Maximizing Efficiency: Batching Requests in Hystrix
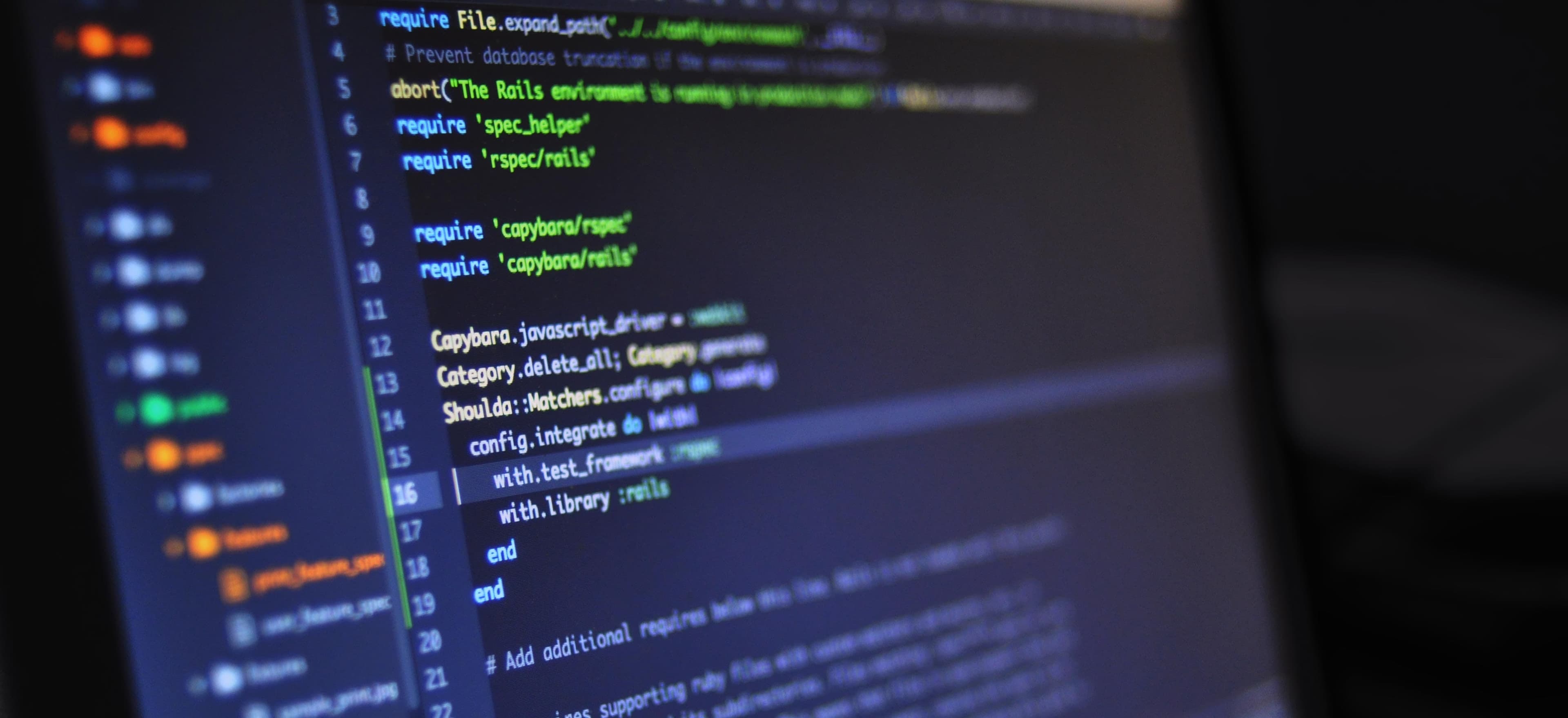
- Published on
Maximizing Efficiency: Batching Requests in Hystrix
In the realm of microservices architecture, efficiency and resilience are paramount. One widely regarded approach to enhance service performance and fault tolerance is Netflix's Hystrix library. A standout feature of Hystrix is its ability to batch requests, minimizing latency and optimizing resource utilization. This blog post will delve into the concepts behind batching requests in Hystrix, discussing its advantages, implementation strategies, and providing practical code snippets along the way.
What is Hystrix?
Hystrix is a library designed to control the interactions between distributed services and prevent cascading failures. It provides a way to define fallback methods, circuit breakers, and request caching, all of which contribute to service reliability.
The essence of Hystrix is its ability to isolate dependencies. This isolation allows a service to function effectively, even when downstream dependencies become unreliable. You can read more about the capabilities of Hystrix on the official Netflix Hystrix documentation.
The Need for Request Batching
When microservices interact with one another, they inevitably generate requests that can lead to latency issues, especially under high load. Request batching can significantly reduce the number of calls made to a service by grouping them into a single call. This not only minimizes the overhead of HTTP (or any transport protocol) requests but also optimizes throughput and resource usage.
Advantages of Batching Requests
- Reduced Latency: Batching combines multiple requests, negating the need for separate latency from multiple network calls.
- Improved Throughput: By efficiently using connection resources, you can increase the volume of requests processed.
- Lower Resource Consumption: Fewer connections mean fewer resources used by both client and server.
- Enhanced Reliability: Fewer requests lead to reduced chances of failure due to network issues.
Implementing Request Batching in Hystrix
Dependencies
Before we jump into implementation, ensure you have the necessary dependencies in your pom.xml
if you're using Maven:
<dependency>
<groupId>com.netflix.hystrix</groupId>
<artifactId>hystrix-core</artifactId>
<version>1.5.18</version>
</dependency>
<dependency>
<groupId>com.netflix.hystrix</groupId>
<artifactId>hystrix-metrics-event-stream</artifactId>
<version>1.5.18</version>
</dependency>
Basic Setup
To enable request batching in Hystrix, we use the HystrixObservableCommand
class. This abstract class allows you to define a command that can be executed and handles batching inherently. The following code snippet provides a simplified example of how this can be defined.
import com.netflix.hystrix.HystrixObservableCommand;
import rx.Observable;
import java.util.List;
import java.util.ArrayList;
public class BatchRequestCommand extends HystrixObservableCommand<List<String>> {
private final List<String> requestIds;
protected BatchRequestCommand(List<String> requestIds) {
super(Setter.withGroupKey(HystrixCommandGroupKey.Factory.asKey("BatchGroup")));
this.requestIds = requestIds;
}
@Override
protected Observable<List<String>> construct() {
// Simulating a batch fetch request
return Observable.fromCallable(() -> {
List<String> results = new ArrayList<>();
for (String id : requestIds) {
// Fetch the data for each requestId
results.add(executeNetworkCall(id));
}
return results;
});
}
private String executeNetworkCall(String requestId) {
// Simulating a network call
// In a real application, you could use RestTemplate or Feign to fetch data
return "Response for ID: " + requestId;
}
}
Explanation
In this code snippet:
- We define a
BatchRequestCommand
that extendsHystrixObservableCommand
. - The constructor takes a list of request IDs.
- The
construct()
method uses anObservable
to execute the network calls in a batched manner.
Batching occurs at the method level, meaning each individual request is not sent until we call the execute
method on this command. This can lead to significant performance improvements in scenarios where multiple requests are common.
Executing the Command
To execute our batch command, you can do so like this:
import rx.Observable;
import java.util.Arrays;
public class BatchRequestExample {
public static void main(String[] args) {
List<String> ids = Arrays.asList("1", "2", "3", "4", "5");
BatchRequestCommand command = new BatchRequestCommand(ids);
Observable<List<String>> observable = command.observe();
observable.subscribe(
result -> System.out.println("Received: " + result),
error -> System.err.println("Error: " + error)
);
}
}
Benefits of Observable
Using Observable
allows us to handle asynchronous and event-driven programming paradigms seamlessly. It maximizes throughput while minimizing resource wastage. Each time an instance of BatchRequestCommand
is called, you are effectively orchestrating a batch request and generating an observable that can be subscribed to for results.
Consistency and Fallbacks
While batching helps with efficiency, it’s also crucial to implement fallback strategies. A well-designed application should handle scenarios where the batching request fails. For instance, you can specify a fallback method using Hystrix's capabilities:
@Override
protected Observable<List<String>> resumeWithFallback() {
// Return a default value or an error response
return Observable.just(Arrays.asList("Fallback response for failed requests"));
}
Summary
By implementing batching requests in Hystrix, developers can effectively improve the responsiveness and resource efficiency of their microservices. By grouping requests and using observables, not only can you reduce the overhead involved in network communication, but you can also add resilience into your application design.
It's essential to remember that while batching reduces the number of requests, it shouldn't introduce excessive complexity or lead to bottlenecks in your service. Regularly monitor the performance of your Hystrix commands and tweak the batching strategy as necessary to ensure optimal results.
For further reading and exploration:
- Explore how Hystrix works with Spring Boot for a more integrated approach.
- Refer to the Hystrix Dashboard to visualize metrics and performance.
Happy coding!