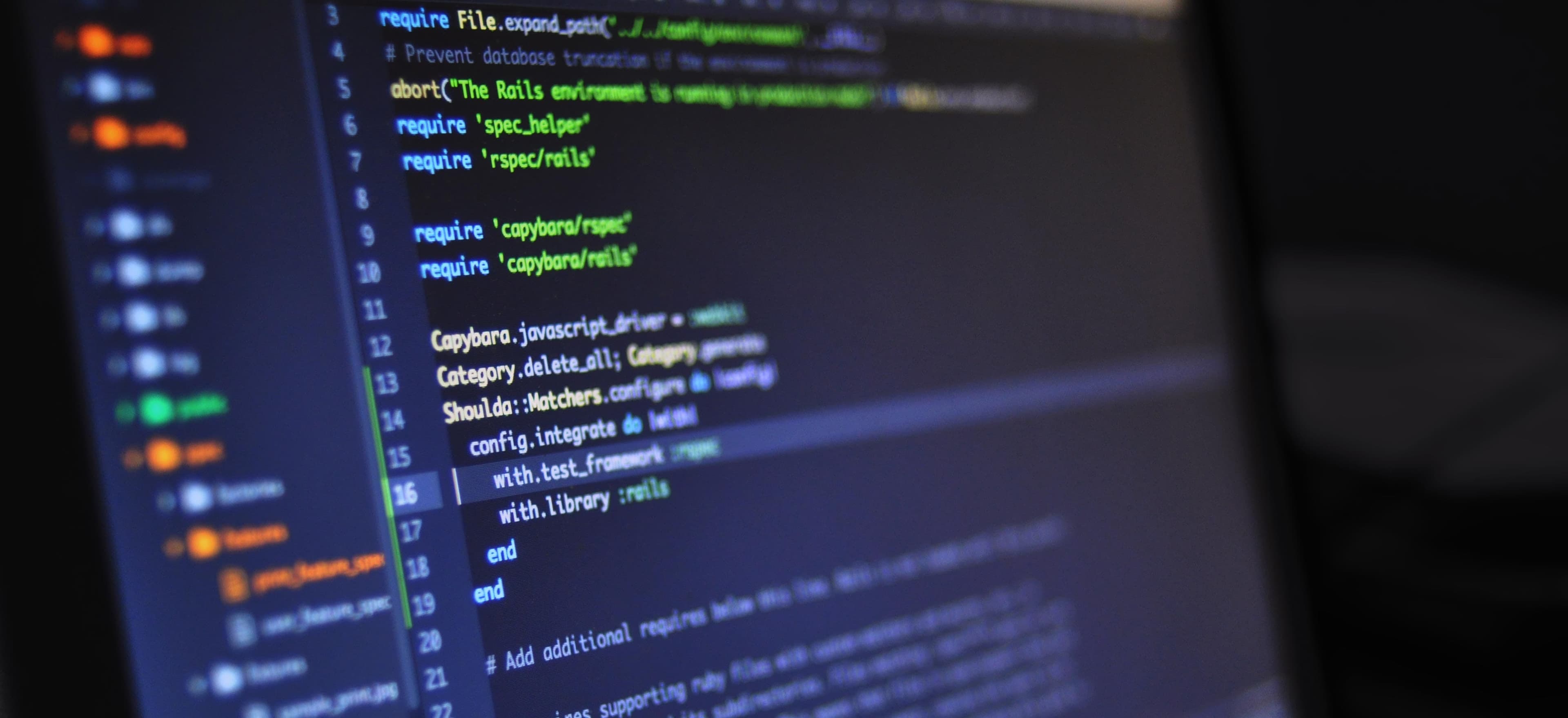
- Published on
Common Mistakes When Implementing reCAPTCHA in Spring MVC
In an era when web applications are ubiquitous, protecting them against malicious bots is paramount. One of the most popular methods for achieving this is Google’s reCAPTCHA. Integrating reCAPTCHA into a Spring MVC application, however, can present several pitfalls. This blog post aims to provide developers with an insightful guide on common mistakes made during this implementation and offers solutions to each.
What is reCAPTCHA?
reCAPTCHA is a free service that helps protect websites from spam and abuse. It provides an additional layer of security by requiring users to complete challenges that are easy for humans but difficult for bots. You can find more about it on the Google reCAPTCHA website.
Prerequisites
Before diving into common mistakes, ensure that you have a basic understanding of Spring MVC and how to set up a Spring application. You will also need API keys for reCAPTCHA, which can be acquired from the Google reCAPTCHA admin console.
1. Forgetting to Include Required Dependencies
One of the initial hurdles to overcome is ensuring that your project includes all necessary dependencies. Failing to do this will result in the application not functioning as expected.
Solution
For a Maven project, you need to include the following dependency in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
This dependency is essential for developing web applications in Spring.
2. Incorrectly Renewing Tokens
Another common error involves the mismanagement of reCAPTCHA tokens. During the communication between your frontend and backend, ensuring that the token generated by reCAPTCHA is correctly validated is crucial.
Solution
You should send the token received from the reCAPTCHA widget to your Spring controller and verify it using a request to Google's reCAPTCHA API. Below is an example of how to do this:
@PostMapping("/submit")
public String submitForm(@RequestParam("g-recaptcha-response") String recaptchaResponse, Model model) {
String secretKey = "YOUR_SECRET_KEY"; // Replace with your secret key
String url = "https://www.google.com/recaptcha/api/siteverify";
RestTemplate restTemplate = new RestTemplate();
MultiValueMap<String, String> params = new LinkedMultiValueMap<>();
params.add("secret", secretKey);
params.add("response", recaptchaResponse);
ResponseEntity<String> response = restTemplate.postForEntity(url, params, String.class);
if (isValidCaptcha(response.getBody())) {
model.addAttribute("message", "Form submitted successfully!");
} else {
model.addAttribute("error", "Invalid reCAPTCHA. Please try again.");
}
return "form-view";
}
Commentary
In the above snippet, we utilize Spring’s RestTemplate
to send a POST request to Google's API. The isValidCaptcha
method processes the response to determine success or failure. This is crucial for security.
3. Failing to Handle reCAPTCHA Errors Properly
Another mistake developers often make is overlooking error handling related to reCAPTCHA. Users expecting smooth interactions may find the application confusing without clear feedback.
Solution
Implement error handling that clearly communicates issues to the user. An example of handling errors in a form submission could look like this:
private boolean isValidCaptcha(String responseBody) {
JsonObject jsonObject = new JsonParser().parse(responseBody).getAsJsonObject();
boolean success = jsonObject.get("success").getAsBoolean();
if (!success) {
// Log specific error codes for further inspection or debugging
String errorCodes = jsonObject.get("error-codes").toString();
System.err.println("ReCAPTCHA Error: " + errorCodes);
}
return success;
}
Commentary
In the isValidCaptcha
method, we check the success status. If it fails, we log the error codes. This is not only informative for debugging but also helps with improving the user experience.
4. Not Utilizing Async Functionality
reCAPTCHA can potentially slow down your application if the token verification is performed synchronously. This can hinder user experience.
Solution
Using asynchronous calls for verifying reCAPTCHA can improve performance. One way to achieve this is by using Spring's @Async
annotation. Here’s how:
@Service
public class CaptchaService {
@Async
public CompletableFuture<Boolean> verifyCaptcha(String recaptchaResponse) {
// logic to verify reCAPTCHA
return CompletableFuture.completedFuture(isValidCaptcha(response));
}
}
Commentary
By using CompletableFuture
, we can execute the verification in a non-blocking manner. This enhances user experience, making the application feel more responsive.
5. Overlooking Frontend Integration
Integration mistakes aren’t limited to the backend. Make sure the reCAPTCHA widget is correctly set up on the frontend. The HTML should contain the following script:
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
And the widget might be included like this:
<div class="g-recaptcha" data-sitekey="YOUR_SITE_KEY"></div>
Commentary
Including the correct JavaScript source is essential for rendering the reCAPTCHA widget correctly. Ensure that your site key is valid and corresponds to your registered domain.
6. Neglecting to Include Security Checks on All Endpoints
Believing that reCAPTCHA automatically secures your application is another common misconception. Always enforce additional validation checks on your form submission endpoints.
Solution
Consider validating user inputs beyond just reCAPTCHA validation. Always sanitize and validate incoming data to protect against attacks such as SQL Injection or Cross-Site Scripting (XSS).
@InitBinder
protected void initBinder(WebDataBinder binder) {
binder.registerCustomEditor(String.class, "username", new StringTrimmerEditor(true));
}
Commentary
The approach above ensures that even if reCAPTCHA validation passes, the input sanitization will mitigate risks from malicious input.
My Closing Thoughts on the Matter
Integrating reCAPTCHA in Spring MVC can enhance your web application's security, but it comes with its own set of challenges. By avoiding common pitfalls such as forgetting dependencies, mishandling token verification, neglecting error handling, and overlooking frontend integration, you can create a more robust application.
Always remember to stay updated with the official Google reCAPTCHA documentation to get the latest best practices and examples.
Implementing a secure and user-friendly application is not just a technical requirement but a step toward providing your users with a trustworthy environment. Happy coding!